An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
C Array Methods
In C# there is an Array class which provides methods related to array operations. In this blog we'll look into some of the common methods used with arrays.
Basics
Declaring an array
// Array of integersint[] myArray = new int[5] { 1, 2, 3, 4, 5 };// Array of stringsstring[] people = new string[3] {"John", "Smith", "Bill"};
Getting the length of an array
To get the length of an array we can use the Length
property of the Array class.
int[] nums = {1,2,3,4,5,6};Console.Write(nums.Length);
Array.Find()
This method is used to search an element in an array. It will return the first element found.
int[] arr = new int[] {1,2,3,4,5};Console.Write(Array.Find(arr, (elem) => elem % 2 == 0)); // 2
Array.FindAll()
This methods is used to find all the elements in the array that matches the given condition.
int[] arr = new int[] {1,2,3,4,5};var result = Array.FindAll(arr, (elem) => elem % 2 == 0);
The result from above code snippet will contain the array of elements returned from the function that matches the condition. We can use the foreach loop to print the elements in the result array.
foreach(var elem in result){ Console.WriteLine(elem);}// 2// 4
Array.Exist()
This method is used to check whether an element exists that match the specified condition in the function.
string[] users = {"Steve", "Bob", "Shane", "Andrew"};Console.WriteLine(Array.Exists(users, user=> user.StartsWith("S"))); // True
Array.IndexOf()
This method is used to find the first occurrence of an element in an array.
string[] users = { "Smith", "John", "Clark", "Peter", "John" };Console.WriteLine(Array.IndexOf(users, "John")); // 1
Array.Sort()
This method is used to sort the given array. This method returns void
, that means it manipulates the same array and didn't return any result.
string[] users = {"Zack", "Bob", "Shane", "Andrew"};Array.Sort(users);// users = {"Andrew", "Bob", "Shane", "Zack"}
This is basic method I have shown here, you can view all the overloads for this method here.
Array.Reverse()
This method reverse the order of an array. For example,
string[] users = {"Zack", "Bob", "Shane", "Andrew"};Array.Reverse(users);// users = {"Andrew", "Shane", "Bob", "Zack"}
Array.BinarySearch()
Using this method we can perform a binary search on the array. The array must be sorted before passing it to this method. It returns the index of the element found in the array. If the array is not sorted before using this method you may see some unexpected results.
int[] nums = {1,6,12,18,32};Console.WriteLine(Array.BinarySearch(nums, 12)); // 2
Original Link: https://dev.to/sheikh_ishaan/c-array-methods-54dj

Dev To
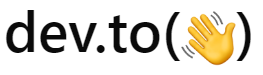
More About this Source Visit Dev To