An Interest In:
Web News this Week
- March 28, 2024
- March 27, 2024
- March 26, 2024
- March 25, 2024
- March 24, 2024
- March 23, 2024
- March 22, 2024
Unraveling the Mysteries of JavaScript, Tackling Tough Logic
Introduction:
JavaScript, with its flexibility and power, often presents developers with challenges that test their problem-solving skills to the limit. In this post, we'll dive deep into some of the toughest logic problems in JavaScript and unravel the solutions step by step. From tricky algorithms to mind-bending puzzles, get ready to sharpen your JavaScript skills and conquer the toughest of challenges.
Problem: Palindrome Check
One classic problem that can stump even seasoned developers is checking whether a given string is a palindrome or not. A palindrome is a word, phrase, number, or other sequences of characters that reads the same forward and backward. For example, "radar" and "madam" are palindromes.
Approach:
To solve this problem, we'll compare characters from the beginning and end of the string, moving towards the middle. If all characters match, the string is a palindrome.
function isPalindrome(str) {
// Remove non-alphanumeric characters and convert to lowercase
str = str.replace(/[^A-Za-z0-9]/g, '').toLowerCase();
// Compare characters from start and endfor (let i = 0; i < Math.floor(str.length / 2); i++) { if (str[i] !== str[str.length - 1 - i]) { return false; }}return true;
}
// Test cases
console.log(isPalindrome("radar")); // Output: true
console.log(isPalindrome("hello")); // Output: false
console.log(isPalindrome("A man, a plan, a canal, Panama")); // Output: true
Explanation:
- We first remove non-alphanumeric characters from the input string using a regular expression and convert the string to lowercase to handle case-insensitive comparisons.
- Then, we use a for loop to iterate through the characters of the string from the beginning towards the middle.
- Inside the loop, we compare each character with its corresponding character from the end of the string. If any pair of characters doesn't match, we return false, indicating that the string is not a palindrome.
- the loop completes without finding any mismatches, we return true, indicating that the string is indeed a palindrome.
Conclusion:
By understanding and mastering these tough logic problems in JavaScript, you'll not only enhance your problem-solving skills but also gain a deeper insight into the intricacies of the language. Keep exploring, keep learning, and keep pushing the boundaries of what you can achieve with JavaScript. Happy coding!
Original Link: https://dev.to/yugandhar_dasari_93/unraveling-the-mysteries-of-javascript-tackling-tough-logic-31e3

Dev To
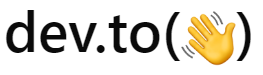
More About this Source Visit Dev To