An Interest In:
Web News this Week
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
April 18, 2024 03:56 am GMT
Original Link: https://dev.to/hyperkai/minimum-maximum-kthvalue-and-topk-in-pytorch-59ak
minimum(), maximum(), kthvalue() and topk() in PyTorch
minimum() can get the zero or more minimum values from 2 tensors as shown below:
*Memos:
minimum()
can be called both from torch and a tensor.minimum()
can be used with 0D or more D tesnors.- 2 tensors must be the same size.
- Only zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
import torchtensor1 = torch.tensor([[7, 1, 4], [5, 3, 0]])tensor2 = torch.tensor([[3, 5, 1], [9, 0, 6]])torch.minimum(tensor1, tensor2)tensor1.minimum(tensor2)# tensor([[3, 1, 1], [5, 0, 0]])tensor1 = torch.tensor([[7., 1., 4.], [5., 3., 0.]])tensor2 = torch.tensor([[3., 5., True], [9., False, 6.]])torch.minimum(tensor1, tensor2)tensor1.minimum(tensor2)# tensor([[3., 1., 1.], [5., 0., 0.]])
maximum() can get the zero or more maximum values from 2 tensors as shown below:
*Memos:
maximum()
can be called both fromtorch
and a tensor.maximum()
can be used with 0D or more D tesnors.- 2 tensors must be the same size.
- Only zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
import torchtensor1 = torch.tensor([[7, 1, 4], [5, 3, 0]])tensor2 = torch.tensor([[3, 5, 1], [9, 0, 6]])torch.maximum(tensor1, tensor2)tensor1.maximum(tensor2)# tensor([[7, 5, 4], [9, 3, 6]])tensor1 = torch.tensor([[7., 1., 4.], [5., 3., 0.]])tensor2 = torch.tensor([[3., 5., True], [9., False, 6.]])torch.maximum(tensor1, tensor2)tensor1.maximum(tensor2)# tensor([[7., 5., 4.], [9., 3., 6.]])
kthvalue() can get the one or more k
th smallest elements and their indices of a 0D or more D tensor as shown below:
*Memos:
kthvalue()
can be called both fromtorch
and a tensor.- The 2nd argument with
torch
or the 1st argument with a tensor isk
(Required). - The 3rd argument with
torch
or the 2nd argument with a tensor isdim
(Optional) which is a dimension. - The 4th argument with
torch
or the 3rd argument with a tensor iskeepdim
(Optional-Default:False
) which keeps the dimension. - Only one or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
- If there are the multiple same
k
th values, one is returned nondeterministically.
import torchmy_tensor = torch.tensor([5, 1, 9, 7, 6, 8, 0, 5])torch.kthvalue(my_tensor, 3)my_tensor.kthvalue(3)torch.kthvalue(my_tensor, 3, 0)my_tensor.kthvalue(3, 0)torch.kthvalue(my_tensor, 3, -1)my_tensor.kthvalue(3, -1)# torch.return_types.kthvalue(# values=tensor(5),# indices=tensor(7))torch.kthvalue(my_tensor, 3, 0, True)# torch.return_types.kthvalue(# values=tensor([5]),# indices=tensor([7]))torch.kthvalue(my_tensor, 4)my_tensor.kthvalue(4)torch.kthvalue(my_tensor, 4, 0)my_tensor.kthvalue(4, 0)torch.kthvalue(my_tensor, 4, -1)my_tensor.kthvalue(4, -1)# torch.return_types.kthvalue(# values=tensor(5),# indices=tensor(0))torch.kthvalue(my_tensor, 4, 0, True)# torch.return_types.kthvalue(values=tensor([5]),indices=tensor([0]))my_tensor = torch.tensor([[5, 1, 9, 7], [6, 8, 0, 5]])torch.kthvalue(my_tensor, 3)my_tensor.kthvalue(3)torch.kthvalue(my_tensor, 3, 1)my_tensor.kthvalue(3, 1)torch.kthvalue(my_tensor, 3, -1)my_tensor.kthvalue(3, -1)# torch.return_types.kthvalue(# values=tensor([7, 6]),# indices=tensor([3, 0]))torch.kthvalue(my_tensor, 3, 1, True)# torch.return_types.kthvalue(# values=tensor([[7], [6]]),# indices=tensor([[3], [0]]))my_tensor = torch.tensor([[5., True, 9., 7.], [6, 8, False, 5]])torch.kthvalue(my_tensor, 1)my_tensor.kthvalue(1)# torch.return_types.kthvalue(# values=tensor([1., 0.]),# indices=tensor([1, 2]))
topk() can get the zero or more k
largest or smallest elements and their indices of a 0D or more D tensor as shown below:
*Memos:
topk()
can be called both fromtorch
and a tensor.- The 2nd argument with
torch
or the 1st argument with a tensor isk
(Required). - The 3rd argument with
torch
or the 2nd argument with a tensor isdim
(Optional) which is a dimension. - The 4th argument with
torch
or the 3rd argument with a tensor islargest
(Optional-Default:True). *True
gets the zero or more largest elements whileFalse
gets the zero or more smallest elements. - The 5th argument with
torch
or the 4th argument with a tensor issorted
(Optional-Default:True). *Sometimes, a return tensor is sorted withFalse
but sometimes not so make itTrue
if you want to definitely get a sorted tensor. - Only one or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
- If there are the multiple same
k
values, one or more ones are returned nondeterministically.
