JS interview in 2 minutes / this
Question:
Explain this
keyword in JavaScript.
Quick answer:this
keyword is referencing the current execution context.
Longer answer:this
works differently depending on where it was called.
If you use this
in the global context, it will reference the window
object in the browser and the global
object in the node.
// browserconsole.log(window.a) // undefinedthis.a = 1console.log(window.a) // 1// nodeconsole.log(global.a) // undefinedthis.a = 1console.log(global.a) // 1
For functions, it works similarly, but a still bit differently for the strict
mode.
function f1() { return this // default to global context}function f2() { 'use strict'; return this // undefined}
Arrow functions have their own tricks as well, they always refer to enclosing this
. We will get into details in the next section.
let f1 = function() { return this}let f2 = () => thisconsole.log(f1(), f2()) // Window, Windowlet obj = { f1, f2 }console.log(obj.f1(), obj.f2()) // obj reference, Window// ^^^ f1 changed reference, but f2 didn't
As for the class context, this
refers object itself
class Tmp { a = 10 method() { console.log(this) }}let tmp = new Tmp()tmp.method() // Tmp{a: 10}
Feels like these are the most popular cases, but there are much much more corner cases, take a look on mdn.
Real-life applications:
I think one of the most common caveats with this
is when you are using callbacks, which are popular in React and in Angular as well.
class User { _say(text) { console.log(text) } sayHello() { this._say('Hello world') } sayHi = () => this._say('Hi!')}let user = new User()user.sayHi() // Worksuser.sayHello() // WorkssetTimeout(user.sayHi, 1000) // Works// callback will show an error, because `this` reference will change// Uncaught TypeError: this._say is not a function at sayHellosetTimeout(user.sayHello, 1000)
So be careful and stay safe!
Resources:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this
Other posts:
- JS interview in 2 minutes / Encapsulation (OOP)
- JS interview in 2 minutes / Polymorphism (OOP)
- JS interview in 2 minutes / Inheritance in OOP
Btw, I will post more fun stuff here and on Twitter. Let's be friends
Original Link: https://dev.to/kozlovzxc/js-interview-in-2-minutes-this-3hlm

Dev To
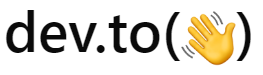
More About this Source Visit Dev To