An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
How to mint 10000 NFTs on Opensea
Minting to Opensea
After creating all your NFTs the more hardest step is to mint so many NFTs to Opensea mostly in large quantities without a contract.
To do it I'll share my repository that uses puppeteer to automate the process of minting the NFTs, you just need an images folder file and the images name to mint all. I'll teach you how to use the repository below.
If you want to add another property on the mint time send me an email we can discuss it
[email protected]
.
Install the packages
To start we go to install the packages to run the script, on your project run:
yarn add @chainsafe/dappeteer puppeteer esbuild esbuild-register
The @chainsafe/dappeteer
serves to automate the metamask connect to mint the NFTs.
The puppeteer
we will use to upload the images and fill the inputs.
And we are going to use the esbuild
and esbuild-register
to run our script.
Create the script
Let's start creating a file called script.ts
file doing the first imports and adding your first variables.
script.ts
import puppeteer, { Page } from 'puppeteer';import dappeteer from '@chainsafe/dappeteer';import fs from 'fs';const collectionName = "Your Collection Name"const collectionURL = `https://opensea.io/collection/${collectionName}/assets/create`const openseaDescription = `Your description here`const lockedContent = `Locked content text here`
Connect Wallet Function
So to select the Metamask wallet when our script opens the Browser, let's create a function to click on the Metamask button and connect to the wallet.
script.ts
const connectWallet = async (page: Page, metamask) => { const button = await page.$('button.dBFmez:first-child'); await button.click(); await metamask.approve(); return;}
Create the Upload Image function
Now to upload the image on create NFT page let's create the function which receives the page and the file then takes the input element in the HTML and uploads the image to it.
script.ts
const uploadImage = async (page: Page, file: string) => { const elementHandle = await page.$("#media"); await elementHandle.uploadFile(`images/${file}`); return;}
Create the page timeout function
This function is to give time for our script to perform the filling or clicks in the application.
script.ts
const pageTimeout = async (time: number, page: Page) => { await page.waitForTimeout(time) return;}
Create the fill fields function
this is the function where we are going to get each field from the create NFT page and fill it.
the steps this function takes
1- Fill in the field name
2 - Fill in the field input.
3 - Turn on the unlockable content and fill the unlockable content text.
4 - Select the Polygon chain
script.ts
const fillFields = async (page: Page, fileName: string) => {// Get and fill in the input name await page.focus('#name') await page.keyboard.type(fileName) await pageTimeout(1000, page)//Get and fill in the input name await page.$eval('#description', (el, value) => el.value = value, openseaDescription); await page.focus('#description') await page.keyboard.type(' ') await pageTimeout(1000, page)// Click on the unlockable content checkbox await page.evaluate(() => { document.querySelector("#unlockable-content-toggle").parentElement.click(); }); await pageTimeout(1000, page)// Fill in the unlockable content text await page.$eval('textarea[placeholder="Enter content (access key, code to redeem, link to a file, etc.)"]', (el, value) => el.value = value, lockedContent); await page.focus('textarea[placeholder="Enter content (access key, code to redeem, link to a file, etc.)"]') await page.keyboard.type(' ')// Open the select chain input const input = await page.$("#chain") input.click() await pageTimeout(1000, page)// Select the polygon chain await page.click('img[src="/static/images/logos/polygon.svg"]') return;}
Creating the Main Function
In this function we go to create all the main functions to run our scripts the steps are:
1 - Run the dappeteer to set up the Metamask on Opensea.
2 - Get our files from the images folder file.
3 - Remove the first file(.DS_Store) - just for macOS.
4 - Open the create asset page of the collection.
5 - Run the connect wallet function
6 - Run a loop for each image on the images folder (to create the asset one by one)
See the code below step-by-step:
script.ts
(async () => { // Async function because we need promises to do it const browser = await dappeteer.launch(puppeteer, { metamaskVersion: 'v10.1.1' }); // Launch the browser with metamask const metamask = await dappeteer.setupMetamask(browser, { seed: "Secret phase here"}); // Add your secret phase here to metamask connect with your account const files = await fs.promises.readdir("images/"); // Get an List with all images on images folder files.shift() // WARN: just on macOS: remove the first file .DS_Store // Open the create assets url of the collection const page = await browser.newPage(); await page.goto(collectionURL);// Get the tabs and close the first tab openned by the dappeteer const firstTabs = await browser.pages() await firstTabs[0].close() await pageTimeout(2000, page)// Run our function to click on the Metamask button in the Opensea await connectWallet(page, metamask)// Start the loop on each image of images folder for (let i = 0; i <= files.length ; i++) { const tabs = await browser.pages() // Get the tabs on each loop const data = { name: `Your Asset name here #${1 + i}`, // Add your NFT name (the count start on 1 and stop on the quantity of the files) }// At the first time on loop you need to do an sign to create the assets if(i === 0) { await tabs[1].bringToFront() // Move one tab await tabs[1].goto(collectionURL) // Change the page to collection url again await pageTimeout(2000, page) await metamask.sign() // Use the metamask to do the transaction await metamask.page.waitForTimeout(2000) // wait for the transaction }// Now if not the first time, after creating the first NFT just open the create assets page again to create the second NFT and so sequentially. if(i === 0) { await tabs[1].bringToFront() await tabs[1].goto(collectionURL) } else { await tabs[1].bringToFront() await tabs[1].goto(collectionURL) } await pageTimeout(2000, page)// Upload the current image file await uploadImage(page, files[i]);// Fill the fields using the asset name with the count await fillFields(page, data.name);// Click on create asset button const button = await page.$('.AssetForm--action button'); await button.click() await pageTimeout(4000, page)// Rename the image name to know if already is completed fs.renameSync(`images/${files[i]}`, `images/completed-${files[i]}`) console.log({ message: `Mint the NFT: ${data.name}`, fileName: files[i]}) console.log(`Mint the NFT: ${data.name}`) } console.log('Minted all NFTs with success')})();
Ready!! now Let's config our package.json
to run the script with one line
package.json
{ "name": "node", "version": "1.0.0", "main": "index.js", "license": "MIT", "dependencies": { "@chainsafe/dappeteer": "^2.2.0", "dappeteer": "^1.0.0", "esbuild": "^0.13.10", "esbuild-register": "^3.0.0", "puppeteer": "^10.4.0" }, "scripts": { "es": "node -r esbuild-register" }}
Now to run your script just run on the CLI:
yarn es ./src/script.ts
Download the Repository
First, you need to download my repository here that contains the script to mint the NFTs.
Star my repository here
It's done, now the puppeteer will create all your NFTs one by one and so much fast.
That's it!!
Original Link: https://dev.to/emanuelferreira/how-to-mint-10000-nfts-on-opensea-487c

Dev To
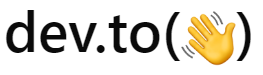
More About this Source Visit Dev To