import torchmy_tensor = torch.tensor([5, 1, 9, 7, 6, 8, 0, 5])torch.topk(my_tensor, 3)my_tensor.topk(3)torch.topk(my_tensor, 3, 0)my_tensor.topk(3, 0)torch.topk(my_tensor, 3, -1)my_tensor.topk(3, -1)# torch.return_types.topk(# values=tensor([9, 8, 7]),# indices=tensor([2, 5, 3]))torch.topk(my_tensor, 3, 0, False)my_tensor.topk(3, 0, False, True)# torch.return_types.topk(# values=tensor([0, 1, 5]),# indices=tensor([6, 1, 0]))torch.topk(my_tensor, 3, 0, False, False)my_tensor.topk(3, 0, False, False)# torch.return_types.topk(# values=tensor([1, 0, 5]),# indices=tensor([1, 6, 0]))torch.topk(my_tensor, 4)my_tensor.topk(4)torch.topk(my_tensor, 4, 0)my_tensor.topk(4, 0)torch.topk(my_tensor, 4, -1)my_tensor.topk(4, -1)# torch.return_types.topk(# values=tensor([9, 8, 7, 6]),# indices=tensor([2, 5, 3, 4]))torch.topk(my_tensor, 4, 0, False)my_tensor.topk(4, 0, False)# torch.return_types.topk(# values=tensor([0, 1, 5, 5]),# indices=tensor([6, 1, 0, 7]))torch.topk(my_tensor, 4, 0, False, False)my_tensor.topk(4, 0, False, False)# torch.return_types.topk(# values=tensor([1, 0, 5, 5]),# indices=tensor([1, 6, 0, 7]))my_tensor = torch.tensor([[5, 1, 9, 7], [6, 8, 0, 5]])torch.topk(my_tensor, 3)my_tensor.topk(3)torch.topk(my_tensor, 3, 1)my_tensor.topk(3, 1)torch.topk(my_tensor, 3, -1)my_tensor.topk(3, -1)# torch.return_types.topk(# values=tensor([[9, 7, 5], [8, 6, 5]]),# indices=tensor([[2, 3, 0], [1, 0, 3]]))torch.topk(my_tensor, 3, 1, False)my_tensor.topk(3, 1, False)# torch.return_types.topk(# values=tensor([[1, 5, 7], [0, 5, 6]]),# indices=tensor([[1, 0, 3], [2, 3, 0]]))torch.topk(my_tensor, 3, 1, False, False)my_tensor.topk(3, 1, False, False)# torch.return_types.topk(# values=tensor([[1, 5, 7], [5, 0, 6]]),# indices=tensor([[1, 0, 3], [3, 2, 0]]))my_tensor = torch.tensor([[5., True, 9., 7.], [6, 8, False, 5]])torch.topk(my_tensor, 4)my_tensor.topk(4)# torch.return_types.topk(# values=tensor([[9., 7., 5., 1.], [8., 6., 5., 0.]]),# indices=tensor([[2, 3, 0, 1], [1, 0, 3, 2]]))
Original Link: https://dev.to/hyperkai/minimum-maximum-kthvalue-and-topk-in-pytorch-59ak
Share this article:
Tweet
View Full Article

Dev To
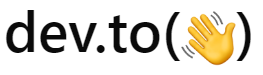
More About this Source Visit Dev To