How to convert objects into arrays in Javascript
Gone are the days when transforming Javascript objects
into arrays
required complex logic. Starting with E2017(ES8), we can do just that pretty easily. Depending on our needs, we can convert an object into an array containing the keys
, the values
or the whole key-value
pairs. Let's see how this works. We are going to use the Object
class and some specific static methods found on this class. These are: Object.keys()
, Object.values()
and Object.entries()
.
// We have an objectconst pairs = { Key1: "value1", Key2: "value2", Key3: "value3"}// Converting the object into an array holding the keysconst arrayOfKeys = Object.keys(pairs);console.log(arrayOfKeys);// prints [Key1, Key2, Key3]// Converting the object into an array holding the valuesconst arrayOfValues = Object.values(pairs);console.log(arrayOfValues);// prints [value1, value2, value3]// Converting the object into an array holding the key-value pairsconst arrayOfCompleteEntries = Object.entries(pairs);console.log(arrayOfCompleteEntries);// prints [[Key1, value1], [Key2, value2], [Key3, value3]];
As we can see, in the last example where we are extracting the key-value
pairs we end up with an array of arrays. If we want to work with each key
or value
from the original object, we can use forEach
to loop through every sub-array
:
arrayOfCompleteEntries.forEach(([key, value]) => { console.log(key); console.log(value);})// prints Key1value1Key2value2Key3value3
If for some reason we want to convert the newly created array back into an object, we can do that very easily like this:
const backToObject = Object.fromEntries(arrayOfCompleteEntries);console.log(backToObject);// prints{Key1:"value1",Key2:"value2",Key3:"value3"}
Image source: Adeolu Eletu/ @adeolueletu on Unsplash
Original Link: https://dev.to/cilvako/how-to-convert-objects-into-arrays-in-javascript-5gm

Dev To
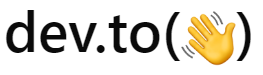
More About this Source Visit Dev To