An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Generate Chapter Notes with Lyzr ChatAgent,Summarizer,OpenAI and Streamlit
In today's fast-paced world, students and educators are constantly seeking efficient ways to comprehend and summarize vast amounts of information. With the advancements in artificial intelligence (AI) and natural language processing (NLP), creating automated tools to generate chapter notes has become a reality.
This blog post will introduce you to a cool web app built with Streamlit! This app, called Lyzr Chapter Notes Generator, helps you automatically summarize chapters from your study materials.
How itWorks:
Choose Your Input: The app offers a default pre-loaded chapter or lets you upload your own PDF file.
Specify the Topic: Let Lyzr know the specific topic you want to focus on within the chapter.
Generate Summary: Click the "Submit" button and watch Lyzr work its magic!
The app will display a bulleted summary that highlights the key points of the chosen topic.
Setting Up the Environment
Imports:
pip install streamlit lyzr openaiimport streamlit as stimport osfrom PIL import Imagefrom utils import utilsfrom lyzr import Summarizerfrom dotenv import load_dotenvfrom lyzr_qa import question_generation
streamlit as st: Imports the Streamlit library for building the web app.
os: Used for interacting with the operating system (creating directories).
from PIL import Image: Imports the Image class from Pillow library for handling images.
from utils import utils: Imports functions from a custom utils.py file (likely containing helper functions for Streamlit).
from lyzr import Summarizer: Imports the Summarizer class from the lyzr library for text summarization.
from dotenv import load_dotenv: Imports the load_dotenv function to load environment variables from a.env file (likely containing the OpenAI API key).
Loading API Key:
load_dotenv()api = os.getenv("OPENAI_API_KEY")
load_dotenv(): Loads environment variables from the.env file.
api = os.getenv("OPENAI_API_KEY"): Retrieves the API key from the environment variable named "OPENAI_API_KEY".
Initializing Summarizer:
summarizer = Summarizer(api_key=api)
summarizer = Summarizer(api_key=api): Creates a Summarizer object using the retrieved API key.
Data Directory Setup:
data = "data"os.makedirs(data, exist_ok=True)
data = "data": Defines a variable data containing the directory name for storing uploaded files.
os.makedirs(data, exist_ok=True): Creates the data directory if it doesn't exist, ignoring errors if it already exists.

Simplify Your System Design Process with Lyzr Automata,OpenAI and Streamlit | by Harshit | Apr, 2024 | Medium
Harshit
Medium
rag_response Function:
def rag_response(topic, path): agent = question_generation(path) metric = f""" You are an expert of this {topic}. Tell me everything you know about this {topic}, Provide a detailed reponse on this {topic} from the given file""" response = agent.query(metric) return response.response
agent = question_generation(path): This part likely utilizes an external library (not imported here) to create a question generation agent based on the provided path (probably the document).
response = agent.query(metric): Queries the agent using the defined message.
return response.response: Returns the agent's response about the topic.
gpt_summary Function:
def gpt_summary(response): style = "Summary with bullet points and subheading font is bold" summary = summarizer.summarize(response, style=style) return summary
Defines the desired style for the summary (bullet points and bold subheadings).
summary = summarizer.summarize(response, style=style): Uses the Summarizer object to create a summary of the response text with the specified style.
return summary: Returns the generated summary.
default() Function(Displays options for using a default file):
def default(): st.info('Default file: Haloalkanes and Haloarenes') st.markdown(""" ##### Topics can be: 1. Haloalkanes 2. Haloarenes """) path = './lech201.pdf' user_topic = st.text_input('Enter the topic according to subject') if user_topic is not None: if st.button('Submit'): rag_generated_response = rag_response(topic=user_topic, path=path) # getting reponse from rag about the subject/topic gpt_response = gpt_summary(response=rag_generated_response) # create n number of question on rag response st.subheader('Summary') st.write(gpt_response)
st.info('Default file: Haloalkanes and Haloarenes'): Displays informational text about the default file.
Lists possible topics (Haloalkanes and Haloarenes).
path = './lech201.pdf': Defines the path to the default PDF file.
user_topic = st.text_input('Enter the topic according to subject'): Creates a text input field for the user to enter a specific topic.
Conditional statement to handle user input and button click:
If user_topic is not None (user entered a topic):
If the 'Submit' button is clicked:
Calls rag_response to get a response about the user-entered topic from the default file.
Calls gpt_summary to summarize the response.
Displays the generated summary under a "Summary" subheader.
upload() (Handles uploading a PDF file):
def upload(): file = st.file_uploader("Upload a Subject Book Pdf", type=["pdf"]) if file: utils.save_uploaded_file(file, directory_name=data) path = utils.get_files_in_directory(directory=data) filepath = path[0] # get the first filepath user_topic = st.text_input('Enter the topic according to subject') if user_topic is not None: if st.button('Submit'): rag_generated_response = rag_response(topic=user_topic, path=filepath) # getting reponse from rag about the subject/topic gpt_response = gpt_summary(response=rag_generated_response) # create n number of question on rag response st.subheader('Summary') st.write(gpt_response) else: st.warning('Please Upload subject pdf file!!!')
file = st.file_uploader("Upload a Subject Book Pdf", type=["pdf"]): Creates a file uploader for the user to upload a PDF file.
Conditional statement to handle uploaded file:
If file is not None (user uploaded a file):
Saves the uploaded file using a function from utils.py (likely save_uploaded_file).
main() Function:
def main(): image = Image.open("./logo/lyzr-logo.png") st.sidebar.image(image, width=150) st.sidebar.subheader('Lyzr Chapter Notes Generator') # session state for default button if 'default_button' not in st.session_state: st.session_state.default_button = False # session state for upload button if 'upload_button' not in st.session_state: st.session_state.upload_button = False def default_button(): st.session_state.default_button = True st.session_state.upload_button = False def upload_button(): st.session_state.upload_button = True st.session_state.default_button = False st.sidebar.button('Default File', on_click=default_button) st.sidebar.button('Upload File', on_click=upload_button) if st.session_state.default_button: default() if st.session_state.upload_button: upload()if __name__ == "__main__": utils.style_app() main() utils.template_end()
This section of the code manages the user interface elements for selecting between using a default file or uploading a file. It uses Streamlit's session state to track the button clicks and conditionally call the default or upload function based on the user's choice. The default and upload functions are likely defined elsewhere in your code and handle the specific processing for those scenarios.
The Lyzr Chapter Notes Generator demonstrates the power of Lyzr in simplifying complex tasks like summarizing academic materials. By leveraging Lyzr,Streamlit and OpenAI's GPT-3, we've created an intuitive and efficient tool for students and educators alike.
try it now: https://lyzr-chapter-notes.streamlit.app/For more information explore the website: Lyzr
Original Link: https://dev.to/harshitlyzr/generate-chapter-notes-with-lyzr-chatagentsummarizeropenai-and-streamlit-591f

Dev To
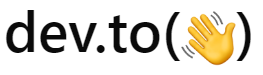
More About this Source Visit Dev To