An Interest In:
Web News this Week
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
- April 9, 2024
Building Pagination in React with React Paginate
Introduction
Pagination is a crucial component in any web application, as it allows for the efficient and seamless navigation of large amounts of data. In React, implementing pagination can be a challenge for developers, especially for those who are new to the framework. However, with the right understanding of React components and hooks, building pagination in React can be a straightforward process.
In this article, we will explore the steps involved in building a pagination system in React, from setting up the component structure to integrating it with your application's data. This guide will provide a comprehensive overview of the process and provide you with the knowledge and skills you need to build an effective and user-friendly pagination system.
We are going to look into the following topics in the article:
- Installing Component
- Understanding the
react-paginate
library with props - Data filtering for different page
- Pagination
- Conclusion
Now, let's get started.
Installing Library
We are going to use the react-paginate library to build our pagination features. You can install the library with the below command:
npm i react-paginate
Along with that, we will need some icons. For icons, I am using the react-icons library. Install it with the below command:
npm install react-icons --save
App.js
We are building an application that is going to have data in an array. We need to display only 3 data on each page(We can change as per requirement).
Let's start building them one by one.
Imports
Here are the imports that we will require for the component.
import ReactPaginate from "react-paginate"; // for paginationimport { AiFillLeftCircle, AiFillRightCircle } from "react-icons/ai"; // icons form react-iconsimport { IconContext } from "react-icons"; // for customizing iconsimport { useEffect, useState } from "react"; // useState for storing data and useEffect for changing data on click import "./styles.css"; // stylesheet
You can look for the comments for each import.
Data
The data is simple, it's the letter of the alphabet in an array.
const data = [ "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z" ];
useEffect and useState
Here are the states of our application.
const [page, setPage] = useState(0);const [filterData, setFilterData] = useState();const n = 3
page: It is for storing the current page number for the pagination component
filterData: It is the data that will be shown after filtering data
for each page.
n: It is the maximum number of items to show on a page.
Here is the code for the useEffect
hook that we are using to filter data. The data will filter when the user change to a different page. On each page, there will be filtered data for displaying.
useEffect(() => { setFilterData( data.filter((item, index) => { return (index >= page * n) & (index < (page + 1) * n); }) );}, [page]);
The return formula will return the item from the data
array in an array with an index in the multiple of 3.
Return
In the return statement, at the top, we are displaying the filterData
.
<ul>{filterData && filterData.map((item, index) => <li>Item #{item}</li>)}</ul>;
Now, let's look into the ReactPaginate
component. First, let's look at the code then I will explain each prop.
<ReactPaginate containerClassName={"pagination"} pageClassName={"page-item"} activeClassName={"active"} onPageChange={(event) => setPage(event.selected)} pageCount={Math.ceil(data.length / n)} breakLabel="..." previousLabel={ <IconContext.Provider value={{ color: "#B8C1CC", size: "36px" }}> <AiFillLeftCircle /> </IconContext.Provider> } nextLabel={ <IconContext.Provider value={{ color: "#B8C1CC", size: "36px" }}> <AiFillRightCircle /> </IconContext.Provider> }/>;
Props | Description |
---|---|
containerClassName | You can provide a class name for the container of the pagination component. |
pageClassName | It is for the class name for each page number. |
activeClassName | Classname of the active page. |
onPageChange | It is the function that will be triggered on changing page. In this, we are storing the page number in the page state. Also, it starts with 0 and the number in the component starts with 1. |
pageCount | It is a required prop. As the name suggests, it will display the number of pages. I have divided the length of the data by the number of each item on a page. |
breakLabel | The component will be displayed when there will be a break between pages. It is to show that there is more page in between. |
previousLabel | The icon component is used here for displaying the previous button. |
nextLable | Here it is for the next button. |
CSS
Here is the CSS of each component used in the code.
.App { display: flex; font-family: sans-serif; margin: 0 auto; align-items: center; justify-content: center; flex-direction: column;}.pagination { list-style: none; height: 31.5px; width: 31.5px; display: flex; justify-content: center; align-items: center; margin-top: 2px; cursor: pointer;}.active { background-color: #1e50ff; border-radius: 50%;}.page-item { list-style: none; padding: 2px 12px; height: 31.5px; width: 31.5px; display: flex; justify-content: center; align-items: center; margin-top: 2px;}
CodeSandbox
You can look into the complete code and the output of the project in the below codesandbox.
Conclusion
Building pagination in React is an important aspect of web development and can greatly enhance the user experience in navigating large amounts of data. By following the steps outlined in this article, you can easily implement pagination in your React application and provide your users with a smooth and efficient way to access the data they need.
I hope the articles have helped you in understanding the process of creating a pagination component in React. Thanks for reading the article.
Original Link: https://dev.to/documatic/building-pagination-in-react-with-react-paginate-4nol

Dev To
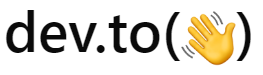
More About this Source Visit Dev To