An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Mastering SEO with React: Strategies and Code Insights
React is widely used in various web application developments, but understanding SEO optimization techniques is necessary. This article will explain the key elements of SEO optimization using React, with specific code examples.
Implementing Server-Side Rendering (SSR): Using SSR with Next.js can significantly enhance the SEO of a React app. For example, by pre-rendering pages on the server, search engines can easily recognize content at the initial load.
// pages/index.jsimport { useEffect, useState } from 'react';function Home() { const [data, setData] = useState(null); useEffect(() => { // Logic to pre-fetch data from the server fetch('/api/data') .then(response => response.json()) .then(data => setData(data)); }, []); return ( <div> <h1>Home Page</h1> <p>{data ? data.content : 'Loading...'}</p> </div> );}export default Home;
Dynamic Meta Tag Management: In React, you can use React Helmet to set different meta tags for each page. This is crucial for proper recognition and indexing by search engines like Google.
// components/SEO.jsimport { Helmet } from 'react-helmet';function SEO({ title, description }) { return ( <Helmet> <title>{title}</title> <meta name="description" content={description} /> </Helmet> );}export default SEO;
Code Splitting and Routing Optimization: Implementing code splitting using libraries like React Router allows loading only necessary components, reducing user loading times and enhancing SEO scores.
// App.jsimport React, { Suspense, lazy } from 'react';import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';const Home = lazy(() => import('./Home'));const About = lazy(() => import('./About'));function App() { return ( <Router> <Suspense fallback={<div>Loading...</div>}> <Switch> <Route exact path="/" component={Home} /> <Route path="/about" component={About} /> </Switch> </Suspense> </Router> );}export default App;
React can be very effective for SEO when the right technologies and strategies are used. Utilize server-side rendering, dynamic meta tag management, and code splitting to meet search engine requirements and optimize user experience.
Related Posts
Original Link: https://dev.to/function12_io/mastering-seo-with-react-strategies-and-code-insights-2e4

Dev To
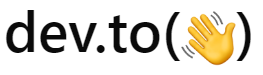
More About this Source Visit Dev To