Setup nested routes in react
Setup nested routes in react
reactrouterdom, reactjs, nestedrouting, react
all the files used are here https://codesandbox.io/s/setup-nested-routes-in-react-j80to
Using routes in a single file in a big react project is a huge mess and a lot of work
So the solutions comes as nested routing
in simpler terms routes in different files one inside another
In the above file structure , the routes in the Auth.js gets imported in the Routes.js file
Route.js
import React from 'react';import { BrowserRouter as Router, Switch } from 'react-router-dom';import Auth from './auth/Auth';const Routes = () => { return ( <Routes> <Switch> <Route path={`/auth`} component={Auth} /> <Route path={`/`}> <h1>Home</h1> </Route> </Switch> </Routes> );};export default Routes;
The Route.js component will contain basic router code but the magic will happen in Auth.js
We start with creating a switch will hold multiple routes
Auth.js
import React from 'react';import { Switch } from 'react-router-dom';const Auth = () => { return <Switch></Switch>;};export default Auth;
then add the required routes to it
import React from 'react';import { Switch, Route } from 'react-router-dom';const Auth = () => { return ( <Switch> <Route> <h1>Login Page</h1> </Route> <Route> <h1>Register Page</h1> </Route> </Switch> );};export default Auth;
then we will add a useRouteMatch
function from react-router-dom
the useRouteMatch
function will give us a path and a url what we will be needing is the path
var
import React from 'react';import { Switch, useRouteMatch, Route } from 'react-router-dom';const Auth = () => { const { path } = useRouteMatch(); return ( <Switch> <Route> <h1>Login Page</h1> </Route> <Route> <h1>Register Page</h1> </Route> </Switch> );};export default Auth;
then we will add path to the routes , the path will be useRoutesMatch path + /whatever_the_sub_path_is
import React from 'react';import { Switch, useRouteMatch } from 'react-router-dom';const Auth = () => { const { path } = useRouteMatch(); return ( <Switch> <Route path={`${path}/login`}> <h1>Login Page</h1> </Route> <Route path={`${path}/register`}> <h1>Register Page</h1> </Route> </Switch> );};export default Auth;
Whats useRouteMatch?
useRouteMatch is provided by react-router-dom
which gives use a object
if you log the value of useRouteMatch
you will get something like this
{ "path: "/auth", "url": "/auth", "isExact": false }
the path variable gives us the path we mentioned in the Routes.js file here
<Route path={`/auth`} component={Auth} /> ^
then the route we mentioned in the Auth.js file
<Route path={`${path}/login`}> <h1>Login Page</h1></Route>
if we log ${path}/login
it will give us /auth/login
also make sure to not put a /
in the parent route it will make the sub route = /auth//login
which cause problems
this simple nesting can be used in a bigger project without causing mess in a single file
Thanks for reading , hearts if you liked it , follow if you loved it
Original Link: https://dev.to/akshatsinghania/setup-nested-routes-in-react-bf5

Dev To
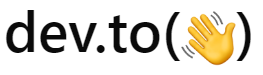
More About this Source Visit Dev To