An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
PYTHON FUNCTIONS
INTRODUCTION
A set of statements that returns the particular task is called a Python function. The concept is to group together some often performed activities into a function so that, rather than writing the same code repeatedly for various inputs, we can call the function calls repeatedly to reuse the code they contain.
A Few Advantages of Function Use
Boost the Readability of Code
Boost the Reusability of Code
Types of Python Functions
The different types of Python functions are listed below:
Built-in library function: These are accessible Standard functions in Python.
User-defined function: In accordance with our needs, we are able to design our own functions.
Creating a Python Function
The def
keyword in Python allows us to define a function. It can have any kind of functionality and property added to it that we need. The subsequent illustration enables us to comprehend the process of writing a function in Python. Using the def
keyword, we may define a Python function in this manner.
# A simple Python functiondef fun(): print("Welcome to python")
Invoking a Python Function
Once a function has been created in Python, it can be called by supplying its name in parentheses with the parameters of that specific function. The example of calling the def function in Python is shown below.
# A simple Python functiondef fun(): print("Welcome to python")# Driver code to call a functionfun()
Output:Welcome to python
Function in Python with Parameters
If you've worked with Java or C/C++ before, you probably already know about function return types and argument data types.
Arguments for Python Functions
Values given inside the function's parenthesis are called arguments. Any number of parameters can be passed to a function, separated by commas.
To determine whether the integer supplied as an argument to the function is even or odd, we will build a straightforward Python function in this example.
# A simple Python function to check# whether x is even or odddef evenOdd(x): if (x % 2 == 0): print("even") else: print("odd")# Driver code to call the functionevenOdd(2)evenOdd(3)
Outputevenodd
Types of Python Function Arguments
Python Function Argument Types
Python allows for the passing of several kinds of parameters when calling a function. The following function argument types are available in Python:
Default argument
Keyword arguments (named arguments)
Positional arguments
Arbitrary arguments (variable-length arguments *args and **kwargs)
DefaultArguments
When a value is not supplied in the function call for an argument, the argument is said to have a default value. The default arguments for writing functions in Python are demonstrated in the example that follows.
# Python program to demonstrate# default argumentsdef myFun(x, y=50): print("x: ", x) print("y: ", y)# Driver code (We call myFun() with only# argument)myFun(10)
#Outputx: 10y: 50
Keyword Arguments
In order to avoid the caller having to remember the parameter order, the idea is to let the caller declare the argument name along with values.
# Python program to demonstrate Keyword Argumentsdef student(firstname, lastname): print(firstname, lastname)# Keyword argumentsstudent(firstname='John', lastname='Doe')student(lastname='Doe', firstname='John')
#OutputJohn DoeJohn Doe
Positional Arguments
In order to assign the first parameter (or value) to the name and the second argument (or value) to the age, we used the Position argument during the function call. The values can be utilized incorrectly by shifting the positions or forgetting the order of the positions, as demonstrated in the Case-2 example below, where Suraj is allocated the age and 27 is assigned the name.
def nameAge(name, age): print("Hi, I am", name) print("My age is ", age)# You will get correct output because # argument is given in orderprint("Case-1:")nameAge("Suraj", 27)# You will get incorrect output because# argument is not in orderprint("
Case-2:")nameAge(27, "Suraj")
#OutputCase-1:Hi, I am SurajMy age is 27Case-2:Hi, I am 27My age is Suraj
Arbitrary Keyword Arguments
Using special symbols, *args and **kwargs in Python Arbitrary Keyword Arguments can pass a configurable number of arguments to a function. Two unique symbols are present:
Python's **kwargs (Keyword Arguments) and *args (Non-Keyword Arguments)
# Python program to illustrate# *args for variable number of argumentsdef myFun(*argv): for arg in argv: print(arg)myFun('Hello', 'Welcome', 'to', 'Python')
#OutputHelloWelcometoPython
Original Link: https://dev.to/maame-codes/python-functions-l11

Dev To
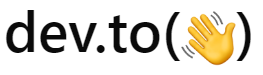
More About this Source Visit Dev To