An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Optimizing Classroom Time: Using Lyzr-Automata to Generate Effective Timetables
Juggling teacher availability, student needs, and subject requirements for your classroom can be quite a hassle. But what if there was a way to take the stress out of scheduling? In this blog post, we'll explore how Lyzr-Automata can help you build efficient, balanced, and fair timetables that meet the needs of your students and teachers.
Setup
Create a folder, set up a virtual environment and activate it. Create .env
file with your OPENAI_API_KEY. Then install the following libraries to get started.
Libraries
streamlit
: for building the web app interface.lyzr_automata
: for implementing our AI models, and tasks.dotenv
: for loading environment variables (API key).
lyzr-automata==0.1.2streamlit==1.33.0python-dotenv==1.0.1
Getting Started
We will split the task into 2 files. One for frontend components (main.py) while the other for Lyzr-Automata (lyzr_functions.py)
main.py
1.Import Libraries
import streamlit as stfrom lyzr_functions import generate_basic_timetable
2. Input Components
st.text_area
Text area to enter timeslots, subjects list and constraints.st.button
Button to submit input
# Time Slotstimeslot_input = st.text_area( "TIMESLOTS", '''08:00 AM - 09:00 AM09:15 AM - 10:15 AM10:30 AM - 11:30 AM11:45 AM - 12:45 PM01:00 PM - 02:00 PM02:15 PM - 03:15 PM03:30 PM - 04:30 PM04:45 PM - 05:45 PM''')# Subjectssubjects_input = st.text_area( "SUBJECTS", '''MathematicsScienceEnglishSocial StudiesHistoryGeographyPhysical EducationComputerArtMusic''')# Constraintsconstraints_input = st.text_area( "CONSTRAINTS", '''1. Mathematics - 5 classes a week2. Science - 5 classes a week3. English - 4 classes a week4. Social Studies - 4 classes a week5. History - 3 classes a week6. Geography - 3 classes a week7. Physical Education - 4 classes a week8. Computer - 5 classes a week9. Art - 2 class a week10. Music - 2 class a week11. Each classroom is allocated once per time slot on any given day.12. Same subjects cannot be assigned consecutively''')# Submit buttonsubmit_inputs = st.button("Submit", type="primary")
3. Handle Inputs
if submit_inputs: # Save inputs to a file with open("example_slot.txt", "w") as my_file: my_file.write("TIMESLOTS") my_file.write("
") my_file.write(timeslot_input) my_file.write("
") my_file.write("SUBJECTS") my_file.write("
") my_file.write(subjects_input) my_file.write("
") my_file.write("CONSTRAINTS") my_file.write("
") my_file.write(constraints_input) # Call timetable generator generate_basic_timetable() # Read and display from result file with open("example_result.txt", "r") as my_file: content = my_file.read() st.write(content)
lyzr_functions.py
1.Import Libraries
from lyzr_automata.ai_models.openai import OpenAIModelfrom lyzr_automata.memory.open_ai import OpenAIMemoryfrom lyzr_automata import Agent, Taskfrom lyzr_automata.tasks.task_literals import InputType, OutputTypefrom dotenv import load_dotenvimport osload_dotenv()OPENAI_API_KEY = os.getenv("OPENAI_API_KEY")
2. Initialize Model and Memory
OpenAIModel
Create our models using OpenAI Key and specify the model type and name.OpenAIMemory
timetable_memory, Create a memory of instructions for the agent.
# OpenAI Text Modelopen_ai_model_text = OpenAIModel(api_key=OPENAI_API_KEY,parameters={ "model": "gpt-4-turbo-preview", "temperature": 0.1, "max_tokens": 4096, },)# OpenAI Memory filetimetable_memory = OpenAIMemory( file_path='example_slot.txt')
3. generate_basic_timetable functions
Agent
timetable_agent, Lyzr Agent with instructions and persona to create timetable.Task
timetable_task, Lyzr Task to create timetable.Agent
timetable_checker_agent, Lyzr Agent with instructions and persona to verify timetable.Task
timetable_checker_task, Lyzr Task to verify if the timetable is valid.
def generate_basic_timetable(): # Remove file if exists if os.path.exists("assistant_ids.json"): os.remove("assistant_ids.json") # Timetable generator Agent timetable_agent = Agent( prompt_persona="You are an intelligent agent that can create efficient class timetables for a week in a simple, structured format. Do not assign more classes than required, assign free slots instead. Generate timetable for every day from Monday to Friday.", role="Timetable creator", memory=timetable_memory ) # Timetable generator Task timetable_task = Task( name="Timetable Creator", agent=timetable_agent, output_type=OutputType.TEXT, input_type=InputType.TEXT, model=open_ai_model_text, instructions="Using the time slots, subject details and requirements, create a timetable that satisfies every constraint. Return the timetable in a simple format and a count of number of classes scheduled for each subject.", log_output=True, enhance_prompt=False, ).execute() # Save output to a file with open("example_result.txt", "w") as my_file: my_file.write("# GENERATED TIMETABLE - Timetable Agent") my_file.write("
") my_file.write(timetable_task) my_file.write("
") # Timetable verification Agent timetable_checker_agent = Agent( prompt_persona="You are an intelligent agent that can verify if a generated timetable fulfills all the constraints or not. Make sure all classes meet the exact requirements; not more, not less.", role="Timetable checker", memory=timetable_memory ) # Timetable verification Task timetable_checker_task = Task( name="Timetable Checker", agent=timetable_checker_agent, output_type=OutputType.TEXT, input_type=InputType.TEXT, model=open_ai_model_text, instructions="Verify that the generated timetable input fulfills the requirements mentioned in the file. If VALID, return <!VALID!> and the timetable in a table format; If INVALID, return <!INVALID!> the reason why invalid. Do not return anything else.", log_output=True, enhance_prompt=False, previous_output=timetable_task ).execute() # Save output to a file with open("example_result.txt", "a") as my_file: my_file.write("
") my_file.write("# GENERATED VERIFICATION - Verification Agent") my_file.write("
") my_file.write(timetable_checker_task) my_file.write("
")
Run App
streamlit run main.py
Flow Diagram
Want to create more of such amazing AI Workflows? Visit our website at GitHub to learn more about Lyzr-Automata!
Also checkout Lyzr SDKs at GitHub
Lyzr Website: Lyzr.ai
Lyzr Community Channel: Discord
Code: https://github.com/rasswanth-lyzr/timetable_bot
Video Walkthrough: https://youtu.be/h9c-bL0a3TM
Demo: https://rasswanth-lyzr-timetable-bot-main-n3n6ul.streamlit.app/
Original Link: https://dev.to/iamrash/optimizing-classroom-time-using-lyzr-automata-to-generate-effective-timetables-17p7

Dev To
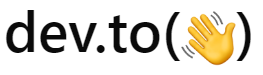
More About this Source Visit Dev To