An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
How JavaScript Variables Should Be Named
Lets talk about how your JS variables should be named.
Well cover what the language doesnt allow you to do and how you should name variables so you and other developers can easily know what they contain:
Case-Sensitive
Lets start with a questionIll create three variables name, one lowercase, one capitalize and one with all caps:
const name = "Jane";const Name = "Mark";const NAME = "Fred";
Are these the same variable? What do you think? Will I get an error when I console log each of these variables?
console.log(name, Name, NAME); // "Jane" "Mark" "Fred"
These are all valid variables name, despite their different. We can see that variables, first of all, are case-sensitive. They can have the same sequence of letters, but casing matters.
Self-Descriptive
Second, variable names should be clear about what they contain.
Lets say we are looking at someone elses code and see this:
let newValue = `${value1} ${value2}`;
Do you know whats taking place? Two values are being added, but you have no idea what or what data type they are.
Now if the names are improved, for example:
let fullName = `${firstName} ${lastName}`;
We see and understand exactly whats going on. We can infer from these names, as well as that they are strings and fullName will be a string as well. So variable identifiers should be self-descriptive and not require comments for others to know what they hold.
Third, variables should in most cases be written in camel syntax, where the first word in the name lowercase, and the rest uppercase:
let firstname;let first_name;let FIRSTNAME;let FIRST_NAME;let firstName;
Which of these are correctly written in camel case? Just the last one.
The camel case convention is the most important rule to remember when writing JS variables, but there are other helpful conventions, meaning ones that are helpful to follow because of what they signal to developers, but are not required by the language itself.
Boolean naming convention
Since JS is a loosely typed language, meaning any variable can contain any data type, the way we name variables can tell other developers what type of data it should hold.
Lets say we control whether a modal or popup is visible with a variable. If a variable is true, its visible, if false, its not.
To tell other developers exactly what this does, we could have a variable called isModalVisible
. Why prefix it with is? Not only does it tell us it contains a boolean, but is easy to read in conditionals. If we want to do something if the modal was visible, our code would be this:
if (isModalVisible) { // do this}
Now this conditional reads just like a sentence. The closer we can get our code to reading like it was plain instructions, the better.
To figure out the prefix, put the variable in a conditional and read what it says. Usually variables that hold booleans are prefixed with is, has:
let isLoading;let hasPosition;
Constant naming convention
Another valuable convention is for variables whose values should never change. We already know that we have the const keyword to create variables that can never be reassigned. However, for variables whose value should never be changed by other developers manually, either, we write the variable names in all caps.
For example, we might have the hex color red saved in a variable to easily reference it. The color red is never going to change and doesnt need to be updated so we can use all caps:
const COLOR_RED = "#F00";
And note that unlike camelcase, for all caps constants, we separate words in the identifier with underscores
Summary
Lets review:
- Variables are case-sensitive
- Their names should be self-descriptive; we should know exactly what they hold based on its name, and from that have an idea about what data type that is
- Most every JS variable you write will be in camelCase
- Since variables can hold anything and can be changed easily, we use conventions to give more information to other developers,
- Like using is or has to prefix variables that hold booleans
- And using all caps for variables that hold values that should never change
Want To Become a JS Master? Join the 2020 JS Bootcamp
Follow + Say Hi! Twitter Instagram reedbarger.com codeartistry.io
Original Link: https://dev.to/codeartistryio/how-javascript-variables-should-be-named-13hm

Dev To
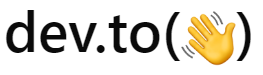
More About this Source Visit Dev To