An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
Create react subcomponents in a simple way!
Hello Folks,
If you are working in react and you have used libraries like React Bootstrap you must have seen or used components like <Dropdown.Item>
. Have you ever wondered how to create such components?
In this article, we will understand creating components which have their own modules or subcomponents, just like above Dropdown
component.
Note: If there is any particular name or jargon for subcomponents, please let me know that in comments below.
For this tutorial, we will be creating a very simple Card component. This will have Header, Body and Footer as its submodules. For the tutorial purpose, I am keeping this card component very simple and not adding any complex functionality to it. In the practical world, you may add all other features to it just like any other component in react. Though this tutorial is using plain ReactJS, you can use the same component structure for react-native apps as well.
The card component we will be creating will look something like this -
Now, let's see the code first and understand its structure better. Below is the code we used to create the above component.
import React from 'react';const Card = ({ children }) => { let subComponentList = Object.keys(Card); let subComponents = subComponentList.map((key) => { return React.Children.map(children, (child) => child.type.name === key ? child : null ); }); return ( <> <div className='card'> {subComponents.map((component) => component)} </div> </> );};const Header = (props) => <div className='card-header'>{props.children}</div>;Card.Header = Header;const Body = (props) => <div className='card-body'>{props.children}</div>;Card.Body = Body;const Footer = (props) => <div className='card-footer'>{props.children}</div>;Card.Footer = Footer;export default Card;
As you can see, in the above code, we are treating the card component as just another object is javascript. Our subcomponents, Header, Body and Footer are passed to the Card component as its keys.
Hence, inside the card, we will be first creating the list of all the keys i.e. subcomponents for the card. This way, we can add as many subcomponents as we want.
After getting the list of subcomponents, all we require is to render them through Card. Here, we will make use of React.Children api from React. React.Children provides a utility for dealing with opaque data structures the children props have. If, children prop is an array, it will return a function for each child in an array. If child is null
or undefined
, this method will return null
or undefined
.
The div element inside the return statement is used to add styling to the card and inside that div, we are just returning all the sub-components. We can also add more functionalities to this as per our requirements.
In this way, you can create any component with many subcomponents or modules inside it. Although, it is easy to create a single component and add all subcomponent functionalities inside it, but creating components this way will add much more readability to the code. So, depending on the use-cases, we can use this pattern in our apps.
If you have ever tried creating subcomponents like this or feel this can be done in a better way, do share your approaches with me in comments!
And your feedback on article will be welcomed, always!!
Keep learning!
Original Link: https://dev.to/ms_yogii/create-react-subcomponents-in-a-simple-way-5h1f

Dev To
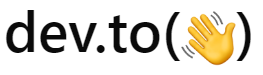
More About this Source Visit Dev To