An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
Finding a single item in an array
If we have an array, and we want to find the item(s) that appears once in the array.
const arr = [1,1,2,2,4,5,4]
The single item in the array above is 5, how can we solve this using JavaScript? We can use a hashmap aka Hash table, we need to convert the array to an object with the item as the key and their number of occurrence as the value.
const hashMap = {};for (i of arr) { if (hashMap[i]) { hashMap[i]++; } else { hashMap[i] = 1; }}console.log(hashMap)//
We declared an empty object, and loop through the array, our array item is passed in as the key, and we check to see if this item appears in the array more than once, if it does, we increment the value, if it appears once we set the value to 1.
We get the result below
{ '1': 2, '2': 2, '4': 2, '5': 1 }
As we can see 5 is the only number that appears once in the array, with a value of 1.
We need to loop through the object and get the keys with the value of 1, then push it into an array.
const single = []for (i in hashMap) { if (hashMap[i] === 1) { single.push(Number(i)); }}console.log(single)
The single item in the array is a string, it is converted to a number as we push it into the array.
We get the value below.
[ 5 ]
Thank you for reading.
Original Link: https://dev.to/bjhaid_93/finding-a-single-item-in-an-array-2kol

Dev To
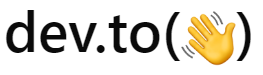
More About this Source Visit Dev To