API's From Dev to Production - Part 9
Series Introduction
Welcome to Part 9 of this blog series that will go from the most basic example of a .net 5 webapi in C#, and the journey from development to production with a shift-left mindset. We will use Azure, Docker, GitHub, GitHub Actions for CI/C-Deployment and Infrastructure as Code using Pulumi.
In this post we will be looking at:
- Static Code Analysis (SCA)
TL;DR
Static Code Analysis is an important step in the journey from dev to production; and continuing into other cycles such as feature development and more. With an abundance of tools out there on the market, there's bound to be one that suits you. CodeCov.io proved useful, but only for code coverage, by taking the next step and using SCA as well as code coverage, your API products will reap the benefits.
Quality should never take a back seat when building mission critical systems.
Requirements
We will be picking-up where we left off in Part 8, which means youll need the end-result from GitHub Repo - Part 8 to start with.
If you have followed this series all the way through, and I would encourage you to do so, but it isn't necessary if previous posts are knowledge to you already.
Introduction
We are looking at Static Code Analysis, another must have when we are going from development to production. These days there are a great number of tools out there from self-hosted to SaaS, for me, I'm more comfortable with less to manage and have opted for a SaaS solution; this certainly doesn't mean you have to. I highly recommend you checkout what is on the market and what will suite your needs.
We will be waving goodbye to CodeCov, there was a nasty breach and you can read more about that here and here. However, the move away is not solely because of that reason, the main reason is the SaaS solution we are going to integrate with provides SCA as well as code coverage :)
We will be integrating with Code Climate Quality
Let's get started!
Step 1
Head to their website Navigate to their Quality product and click Get Started. This will register Code Climate Quality as a GitHub App.
Once you have complete that journey, you'll be presented with the below - A list of all you public repositories.
Step 2
Add the repo of your choice, in our case, this will be, Samples.WeatherForecast-Part-9
.
As soon as you select your chosen repository, Code Climate Quality will starting doing its thing, just wait, have a cuppa tea :)
Once Code Climate Quality has completed its build and scan etc. you'll be informed about it with a nice little pop-up :)
Step 3
Navigate to your repository dashboard.
Checkout the stats - They look pretty good so far!
You'll notice the maintainability rating is currently an A. However, there is no code coverage!
Step 4
Let's fix the code coverage.
Now, Code Climate Quality states in their documentation or technically speaking, doesn't state that C# is supported... Sad face :(
However, let's not give up so quickly, looking at the supported code coverage formats, we should be...covered :D
As we have learnt even in this blog series, lcov
is what we are currently using, and we can also generate Cobertura
as well; these are supported formats.
We need to feed Code Climate Quality the lcov
file, just like we did with our departed CodeCov (don't forget to remove CodeCov from your GitHub Workflows).
Step 4.1
Navigate to Code Climate Quality Your repo Repo Settings Test Coverage.
You'll see your TEST REPORTER ID
, we need to put this into our GitHub Secrets area.
You should know how to do this by now, put it into your secrets for your repo and call it,
CC_TEST_REPORTER_ID
.
Step 4.2
Let's add a new step to our GitHub Action CI Workflow.
Either remove CodeCov.io or before/after the job step, place the following code:
- name: Code coverage [Code Climate] uses: paambaati/[email protected] env: CC_TEST_REPORTER_ID: ${{secrets.CC_TEST_REPORTER_ID}} with: coverageLocations: ${{ github.workspace }}/path/to/artifacts/testresults/coverage.info:lcov prefix: /code/
We have the environment set to our test reporter id so it know which repo in Code Climate Quality to link it to.
We've specified the coverage file, please note that there is a,
:lcov
at the end to state that it is indeed anlcov
file.The prefix parameter is purely required because of how we have built with Docker.
Prefix can refer to sub-commands of the test reporter.
We need to use this simply because when we compiled our code inside our Dockerfile, we copied to a local directory inside Docker, we, or more like I, chose to copy to, /code
by setting the working directory like so, WORKDIR /code
, and then copying the code and building. This means all the paths inside the lcov
file will point to /code
. However, inside the GitHub repository, that directory does not exist.
