How To Download A File With Node.js
Photo by Savannah Wakefield on Unsplash
Over the weekend I made a dependency update of my time tracking app Tie Tracker (PWA / GitHub).
In this particular tool, I defer the heavy work to Web Workers so that the UI does not find itself in a blocking state.
Because the app is meant to work offline and, is available in the App Store and Google Play, I did not import the required workers dependencies through a CDN but, locally.
The app itself is developed with React but, I implemented the workers with vanilla JavaScript and no package manager to handle their dependencies.
Therefore, I had to come with a solution to update the libs with aNode.js script .
Node Fetch
There is no window.fetch
like API in Node.js but, there is a light-weight module that brings such capabilities. Thats why I used node-fetch to implement the download of the file.
npm i node-fetch --save-dev
Script
The script I developed to update my dependencies is the following:
const {createWriteStream} = require('fs');const {pipeline} = require('stream');const {promisify} = require('util');const fetch = require('node-fetch');const download = async ({url, path}) => { const streamPipeline = promisify(pipeline); const response = await fetch(url); if (!response.ok) { throw new Error(`unexpected response ${response.statusText}`); } await streamPipeline(response.body, createWriteStream(path));};(async () => { try { await download({ url: 'https://unpkg.com/...@latest/....min.js', path: './public/workers/libs/....min.js', }); } catch (err) { console.error(err); }})();
The above download
function uses a stream pipeline to download a file, as displayed in the node-fetch README, and the built-in fs
module to write the output to the file system.
Top Level Await is available as of Node.js v14.8.0 but, I used an immediate function because I integrated it in a chain in which it was not available yet.
Thats it
Continue Reading
If you want to read more about React and Web Workers, I published back to back three blog posts about it last year .
To infinity and beyond!
David
You can reach me on Twitter or my website.
Give a try to DeckDeckGo for your next presentations!
Original Link: https://dev.to/daviddalbusco/how-to-download-a-file-with-node-js-1kh7

Dev To
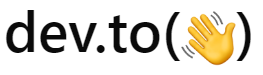
More About this Source Visit Dev To