An Interest In:
Web News this Week
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
- April 19, 2024
- April 18, 2024
PYTHON TURTLE: OVERVIEW
What is Python Turtle?
Python Turtle is a library used to create simple graphics in Python. It is a great way to introduce programming to kids and adults alike, as it is easy to use and understand. Python Turtle also allows you to learn the basics of programming without having any prior knowledge or experience with coding.
Getting Started with Python Turtle
To get started with Python Turtle, you need to download and install Python. Then you can install the Turtle library by following these steps:
Open a new Python file in your favorite text editor (I use Sublime Text)
Type import turtle at the top of your file.
Create a Turtle object by typing t = Turtle() in your code window, then pressing Enter or Return on your keyboard. This will create an instance of our turtle named t that we can use to draw shapes and patterns!
Using Turtle Commands
Turtle Motion Commands:
- forward(distance): moves the turtle forward by a specified distance
- backward(distance) : moves the turtle backward by a specified distance
- right(angle) : turns the turtle clockwise by a specified angle
- left(angle) : turns the turtle counterclockwise by a specified angle
- goto(x, y) : moves the turtle to the specified coordinates (x, y)
- setx(x) : moves the turtle to the specified x-coordinate
- sety(y) : moves the turtle to the specified y-coordinate
- setheading(angle) : sets the turtle's heading to the specified angle
- home() : moves the turtle to the origin (0, 0) and sets the turtle's heading to due east (0 degrees)
Pen Control Commands:
- pendown() : puts the pen down, allowing the turtle to draw
- pinupup() : lifts the pen up, preventing the turtle from drawing
- pensize(width) : sets the width of the pen to the specified value
- pencolor(color) : sets the color of the pen to the specified color
- fillcolor(color) : sets the color used for filling in shapes
- begin_fill() : starts filling in a shape
- end_fill() : stops filling in a shape
Window Control Commands:
- screensize(width, height) : sets the size of the turtle's screen to the specified values
- title(title) : sets the title of the turtle's window
- bgcolor(color) : sets the background color of the turtle's window
- window_width() : returns the width of the turtle's window
- window_height() : returns the height of the turtle's window
Other Commands:
- clear() : clears the turtle's screen and resets the turtle to its original state
- reset() : clears the turtle's screen and resets the turtle to its original state, but does not clear the drawing window
- hideturtle() : hides the turtle from view
- showturtle() : makes the turtle visible again
- speed(speed) : sets the speed of the turtle (0 = fastest, 10 = slowest)
- undo() : undoes the last action performed by the turtle
- isdown() : returns True if the pen is down, False otherwise.
Examples:
import turtleimport randomdef draw_star(size, color, x, y): turtle.penup() turtle.goto(x, y) turtle.pendown() turtle.color(color) turtle.begin_fill() for _ in range(5): turtle.forward(size) turtle.right(144) turtle.end_fill()def animate_stars(): turtle.clear() for _ in range(25): size = random.randint(10, 30) x = random.randint(-300, 300) y = random.randint(-300, 300) color = random.choice(["red", "blue", "green", "yellow", "white", "cyan", "magenta"]) draw_star(size, color, x, y) turtle.ontimer(animate_stars, 1000)turtle. Speed(0)turtle.bgcolor("black")turtle.hideturtle()turtle.ontimer(animate_stars, 1000)turtle.mainloop()
[Youtube]{https://www.youtube.com/watch?v=7dw-MYvF0}
Conclusion
Python Turtle is a great way to introduce programming to kids and adults alike. Its easy to use, understand and a great way to learn the basics of programming.
Original Link: https://dev.to/devloperstoday/python-turtle-overview-2ha2

Dev To
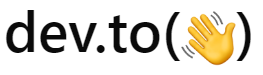
More About this Source Visit Dev To