An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
August 14, 2020 12:21 am GMT
Original Link: https://dev.to/viniciusmdias/easy-start-of-a-typescript-react-project-w-eslint-and-prettier-55d4
Easy start of a Typescript/React project (w/ ESlint and Prettier)
In this super fast tutorial, I'll teach you to initialize a Typescript project in React.
Create project
- Create project with create-react-app:
yarn create react-app *your-application-name* --template=typescript
ESlint
- Add ESlint to the project on the development mode:
yarn add eslint -D
- Start a new eslint file:
yarn eslint --init
- Choose these answers for the above command:
1. To check syntax, find problems, and enforce code style2. JavaScript modules (import/export)3. React4. Yes5. Browser6. Use a popular style guide7. Airbnb8. JSON9. No
- Install with Yarn the list of required dependencies that appear after denying
intall with npm
in the last choice of above command:
yarn add eslint-plugin-react@^7.20.0 @typescript-eslint/eslint-plugin@latest eslint-config-airbnb@latest eslint@^5.16.0 || ^6.8.0 || ^7.2.0 eslint-plugin-import@^2.21.2 eslint-plugin-jsx-a11y@^6.3.0 eslint-plugin-react-hooks@^4 || ^3 || ^2.3.0 || ^1.7.0 @typescript-eslint/parser@latest -D
be careful the dependencies can change.
- Install ESlint in the version 6.6.0 to avoid incompatibility problems with the react-scripts library:
yarn add [email protected] -D
- Create a file
.eslintignore
in the root of the project.
.eslintignore
:
**/*.jsnode_modulesbuild
- Add the following library in the development mode, to import typescript by default:
yarn add eslint-import-resolver-typescript -D
- Add some configurations in file
eslintrc.json
.
eslintrc.json
:
{ ... "extends": [ ... "plugin:@typescript-eslint/recomended" ], ... "plugins": [ ... "react-hooks" ], "rules": { "react-hooks/rules-of-hooks": "error", "react-hooks/exhaustive-deps": "warn", "react/jsx-filename-extension": [ 1, { "extensions": [ ".tsx" ] } ], "import/prefer-default-export": "off", "import/extensions": [ "error", "ignorePackages", { "ts": "never", "tsx": "never", } ] }, "settings": { "import/resolver": { "typescript": {} } }}
...
represents the code already in the file.
Prettier
- Add Prettier to the project on the development mode:
yarn add prettier eslint-config-prettier eslint-plugin-prettier -D
- Integrate the prettier with eslint by adding a few more settings to the file
eslintrc.json
.
eslintrc.json
:
{ ... "extends": [ ... "prettier/@typescript-eslint/recomended", "plugin:prettier/recomended", ], ... "plugins": [ ... "prettier" ], "rules": { ... "prettier/prettier": "error", }, ...}
- Create a file
prettier.config.js
in the root of the project.
prettier.config.js
:
module.exports = { singleQuote: true, trailingComma: 'all', allowParens: 'avoid',}
Bonus - EditorConfig
- Right-click on the project's root directory, generate the Editor config and change the last two fields to true and add the field
end_of_line = lf field
.
.editorconfig
:
root = true[*]indent_style = spaceindent_size = 2charset = utf-8trim_trailing_whitespace = trueinsert_final_newline = trueend_of_line = lf
- Start the project in the development mode:
yarn start
That's it. Both configuration files for Prettier and ESLint can be adjusted to your needs. If you need to add rules, you can do it with both files.
Enjoy!
Original Link: https://dev.to/viniciusmdias/easy-start-of-a-typescript-react-project-w-eslint-and-prettier-55d4
Share this article:
Tweet
View Full Article

Dev To
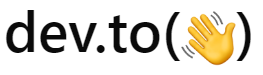
More About this Source Visit Dev To