C Basic Web Applications
HTTP CRUD Methods
CRUDstands for**CreateReadUpdateDelete.
GETrequests information from the server, which is usually displayed to users. ASP.NET includes aHttpGet()
method for GET requests.
POSTalters information on the server. MVC has anHttpPost()
method for POST requests.
PATCHupdatesexistinginformation on the server.
DELETEremoves data from the server.
We must use POST requests to update and delete records because HTML5 forms don't recognize PATCH AND DELETE.
Adding Delete to a Form Example
...<form action="/items/delete" method="post"> <button type="submit" name="button">Clear All Items</button></form>
Adding Delete to a Controller Example
[HttpPost("/items/delete")]public ActionResult DeleteAll(){ // Code goes here.}
Finding Objects with Unique IDs
Readonly property: a property that can be read but not overwritten.
Introduction to RESTful Routing
Terminology
- REST: Short forRepresentational State Transfer.
- RESTful Routing: A set of standards used in many different languages to create efficient, reusable routes.
REST Conventions
- Route Namerefers to the name of the route method in the controller.
- URL Pathrefers to the path listed above the route in a route decorator. This will also be the URL a user sees when navigating to this area of the site.
- HTTP Methodrefers to the HTTP method that route will respond to, or be invoked for.
- Purposedetails what each route is responsible for.
:id
is a placeholder for where a specific object's unique ID will be placed.
Applying RESTful Routing
Dynamic Routing
Dynamic Routingrefers to routes and their URL paths that can dynamicallychange. Here's an example of a dynamic route:
[HttpGet("/items/{id}")]public ActionResult Show(int id){ Item foundItem = Item.Find(id); return View(foundItem);}
- The
{id}
portion of the path is a placeholder. - The corresponding link in the view looks like this:
<ahref='/items/@item.Id'>
.
Objects Within Objects Interface Part 2
RESTful routing conventions for applications that use objects within objects look like the image below.
Following RESTful routing doesn't require we use all routes. It just requires that the routes wedoneed in our applications follow these conventions.
Using Static Content
In order to add CSS or images to our application, we need to update theStartup.cs
toUseStaticFiles()
:
Startup.cs
public void Configure(IApplicationBuilder app) { ... app.UseStaticFiles(); //THIS IS NEW ... app.Run(async (context) => { ... }); }
img
andcss
directories need to be inside ofwwwroot
, which should be in the project's root directory.
wwwroot img css styles.css
Now we can link to an image like this:
<img src='~/img/photo1.jpg'/>
Layouts and Partials
Layout view: A view that allows us to reuse the same code and content on multiple pages.
Partial view: Reusable section of code that can be inserted into other views.
Razor code block: A way to indicate Razor code that looks like this:
@{}
Using Layouts
Views/Shared/_Layout.cshtml
<!DOCTYPE html><html> <head> <meta charset="utf-8"> <title>My To-Do List!</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> <link rel="stylesheet" href="/css/styles.css"> </head> <body> @RenderBody() </body></html>
Using the layout in a view:
Views/Home/Index.cshtml
@{ Layout = "_Layout";}...
Using Partials
A sample partial for a footer:
Views/Shared/Footer.cshtml
<div id="footer">Bottom div content</div>
Adding the partial to another file:
Views/Shared/_Layout.cshtml
<body> @Html.Partial("Footer")</body>
Original Link: https://dev.to/saoud/c-basic-web-applications-3n1e

Dev To
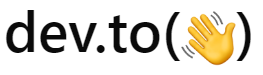
More About this Source Visit Dev To