An Interest In:
Web News this Week
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
- April 9, 2024
Build Your First API with Node.js: A Step-by-Step Guide
Hi, I'm Subham Maity, a software engineer. I also enjoy teaching others how to code through my tutorials. I'm always eager to learn new things and share my knowledge with the community.
I recently wrote an article on Build Your First API and wanted to share it with you all. You can find the article on my website https://codexam.vercel.app/docs/node/node1 [Better View]
I also have a repository on GitHub where you can find all the code and projects related to this topic. You can find the repository at https://github.com/Subham-Maity/node-js-full-stack-tutorial
For a more in-depth look at this topic, including a detailed table of contents, check out the complete tutorial on my GitHub Repo
If you want to stay updated with my latest projects and articles, you can follow me on:
- We already know how to make a simple server. You can check it out the previous chapter
const http = require('http'); http.createServer((req, res) => { } ).listen(5000);
- Now
res.writeHead(200, {'Content-Type': 'application/json'});
- This line sets the response status code and headers using thewriteHead
method of theres
(response) object
writeHead
method takes two arguments, the first is the status code and the second is an object containing the response headers (in this case, we are setting theContent-Type
header toapplication/json
)The
writeHead
method is used to send the response status code and headers to the clientThe status code
200
is a standard HTTP status code that indicates the request was successful.The
Content-Type
header is used to specify the media type of the resource being sent in the response body this means that the client can expect to receive data in a specific format.
application/json
, indicating that the response body will contain data in JSON format & we use/
to separate the type and subtype of the media type.
- Now
res.write(JSON.stringify({name: 'Subham', age: 20}));
- This line writes the response body using thewrite
method of theres
(response) object
The
write
method is used to send the response body to the client means that the client can expect to receive data in a specific format.The
JSON.stringify
method is used to convert a JavaScript object to a JSON stringIn this case, it is sending a JSON representation of an object with properties
name
andage
.The
JSON.stringify
method takes a single argument, the object to be converted to a JSON string
- Now
res.end();
- This line signals the end of the response and sends it to the client using theend
method of theres
(response) object.
- The
end
method is used to indicate that all of the response headers and body have been sent and that the server should consider this message complete.These lines of code are part of a Node.js server that sends a response to an HTTP request. The
writeHead
method is used to set the response status code and headers. In this case, the status code is set to200
to indicate success, and theContent-Type
header is set toapplication/json
to indicate that the response will contain JSON data. Thewrite
method is used to send data in the response body. In this case, it sends a JSON representation of an object with propertiesname
andage
. Finally, theend
method is used to signal the end of the response and send it to the client.
const http = require('http');http.createServer((req, res) => { res.writeHead(200, {'Content-Type': 'application/json'}); res.write(JSON.stringify({name: 'Subham', age: 20})); res.end(); }).listen(5000);
Now we can run this server using
nodemon server.js
and check it out in the browser usinglocalhost:5000
Now we can see the output in the browser like this
{ "name": "Subham", "age": 20}
You can also check it out in the postman we already discussed about it in this project section here
Separate the data using data.js
- Create a new file
data.js
and add this code
const data ={ "name":"Rajesh", "age": 20, "email": "[email protected]" } module.exports=data;
- Here
const data
is a variable which contains an object with some dataname
is a property of the object and its value isRajesh
age
is a property of the object and its value is20
email
is a property of the object and its value is `
module.exports=data
is used to export the data variable so that it can be used in other files
- Now we can import this data in the
server.js
file usingrequire
method
const data = require('./data');
- Now pass the data variable in the
write
method of theres
object insideJSON.stringify
method like this
res.write(JSON.stringify(data));
Now we can run this server using
nodemon server.js
and check it out in the browser usinglocalhost:5000
Now we can see the output in the browser like this
{"name":"Rajesh","age":20,"email":"[email protected]"}
Add more data in the data.js file
- Now we can add more data in the
data.js
file like this
`js
const data = [
{
name: "Rajesh",
age: 20,
address: {
city: "Delhi",
state: "Delhi",
country: "India",
},
hobbies: ["coding", "reading", "playing"],
skills: ["html", "css", "js", "nodejs"],
education: {
school: "Delhi Public School",
college: "Delhi University",
degree: "B.Tech",
},
projects: {
project1: "Portfolio",
project2: "Blog",
project3: "E-commerce",
},
social: {
github: "rajesh.github.io",
linkedin: "rajesh.linkedin.com",
twitter: "rajesh.twitter.com",
},
work: {
company: "XYZ",
position: "Software Engineer",
experience: "2 years",
},
achievements: {
achievement1: "Won a hackathon",
achievement2: "Got a scholarship",
