An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
The Beginner's Guide to Declaring Variables in JavaScript
If you're new to programming and new to javascript, it can be confusing when to use the three declaration key words. We're going to talk about when to use const
, let
, and why you should never use var
to declare variables. Hopefully by the end of this post, you'll be an expert.
Brief History
Before ECMAScript 2015 - ES6 there was only var
, but people found problems with var because you could easily overwrite variables without knowning it. So const
and let
were introduced. But first let's look at the particular problems of var
.
Problems with Var
Let's say you are writing some code about books, and so you assign a variable called book
to "Harry Potter", but later in you code you forget you already used book
as a variable so you assign book
to "Lord of the Rings". And then a third time you assign book
to "The Hobbit". So now you've just overwritten the variable 2 times.
var book = "Harry Potter"//Now book = "Lord of the Rings"var book = "Lord of the Rings"//And now book = "The Hobbit"book = "The Hobbit"book--> "The Hobbit"
Since var
is unreliable, we should never use it. If you need to declare a variable use const
.
When in Doubt, Use const
const
is the most strict with declaratation, a variable can only be assigned once, and it can't ever be changed.
const x = 0const x = 5---> SyntaxError: Identifier 'x' has already been declaredx = 5---> TypeError: Assignment to constant variable.
const
is your friend, it'll save you countless hours searching for a bug because that can be prevented by just using const.
When to just let
it be
When we use let
we are declaring a variable that we plan on changing later. The best example is if we need to use a variable as a counter.
let x = 5---------> 5x + 1--------> 6x = 2---------> 2let x = 2---------> SyntaxError: Identifier 'x' has already been declared
In Conclusion
-When in doubt of which one to use, use const
.
-Use let when you know the variable's contents are going to be added to or changed in some form.
-Never use var.
Original Link: https://dev.to/leepzig/the-beginner-s-guide-to-declaring-variables-in-javascript-5ckf

Dev To
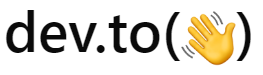
More About this Source Visit Dev To