Very luckily (and with some trial and error on my part), prefix: /code/
resolves this issue :)
Step 5
Make any commit, or simply trigger the CI workflow.
Head on over to Code Climate Quality and you should have code coverage stats in your dashboard like so:
Step 6
Code Climate Quality has a GitHub Bot.
Let's set this up...
Click Set up (Pull request comments)
Install the GitHub App...
Confirm the permissions you've set...
Select Post additional inline comments on new issues
Toggle On Inline issue comments
Step 7
Let's test out Code Climate Quality by making some obvious issues and see how it handles it, plus we can test out the developer experience.
Step 7.1
Modify the, WeatherForecastController.cs
Change Get()
method...
[HttpGet]public IEnumerable<WeatherForecast> Get(){ var rng = new Random(); var temps1 = Enumerable.Range(1, 5).Select(index => new WeatherForecast { Date = DateTime.Now.AddDays(index), TemperatureC = rng.Next(-20, 55), Summary = Summaries[rng.Next(Summaries.Length)] }) .ToArray(); var temps2 = Enumerable.Range(1, 5).Select(index => new WeatherForecast { Date = DateTime.Now.AddDays(index), TemperatureC = rng.Next(-10, 45), Summary = Summaries[rng.Next(Summaries.Length)] }) .ToArray(); return Enumerable.Concat(temps1, temps2);}
All we've done here is duplicate the amount of responses, but it's slightly different. Take note of the
TemperatureC
value in each. Hopefully this will be caught by Code Climate Quality as a duplication.
Step 7.2
For the next modification, let's change the WeatherForecast.cs
file.
Add New property called, TemperatureFahrenheit
.
public int TemperatureFahrenheit => 32 + (int)(TemperatureC / 0.5556);
This is identical, except for the property name, hopefully Code Climate Quality will pick this up as a duplication also.
Step 8
Raise a PR for all of your changes and with your new CI workflow you should see some action taken by Code Climate Quality...
As you can see from the image above, the Code Climate Quality GitHub Bot has made inline comments as it found a duplication, whoohoo!
However, don't jump for joy just yet, as this is the only duplication found, it seems it has not picked-up on the property body duplication :(
Step 9
Let's enable GitHub Status Checks.
Navigate to Code Climate Quality Your repo Repo Settings GitHub Pull request status updates Click Edit Toggle On
Back in GitHub, head over to your repository Branches Branch Rule Check the below items so GitHub knows what required:
A handy thing in Code Climate Quality is you can see issues within its product itself, not just GitHub, so you have 2 views to look at.
By resubmitting the PR, you will see the GitHub Status Checks shine - Note I still have CodeCov.io in there, but it will be gone in subsequent posts.
Given the status checks are true/false - Code Climate Quality has flagged the duplication issues and now I need to approve this impact to the codebase. For this example, I will approve so we can see what happens.
This is a key moment to take note of, by using this workflow we can easily assess the impact and approve or deny, keeping track of codebase health has now never been more simple :)
Codebase Impact
As we expected, we have made things worse! We have duplicated code, our maintainability has gone down, therefore, our rating has dropped from A to B; naturally our test coverage has dropped down as well.
Technical Debt View
A nice view in Code Climate Quality is the Technical Debt graph, it needs to be taken with a pinch of salt in terms resolution time and all, however, I do see how this can be useful from time to time.
What have we learned?
We have learned about why Static Code Analysis is important and how an optimised workflow using SaaS tools such as Code Climate Quality can impact an engineering teams' daily lives. This is part of the journey to production, releasing without knowing elements such as maintainability and code coverage can so easily create issues down the line, especially with missions critical systems.
Understanding maintainability and test coverage are vitally important to the quality of the products engineering teams' produce.
CodeCov.io has now been removed.
Next up
Part 10 in this series will be about:
- SAST - Static Application Security testing is used to secure software by reviewing the source code of the software to identify sources of vulnerabilities.
More information
Original Link: https://dev.to/newday-technology/api-s-from-dev-to-production-part-9-43fd

Dev To
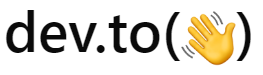
More About this Source Visit Dev To