An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Loading animation with React.js styled-components
So here we are with another post and today I'm going to show you all a way to make a loading animation for your application with styled-components. So let's jump right to it!
Creating a React app
1- First of all we are going to create a project with react and install styled-components.
# Comand to create a react appyarn create react-app my-app# Go to the folder you created## And then install styled-componentsyarn add styled-components
2- Second of all we create a loading component and make 2 styled elements, a LoadingWrapper and a Dot.
(I like to create a folder for the component and create 2 files a index file with the logic and a style file. That's the way I'm going to be doing)
import { Dot, LoadingWrapper } from './styles'export default function Loading() { return ( <LoadingWrapper> <h3>Loading</h3> <Dot /> <Dot /> <Dot /> </LoadingWrapper> )}
3- Okay now we got our Loading component, so let's go to the styles we are going to be using and import then into our file.
import styled from 'styled-components'export const LoadingWrapper = styled.div` display: flex; align-items: flex-end; justify-content: center;`export const Dot = styled.div` background-color: black; border-radius: 50%; width: 0.75rem; height: 0.75rem; margin: 0 0.25rem;`
4- So what we have until now, just the word "Loading" with 3 dots on the side. Now we are going to be making the animation itself. (You can make the animation on the same file of the style for the Loading component or create a file separated)
export const BounceAnimation = keyframes` 0% { margin-bottom: 0; } 50% { margin-bottom: 1rem; } 100% { margin-bottom: 0; }`
5- With the animation in hands we need to add it on the Loading styles and we can make something that's pretty cool with styled-components and that is passing props to the component. So let's go to it.
// If you made the animation in a different folder// you need to import itimport { BounceAnimation } from ''export const Dot = styled.div` background-color: black; border-radius: 50%; width: 0.75rem; height: 0.75rem; margin: 0 0.25rem; /*Animation*/ animation: ${BounceAnimation} 0.5s linear infinite; animation-delay: ${(props) => props.delay};`
6- And last but not least add the delay prop to the Dot component in the Loading folder.
import { Dot, LoadingWrapper } from './styles'export default function Loading() { return ( <LoadingWrapper> <h3>Loading</h3> <Dot delay="0s" /> <Dot delay="0.1s" /> <Dot delay="0.2s" /> </LoadingWrapper> )}
And TADA you have a animated Loading component just like this:
mine is styled a little bit different but the animation is just like that
Now I'm leaving...
And you have a animated Loading component to use through out your applications and go crazy with it, you can use this with Typescript too if y'all want I can make another post about it, See you next time, peace!
Original Link: https://dev.to/gabrlcj/loading-animation-with-react-js-styled-components-3m0n

Dev To
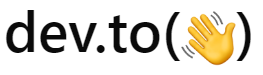
More About this Source Visit Dev To