An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
[623. Add One Row to Tree](https://leetcode.com/problems/add-one-row-to-tree/)
623. Add One Row to Tree
Solved
Medium
Topics
Companies
Given the root of a binary tree and two integers val and depth, add a row of nodes with value val at the given depth depth.
Note that the root node is at depth 1.
The adding rule is:
Given the integer depth, for each not null tree node cur at the depth depth - 1, create two tree nodes with value val as cur's left subtree root and right subtree root.
cur's original left subtree should be the left subtree of the new left subtree root.
cur's original right subtree should be the right subtree of the new right subtree root.
If depth == 1 that means there is no depth depth - 1 at all, then create a tree node with value val as the new root of the whole original tree, and the original tree is the new root's left subtree.
Example 1:
Input: root = [4,2,6,3,1,5], val = 1, depth = 2Output: [4,1,1,2,null,null,6,3,1,5]
Example 2:
Input: root = [4,2,null,3,1], val = 1, depth = 3Output: [4,2,null,1,1,3,null,null,1]
Constraints:
The number of nodes in the tree is in the range [1, 104].
The depth of the tree is in the range [1, 104].
-100 <= Node.val <= 100
-105 <= val <= 105
1 <= depth <= the depth of tree + 1
Seen this question in a real interview before?
1/5
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode() {} * TreeNode(int val) { this.val = val; } * TreeNode(int val, TreeNode left, TreeNode right) { * this.val = val; * this.left = left; * this.right = right; * } * } */class Solution { public TreeNode addOneRow(TreeNode root, int val, int depth) { return dfs(root,val,depth,1,false); } public TreeNode dfs(TreeNode root, int val, int depth, int curr,boolean isLeft){ if(root==null) { if(depth==1) root=new TreeNode(val); return root; } if(depth==1 && root!=null) { TreeNode newNode=new TreeNode(val); newNode.left=root; root=newNode; return newNode; } if(curr+1==depth){ TreeNode newNode=new TreeNode(val); newNode.left=root.left; root.left=newNode; TreeNode newNode1=new TreeNode(val); newNode1.right=root.right; root.right=newNode1; return root; } dfs(root.left,val,depth,curr+1,true); dfs(root.right,val,depth,curr+1,false); return root; } }/**623. Add One Row to TreeSolvedMediumTopicsCompaniesGiven the root of a binary tree and two integers val and depth, add a row of nodes with value val at the given depth depth.Note that the root node is at depth 1.The adding rule is:Given the integer depth, for each not null tree node cur at the depth depth - 1, create two tree nodes with value val as cur's left subtree root and right subtree root.cur's original left subtree should be the left subtree of the new left subtree root.cur's original right subtree should be the right subtree of the new right subtree root.If depth == 1 that means there is no depth depth - 1 at all, then create a tree node with value val as the new root of the whole original tree, and the original tree is the new root's left subtree.Example 1:Input: root = [4,2,6,3,1,5], val = 1, depth = 2Output: [4,1,1,2,null,null,6,3,1,5]Example 2:Input: root = [4,2,null,3,1], val = 1, depth = 3Output: [4,2,null,1,1,3,null,null,1]Constraints:The number of nodes in the tree is in the range [1, 104].The depth of the tree is in the range [1, 104].-100 <= Node.val <= 100-105 <= val <= 1051 <= depth <= the depth of tree + 1 */
Original Link: https://dev.to/mshashikanth1/623-add-one-row-to-treehttpsleetcodecomproblemsadd-one-row-to-tree-27og

Dev To
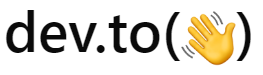
More About this Source Visit Dev To