An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Creating Your First Array in JavaScript: A Beginner's Guide.
What is an Array?
Arrays are fundamental data structures in JavaScript that allow you to store multiple values in a single variable. Whether you're storing a list of names, a collection of numbers, or a set of boolean values, arrays provide a convenient way to organize and manage data in your JavaScript code.
Basic Syntax of Array Creation:
To create an array in JavaScript, you use square brackets [ ]. Here's an example of how to create a simple array:
// Creating an array of numberslet numbers = [1, 2, 3, 4, 5];// Creating an array of stringslet fruits = ["apple", "banana", "orange"];// Creating an array of mixed data typeslet mixedArray = [1, "hello", true];
Initializing Arrays with Values:
You can initialize an array with values at the time of creation by specifying the values inside the square brackets. Here's how you can initialize arrays with values:
// Initializing an array with valueslet weekdays = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday"];// Initializing an array of numberslet primes = [2, 3, 5, 7, 11, 13];
Accessing Array Elements:
You can access individual elements of an array using square bracket notation ([]) along with the index of the element you want to access. Remember, array indices start from 0. Here's how you can access array elements:
let colors = ["red", "green", "blue"];// Accessing the first element (index 0)console.log(colors[0]); // Output: "red"// Accessing the second element (index 1)console.log(colors[1]); // Output: "green"// Accessing the third element (index 2)console.log(colors[2]); // Output: "blue"
Array Length:
The length property of an array allows you to determine the number of elements in the array.
let numbers = [1, 2, 3];// Getting the length of the arrayconsole.log(numbers.length); // Output: 3
Please leave a comment with the topic you'd like to learn more about regarding arrays.
Here are some suggested topics;
- - Array manipulations/Methods
- - Mutating vs Non-mutating array methods
- - Iterative array methods
- - Sorting and searching arrays
- - Error handling with array methods
Original Link: https://dev.to/ticha/creating-your-first-array-in-javascript-a-beginners-guide-k77

Dev To
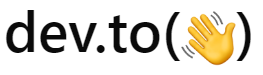
More About this Source Visit Dev To