achievement3: "Got a job",
},
interests: {
interest1: "Reading",
interest2: "Playing",
interest3: "Coding",
},
languages: {
language1: "English",
language2: "Hindi",
language3: "Punjabi",
},
contact: {
phone: "1234567890",
email: "rajesh.dev.com",
},
},
{
name: "Subham",
age: 20,
address: {
city: "Delhi",
state: "Delhi",
country: "India",
},
hobbies: ["coding", "reading", "playing"],
skills: ["html", "css", "js", "nodejs"],
education: {
school: "Delhi Public School",
college: "Delhi University",
degree: "B.Tech",
},
projects: {
project1: "Portfolio",
project2: "Blog",
project3: "E-commerce",
},
social: {
github: "subham.github.io",
linkedin: "subham.linkedin.com",
twitter: "subham.twitter.com",
},
work: {
company: "XYZ",
position: "Software Engineer",
experience: "2 years",
},
achievements: {
achievement1: "Won a hackathon",
achievement2: "Got a scholarship",
achievement3: "Got a job",
},
interests: {
interest1: "Reading",
interest2: "Playing",
interest3: "Coding",
},
languages: {
language1: "English",
language2: "Hindi",
language3: "Punjabi",
},
contact: {
phone: "1234567890",
email: "subham.dev.com",
},
},
{
name: "Rahul",
age: 20,
address: {
city: "Delhi",
state: "Delhi",
country: "India",
},
hobbies: ["coding", "reading", "playing"],
skills: ["html", "css", "js", "nodejs"],
education: {
school: "Delhi Public School",
college: "Delhi University",
degree: "B.Tech",
},
projects: {
project1: "Portfolio",
project2: "Blog",
project3: "E-commerce",
},
social: {
github: "rahul.github.io",
linkedin: "rahul.linkedin.com",
twitter: "rahul.twitter.com",
},
work: {
company: "XYZ",
position: "Software Engineer",
experience: "2 years",
},
achievements: {
achievement1: "Won a hackathon",
achievement2: "Got a scholarship",
achievement3: "Got a job",
},
interests: {
interest1: "Reading",
interest2: "Playing",
interest3: "Coding",
},
languages: {
language1: "English",
language2: "Hindi",
language3: "Punjabi",
},
contact: {
phone: "1234567890",
email: "rahul.dev.com",
},
},
];
module.exports = data;
`
- Now our server will look like this
`js
const http = require('http');
const data = require('./data');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'application/json'});
res.write(JSON.stringify(data));
res.end();
}
).listen(5000);
`
Now we can run this server using
nodemon server.js
and check it out in the browser usinglocalhost:5000
Now we can see the output in the browser like this
[{"name":"Rajesh","age":20,"address":{"city":"Delhi","state":"Delhi","country":"India"},"hobbies":["coding","reading","playing"],"skills":["html","css","js","nodejs"],"education":{"school":"Delhi Public School","college":"Delhi University","degree":"B.Tech"},"projects":{"project1":"Portfolio","project2":"Blog","project3":"E-commerce"},"social":{"github":"rajesh.github.io","linkedin":"rajesh.linkedin.com","twitter":"rajesh.twitter.com"},"work":{"company":"XYZ","position":"Software Engineer","experience":"2 years"},"achievements":{"achievement1":"Won a hackathon","achievement2":"Got a scholarship","achievement3":"Got a job"},"interests":{"interest1":"Reading","interest2":"Playing","interest3":"Coding"},"languages":{"language1":"English","language2":"Hindi","language3":"Punjabi"},"contact":{"phone":"1234567890","email":"rajesh.dev.com"}},{"name":"Subham","age":20,"address":{"city":"Delhi","state":"Delhi","country":"India"},"hobbies":["coding","reading","playing"],"skills":["html","css","js","nodejs"],"education":{"school":"Delhi Public School","college":"Delhi University","degree":"B.Tech"},"projects":{"project1":"Portfolio","project2":"Blog","project3":"E-commerce"},"social":{"github":"subham.github.io","linkedin":"subham.linkedin.com","twitter":"subham.twitter.com"},"work":{"company":"XYZ","position":"Software Engineer","experience":"2 years"},"achievements":{"achievement1":"Won a hackathon","achievement2":"Got a scholarship","achievement3":"Got a job"},"interests":{"interest1":"Reading","interest2":"Playing","interest3":"Coding"},"languages":{"language1":"English","language2":"Hindi","language3":"Punjabi"},"contact":{"phone":"1234567890","email":"subham.dev.com"}},{"name":"Rahul","age":20,"address":{"city":"Delhi","state":"Delhi","country":"India"},"hobbies":["coding","reading","playing"],"skills":["html","css","js","nodejs"],"education":{"school":"Delhi Public School","college":"Delhi University","degree":"B.Tech"},"projects":{"project1":"Portfolio","project2":"Blog","project3":"E-commerce"},"social":{"github":"rahul.github.io","linkedin":"rahul.linkedin.com","twitter":"rahul.twitter.com"},"work":{"company":"XYZ","position":"Software Engineer","experience":"2 years"},"achievements":{"achievement1":"Won a hackathon","achievement2":"Got a scholarship","achievement3":"Got a job"},"interests":{"interest1":"Reading","interest2":"Playing","interest3":"Coding"},"languages":{"language1":"English","language2":"Hindi","language3":"Punjabi"},"contact":{"phone":"1234567890","email":"rahul.dev.com"}}]
Status Code
If I send instead of 200, I send 404, it will show not found in the postman status code because there are lots of status codes are there. We use them according to our need.
Some of the status codes are:
- 200 - OK
- 201 - Created
- 204 - No Content
- 400 - Bad Request
- 401 - Unauthorized
- 403 - Forbidden
- 404 - Not Found
- 500 - Internal Server Error
- 503 - Service Unavailable
You can check all the status codes here
Original Link: https://dev.to/codexam/build-your-first-api-with-nodejs-a-step-by-step-guide-2ogo

Dev To
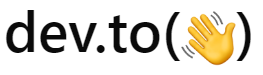
More About this Source Visit Dev To