An Interest In:
Web News this Week
- April 19, 2024
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
What's the Deal with Arrays and Objects?
We all know arrays and objects, right? Sure, but what are some key differences between the two? Let's start with some ways that the two are similar: well, they're both complex data types for starters, meaning that they can hold a multitude of other datatypes within themselves, including other arrays and objects! Both also a have way to loop through the data they contain.
Here's two examples that we'll use throughout this reading:
let dataTypesArr = ['string', 1, true, ['array'], {key: 'value'}];let dataTypesObj = { string: 'string', number: 4, boolean: true, array: [1, 2, 3], object: {inner: true}};
Access... Granted?
We now know that these two datatypes can hold other datatypes, but how do we access them? This is where some key differences begin to show.
Arrays
Arrays can be accessed by using bracket notation. Since arrays can have their data organized, they contain an index which keeps track of what is where. They also have a length property which is handy for looping.
console.log(dataTypesArr[0]); //This prints the value held at the //0 index which is 'string' here//For loops allow us to access all of the data if we wanted tofor(let i = 0; i < dataTypesArr.length; i++){ console.log(dataTypesArr[i])}//This loop prints whatever value is held at the i index during//that iteration, and it runs until it is out of data to iterate//through
Objects
Objects have the ability to be accessed using bracket or dot notation. Keep in mind if you decide to use bracket notation for accessing objects, you have to include the quotes inside the brackets to notate you want to access that literal keyName. They do not have a length property and their data is not organized. Objects also have a special way to loop through their stored data.
console.log(dataTypesObj.number); //Prints value held at the //'number' key, which is 4console.log(dataTypesObj['boolean']); //Prints value held at the //'boolean' key, which is true//To loop through an object, you have to use a for-in loopfor(let key in dataTypesObj){ console.log(key); //This prints all of the keys console.log(dataTypesObj[key]);} //Here you wouldn't put key in quotes because key is used as a//variable. If you used quotes, the program would try to find the//key named 'key'. This will print all of the values held at each//key.
Manipulation?
Now we know how to access them, but how can we manipulate the data with arrays and objects? Easy, actually. Both types have their own ways to change, or mutate, their data
Arrays
Starting with arrays again, you can put new data in, or take it out. People most commonly use array methods to accomplish this.
//.push() and .unshift() add data to the end and beginning of the//array respectivelydatatypesArr.push(5); //adds 5 to the end of the arraydatatypesArr.unshift(10); //adds 10 to the beginning of the array//.pop() and .shift() remove data from the end and beginning of//the array respectivelydatatypesArr.pop(); //removes the last valuedatatypesArr.shift(); //removes the first value
Objects
Objects are really easy to manipulate, no methods needed!
//Say we want to add a new piece of data called namedataTypesObj.name = 'Dakota'; //Adds new key/value pair: //name: 'Dakota'dataTypesObj['age'] = 27; //Also works with bracket notation!//If we wanted to delete our newly created data, just use the//delete keyworddelete dataTypesObj.name; //deletes name: 'Dakota'delete dataTypesObj['age']; //deletes age: 27
Conclusions
Just remember, arrays and objects are really only similar in the way that they are complex data types and that they can loop. They each have their own ways to loop, access, and manipulate the inner data. Hopefully this information helps if you're struggling to get the hang of arrays and objects. Good luck on your own coding adventure!
Original Link: https://dev.to/dakota_day/whats-the-deal-with-arrays-and-objects-5dlj

Dev To
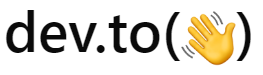
More About this Source Visit Dev To