An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Understanding Type Annotations in TypeScript
TypeScript is a superset of JavaScript that adds optional static typing to the language. One of the most powerful features of TypeScript is its type annotation system, which allows developers to explicitly specify the types of variables, function parameters, and return values. Type annotation will let developers slap labels on variables, parameters, and return values, allowing them to say, "Hey, you're definitely a number!" or "I see you're a string!".
What's the Heck is Type Annotation?
Imagine you're baking a cake, and you need precisely four eggs. You wouldn't just grab a handful of eggs without counting, right? Type annotation is like counting your ingredients to ensure you have the right stuff for your recipe. It involves explicitly stating the type of data each variable or function expects or returns. It's that simple!!
In TypeScript, type annotation follows a simple formula:
let variableName: type;
Here, variableName
is the name of your ingredient (variable), and type is the label telling you what type of ingredient it is. TypeScript offers different types: number, string, boolean, and more.
Still on our baking exercise, let's cook up some examples of type annotation.
let eggs: number = 3;let flour: number = 250; // taking this in grams
In this baking exercise, eggs
and flour
are explicitly labeled as number types. TypeScript helps ensure we're dealing with the right ingredients.
Another example:
For example, I want to create a player in a game, and I want to create a playerName, playerLevel and know if the player is still alive, I can easily declare this variable in TypeScript as below:
let playerName: string = "Hero";let playerLevel: number = 5;let isAlive: boolean = true;
In our gaming world, playerName
is a string, playerLevel
is a number, and isAlive
is a boolean. TypeScript helps us keep track of our player's vital stats.
Why should I use Type Annotation?
Type annotation explicitly specifies the types of variables, function parameters, and return values in your TypeScript code. By doing so, you gain several benefits that can significantly improve the quality, maintainability, and developer experience of your codebase. Here are some other benefits of Type annotation:
Code Clarity: By explicitly stating the data type of variables and functions, type annotation improves code readability and clarity. Anyone going through your codebase can easily understand the intended purpose of each variable and function.
Error Detection: Type annotation helps to catch type-related errors at compile-time rather than runtime. This h helps you to identify and fix issues early in the development process, reducing bugs and improving code robustness.
IDE Support: Many integrated development environments (IDEs) and text editors provide enhanced support for TypeScript, including features such as auto-completion, type checking, and code navigation. In essence, with type annotation in your utility belt, your IDE becomes your trusty sidekick, offering autocomplete suggestions, real-time error checking, and lightning-fast refactoring powers.
Refactoring: When refactoring code or making changes, type annotation provides valuable information about the expected types, enabling developers to make modifications confidently without introducing unintended side effects.
Documentation: Type annotations serve as documentation for the codebase, making it easier for you and any other developer to understand the structure and behavior of the application. This is especially helpful when working in teams or revisiting code after some time.
Exploring Use Cases with Real-Life Scenarios
Imagine you're developing an attendance management application for a school. Your task is to create a TypeScript application that allows administrators to add and manage different types of users students, teachers, and staff. With type annotation, you'll ensure that each user type is properly defined and handled within the application.
We would go ahead to harness the power of type annotation to define the different types of users.
Step 1: Define User Types with Interfaces
interface User { name: string; role: string; contact: string;}interface Student extends User { grade: number;}interface Teacher extends User { subject: string;}interface Staff extends User { department: string;}
We've defined interfaces for User
, Student
, Teacher
, and Staff
, each with specific properties. Type annotation ensures that each user type is distinct and follows a consistent structure.
Step 2: Implement Adding Users Functionality
function addUser(user: User): void { // Logic to add user to database console.log(`User ${user.name} added successfully!`);}
The addUser function accepts a User
object, ensuring that any user type can be added to the system. Type annotation guarantees that only valid user data is processed.
Step 3: Populate the database
let users: User[] = [];const student: Student = { name: "Alice", role: "Student", contact: "[email protected]", grade: 10};const teacher: Teacher = { name: "Mr. Smith", role: "Teacher", contact: "[email protected]", subject: "Mathematics"};const staff: Staff = { name: "Ms. Johnson", role: "Staff", contact: "[email protected]", department: "Administration"};users.push(student, teacher, staff);
With type annotation, our users
array can hold objects of different user types. TypeScript ensures that each user type is correctly represented and stored.
In this brief example through user management with TypeScript, type annotation proved to be of so much help. By explicitly defining user types and leveraging type annotation, we ensured that our application remained robust and easy to maintain. When adding users and populating the database, type annotation guided us every step of the way.
In summary, Type annotation isn't just a fancy term it's your secret weapon for writing robust, maintainable, and error-free code. By explicitly stating data types, you empower yourself and your team to build stronger, more reliable applications. Start harnessing the full potential of TypeScript using Type annotation in your projects!
Original Link: https://dev.to/osalumense/understanding-type-annotations-in-typescript-j19

Dev To
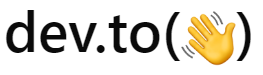
More About this Source Visit Dev To