An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
JSON: All You Need to Know
JSON stands for JavaScript Object Notation. It is a data-interchange format used to communicate between the server and the client. JSON is not a language but a syntax.
The name JavaScript Object Notation came from its deliberate similary to JavaScript objects. However, JSON is made keeping in mind data types like objects, dictionaries, arrays, lists from C-family of programming languages.
The main features of JSON include,
- Being lightweight
- Is text-based
- And language-independent
Why JSON?
JSON has two structures,
- Collection of key:value pairs
- An ordered list
These are structures heavily inspired by JavaScript objects and arrays. In fact you could take JSON objects and JSON arrays, paste it in a JavaScript file and get valid JavaScript. At the same time, similar data types exist in multitude of backend languages like Python, PHP, and Nodejs. This makes JSON very easy to parse. Remember, JSON is a string in the end and need to be parsed to an appropriate data type for programming languages to process.
This is in contrast to XML, the predecessor of JSON. XML is also used to communicate between server and client. But JSON can be parsed directly in to a JavaScript object or array which is ready for use. XML is much harder to parse as it is syntactically different.
Example of XML:
<presidents> <president> <firstName>George</firstName> <lastName>Bush</lastName> </president> <president> <firstName>Barack</firstName> <lastName>Obama</lastName> </president> <president> <firstName>Donald</firstName> <lastName>Trump</lastName> </president></presidents>
Example of JSON:
{"presidents":[ { "firstName":"George", "lastName":"Bush" }, { "firstName":"Barack", "lastName":"Obama" }, { "firstName":"Donald", "lastName":"Trump" }]}
From the above examples, you can take home three points:
- JSON is significantly smaller and easier to read & write
- A JavaScript developer don't need to be worried about learning extra syntax
- JSON can hold array of data (unlike XML)
How to write JSON?
We already saw a glimpse of how JSON looks like. If you want to be a serious developer, you must know how to write JSON. We write JSON in .json
file and its values must be one of the following data types:
- string
- number
- object
- array
- boolean
- null
Remember, all these are accepted data types for values in JSON. JSON itself is fundamentally written in two structures discussed earlier. But, you can still write any of these data types in a .json
file and it is perfectly valid JSON that can be parsed.
JSON written in key:value pairs,
{"name": "John","age": 24,"contact": { "email":"[email protected]", "phone": "987654321",},"hobbies": ["reading", "gardening", "gaming"],"isLoggedIn": true,"employment": null,}
JSON written as an array of data,
["john", 24, {"contact" : { "email":"[email protected]", "phone":"987654321",}}, ["reading", "gardening", "gaming"], true, null ]
Writing JSON shouldn't be hard if you know JavaScript. But it will take sometime before you get used to the right way of nesting data for your particular use case.
Parsing JSON
JSON is a string of data and needs to be parsed before using. In the frontend, JavaScript has an object called JSON
that contains methods for parsing and converting to JSON data.
To parse a JSON data, we use JSON.parse()
.
const obj = JSON.parse(json)
The return value of JSON.parse()
depends on the JSON string. It can be a string, number, object, array, boolean, or null. Mostly, you will be dealing with an object or an array.
Here is an example of how JSON.parse
works.
JSON.parse("{}") // {}JSON.parse("true") // trueJSON.parse('"string"') // "string"JSON.parse('[2, 0, "false"]') // [2, 0, "false"]JSON.parse("null") // null
JSON.parse
accepts a second value called reviver
. The reviver
is a function that can be used to transform the parsed value of JSON before being returned.
const number = JSON.parse(5, (key, value) => value * 2)console.log(number) // 10
Notice how I passed two parameters to the reviver
function. This is because the data is parsed into key:value pairs.
For the backend, parsing JSON totally depends on the language of your choice. In python, there is a module called json
which you can import. In nodejs, you get access to the same JSON
object.
Converting to JSON
To communicate, we also need to convert data back to JSON string. The same JSON
object can used in the frontend again. Since JavaScript and JSON has pretty much the same syntax, all what is remaining is to convert it to a string. For that, we use JSON.stringify()
method.
The JSON.stringify()
method converts a JavaScript object or value to a JSON string.
JSON.stringify({}) // "{}"JSON.stringify(true) // "true"JSON.stringify("string") // '"string"'JSON.stringify([2, 0, "false"]) // '[2, 0, "false"]'JSON.stringify(null) // null "null"
Just like JSON.parse()
method, JSON.stringify()
also accepts a second parameter called replacer
. It can be used to alter the behavior of stringification process.
const json = JSON.stringify(2, (key, value) => value * 2)console.log(json) // 4
Here too, I have passed two parameters.
The stringify
method even accepts a third parameter meant for spacing.
const spaced = JSON.stringify({ a: 1, b: 2 }, null, "")consol.log(spaced)/* { "a": 1, "b": 2}*/
The is the tab character. The string has added a tab space infront of each key:value pair. This is just for improving readability.
Again, for the backend, converting to JSON totally depends on the language of your choice. Python has a module called json
. In nodejs, you get access to same JSON
object.
Practicing JSON
To conclude, I would like to leave you with two resources that can help you practice JSON.
- JSON Placeholder has fake api endpoints with JSON data
- json server can run a local json server
That's about it. Thanks for reading.
Original Link: https://dev.to/aravsanj/json-all-you-need-to-know-3fl6

Dev To
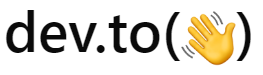
More About this Source Visit Dev To