An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Concurrent Dictionary: Your Go-To Guide
Are you tired of dealing with pesky synchronization issues when working with dictionaries in your .NET applications? Enter ConcurrentDictionary, your new best friend in the world of multithreading and parallel programming! Let's dive into what ConcurrentDictionary is all about, why it's awesome, and when to use (or not use) it.
What is ConcurrentDictionary?
ConcurrentDictionary is a class introduced in the .NET Framework 4.0. It provides a thread-safe way to store key-value pairs, allowing multiple threads to read, write, and update the dictionary concurrently without causing data corruption or synchronization problems. This makes it an invaluable tool for parallel programming scenarios.
Advantages of ConcurrentDictionary over Normal Dictionary
Thread Safety:
Unlike the traditional Dictionary class, ConcurrentDictionary handles concurrent read and write operations gracefully. You don't need to implement your own locking mechanisms or worry about race conditions.
Performance:
ConcurrentDictionary is optimized for concurrent access, providing efficient operations even under heavy multi-threaded workloads. It uses fine-grained locking and internally segmented data structures to minimize contention and maximize throughput.
Scalability:
As your application scales with more threads accessing the dictionary simultaneously, ConcurrentDictionary scales with it. It offers better performance compared to locking the entire dictionary, especially in scenarios with high contention.
Where to Use ConcurrentDictionary
Multithreaded Environments: Whenever you're working with multiple threads that need to access or modify a shared dictionary, ConcurrentDictionary is your go-to choice. It ensures data integrity without sacrificing performance.
Parallel Algorithms:
When implementing parallel algorithms such as map-reduce or parallel processing of data, ConcurrentDictionary shines. It allows you to efficiently aggregate results from multiple threads without worrying about synchronization headaches.
Highly Concurrent Systems:
In scenarios where you anticipate heavy concurrent access to your dictionary, such as web servers handling multiple requests simultaneously, ConcurrentDictionary provides a robust solution.
Where to Avoid ConcurrentDictionary
Single-Threaded Applications:
If your application runs entirely on a single thread or doesn't require concurrent access to the dictionary, using ConcurrentDictionary might introduce unnecessary overhead. Stick with the traditional Dictionary class in such cases.
Performance-Critical Low-Contention Scenarios:
In scenarios where you have low contention and performance is critical, the overhead of ConcurrentDictionary's thread-safe mechanisms might outweigh its benefits. Profile your code and consider alternatives if needed.
Use Cases of ConcurrentDictionary
Let's take a look at a simple example to demonstrate the usage of ConcurrentDictionary:
using System;using System.Collections.Concurrent;using System.Threading.Tasks;class Program{ static void Main() { var concurrentDict = new ConcurrentDictionary<int, string>(); // Adding elements concurrently Parallel.For(0, 10, i => { concurrentDict.TryAdd(i, $"Value {i}"); }); // Retrieving elements concurrently Parallel.For(0, 10, i => { if (concurrentDict.TryGetValue(i, out string value)) { Console.WriteLine($"Key: {i}, Value: {value}"); } }); }}
In this example, we create a ConcurrentDictionary
and add elements to it concurrently using Parallel.For
. Then, we retrieve the elements concurrently using another Parallel.For
loop. Thanks to ConcurrentDictionary's thread-safe nature, we don't need to worry about synchronization issues, and the output will be correct and consistent.
Wrapping Up
ConcurrentDictionary is a powerful tool in your arsenal for building scalable and robust multi-threaded applications in .NET. By providing thread-safe access to key-value pairs, it eliminates the headache of manual synchronization and ensures your application's integrity and performance under concurrent workloads. Just remember to use it wisely in appropriate scenarios, and you'll be on your way to smoother parallel programming experiences. Happy coding!
Original Link: https://dev.to/yogini16/concurrent-dictionary-your-go-to-guide-20n3

Dev To
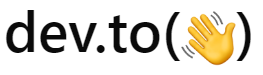
More About this Source Visit Dev To