An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
May 22, 2021 02:53 pm GMT
Original Link: https://dev.to/alexadam/create-a-react-project-from-scratch-with-typescript-and-webpack-2l18
Create a React project from scratch, with TypeScript and Webpack
A step by step guide on how to create a React project from scratch, with TypeScript and Webpack 5.
Setup
Prerequisites:
- node
- yarn
Create the project's folder:
mkdir react-appcd react-app
Generate a default package.json file with yarn:
yarn init -y
Install React, TypeScript and Webpack:
yarn add react react-domyarn add --dev @types/react \ @types/react-dom \ awesome-typescript-loader \ css-loader \ html-webpack-plugin \ node-sass \ sass-loader \ style-loader \ typescript \ webpack \ webpack-cli \ webpack-dev-server
Add build, dev & clean scripts in the package.json file:
.... }, "scripts": { "clean": "rm -rf dist/*", "build": "webpack", "dev": "webpack serve" }
Configure TypeScript by creating the file tsconfig.json with:
{ "compilerOptions": { "incremental": true, "target": "es5", "module": "commonjs", "lib": ["dom", "dom.iterable", "es6"], "allowJs": true, "jsx": "react", "sourceMap": true, "outDir": "./dist/", "rootDir": ".", "removeComments": true, "strict": true, "moduleResolution": "node", "allowSyntheticDefaultImports": true, "esModuleInterop": true, "experimentalDecorators": true }, "include": [ "client" ], "exclude": [ "node_modules", "build", "dist" ]}
To configure Webpack, make a file webpack.config.js containing:
const path = require("path");const app_dir = __dirname + '/client';const HtmlWebpackPlugin = require('html-webpack-plugin');const HTMLWebpackPluginConfig = new HtmlWebpackPlugin({ template: app_dir + '/index.html', filename: 'index.html', inject: 'body'});const config = { mode: 'development', entry: app_dir + '/app.tsx', output: { path: __dirname + '/dist', filename: 'app.js', publicPath: '/' }, module: { rules: [{ test: /\.s?css$/, use: [ 'style-loader', 'css-loader', 'sass-loader' ] }, { test: /\.tsx?$/, loader: "awesome-typescript-loader", exclude: /(node_modules|bower_components)/ }, { test: /\.(woff|woff2|ttf|eot)(\?v=[0-9]\.[0-9]\.[0-9])?$/, exclude: [/node_modules/], loader: "file-loader" }, { test: /\.(jpe?g|png|gif|svg)$/i, exclude: [/node_modules/], loader: "file-loader" }, { test: /\.(pdf)$/i, exclude: [/node_modules/], loader: "file-loader", options: { name: '[name].[ext]', }, }, ] }, plugins: [HTMLWebpackPluginConfig], resolve: { extensions: [".ts", ".tsx", ".js", ".jsx"] }, optimization: { removeAvailableModules: false, removeEmptyChunks: false, splitChunks: false, }, devServer: { port: 8080, // open: true, hot: true, inline: true, historyApiFallback: true, },};module.exports = config;
Example App
Create a folder named client (in the project's folder):
mkdir clientcd client
Make a simple React component, in the file numbers.tsx:
import React, {useState} from 'react';interface INumberProps { initValue: number}const Numbers = (props: INumberProps) => { const [value, setValue] = useState(props.initValue) const onIncrement = () => { setValue(value + 1) } const onDecrement = () => { setValue(value - 1) } return ( <div> Number is {value} <div> <button onClick={onIncrement}>+</button> <button onClick={onDecrement}>-</button> </div> </div> )}export default Numbers
Create the main React component (the entry point), in the file app.tsx:
import * as React from 'react';import * as ReactDOM from 'react-dom';import Numbers from './numbers';ReactDOM.render( <Numbers initValue={42} />, document.getElementById('app') as HTMLElement );
Next, add the index.html:
React TypeScript<p><br></p><pre class="highlight plaintext"><code>Then, run `yarn dev` and open `http://localhost:8080/` in a browser.## Use this project as a templateYou can save the *Setup* steps as a shell script:```sh#!/bin/shrm -rf node_modulesrm package.jsonyarn init --yesyarn add react react-domyarn add --dev @types/react \ @types/react-dom \ awesome-typescript-loader \ css-loader \ html-webpack-plugin \ node-sass \ sass-loader \ style-loader \ typescript \ webpack \ webpack-cli \ webpack-dev-server# Remove the last linesed -i.bak '$ d' package.json && rm package.json.bak# append the scripts commadscat <<EOT >> package.json ,"scripts": { "clean": "rm -rf dist/*", "build": "webpack", "dev": "webpack serve" }}</code></pre><p></p><p>Delete the <strong>node-modules</strong> folder and, when you want to start a new project, you can copy the contents of <em>react-app</em> to the new location:<br></p><pre class="highlight shell"><code><span class="nb">mkdir </span>new-project<span class="nb">cd </span>new-project<span class="c"># copy the react-app folder content to the new project</span>rsync <span class="nt">-rtv</span> /path/to/../react-app/ <span class="nb">.</span>./init.sh</code></pre><p></p>
Original Link: https://dev.to/alexadam/create-a-react-project-from-scratch-with-typescript-and-webpack-2l18
Share this article:
Tweet
View Full Article

Dev To
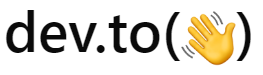
More About this Source Visit Dev To