An Interest In:
Web News this Week
- April 29, 2024
- April 28, 2024
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
How to make a modal with ReactJs
Modals come in handy when you want to notify the user of an important action that was performed successfully or not or when your quckly want to pass an information to your user as soon as they open your app. For example in an ecommerce app, when the user has hitted the complete order or checkout button, you may want to confirm the user's intention to proceed with the order by simply showing a modal requiring the user to either click the continue or cancel button. After completing the order, it is a common practice to also display a form of notification box to inform the user that the order was successfully placed.
There are other many use cases of modal, in fact there are cases that a login form in a modal is pop out for the user to login with.
In this tutorial, we will be making a modal with React useState
and css
.
The main purpose of this tutorial is to demonstrate to the reader how a modal can be made using the techniques stated above.
Understanding of the content of this article will enable the reader to be able to deploy the techniques in any way that suits his/her need.
In case you are starting from scratch, run any of the commands below to create react app or any equivalent command depending on your device and tool your are using.
Run the command in the folder you want to create the app.
yarn create react-app name_of_your_app
npx create-react-app name_of_your_app
In the src folder of the created app, I will a create folder called components. Then inside this folder I will make a
file and name it ModalPage.js
. This file will contain two React components as follows
The first component is the Modal
. This component will accept two props as defined below:
isModalOpen
: This is a react state that signifies if the modal is opened or not. It is of Boolean type that is, it is eithertrue
orfalse
. When it istrue
, it implies the modal is opened while beingfalse
implies the modal is closed.closeModal
: This is a function to close the modal. Hence this function will set theisModalOpen
state value tofalse
.
const Modal = ({isModalOpen, closeModal}) => { return ( <div className={`${isModalOpen? 'modal open' : 'modal'}`}> <div className='modal-content'> <p>Modal opened click the close button below to close modal</p> <button className='btn close-modal' onClick={closeModal}>close modal</button> </div> </div> )}
Note, that the className of the Modal
component is conditionally set to be either modal
or modal open
depending on the value of isModalOpen
using a ternary operator. When the value of isModalOpen
is false
the className of the Modal
component will be modal
while when its value is true
the className
of the Modal
component will be modal open
indicating the Modal
is opened as explained above.
Also, there is a button inside the Modal
which when clicked sets the the value of isModalOpen
to false
thereby closing the Modal
. This is achieved by attaching an nnClick
event listener to button such as when it is clicked, the function closeModal
is called and hence the value of the isModalOpen
is set to false
thereby closing the Modal
.
The second component is the ModalPage
described below.
In this component we define a state variable isModalOpen
and corresponding function, setIsModalOpen
to toggle its value using the React useState
hook. isModalOpen
is passed as prop to the Modal
component.
Two functions are defined:
closeModal
: This function is used to set the value ofisModalOpen
tofalse
, thereby closing theModal
. This function is passed as prop value to theModal
component.openModal
: This function is used to set the value ofisModalOpen
totrue
thereby opening theModal
. The function is attached to a button in this component throughonClick
event. Hence, whenever the button is clicked, the value ofisModalOpen
is set totrue
and thereby opening theModal
const ModalPage = () => { const [isModalOpen, setIsModalOpen] = useState(false) const closeModal = () => { setIsModalOpen(false) } const openModal = () => { setIsModalOpen(true) } return ( <> <Modal closeModal={closeModal} isModalOpen={isModalOpen} /> <main> <section className='page-element'> <h1>Page content</h1> <button className='btn open-modal' onClick={openModal}>open modal</button> </section> </main> </> )}export default ModalPage
Note: In case your Modal
and ModalPage
components are not in the same file, ensure you import the Modal
component into the file containing the ModalPage
component. Also, you can name your components whatever you like as long as they conform to React components naming convention.
Then write the following css inside your index.css located in the source folder of your react app.
.btn { cursor: pointer; border: none; padding: .5rem;}.modal { position: fixed; top: 0; left: 0; min-height: 100vh; width: 100vw; display: none;}.modal.open { display: grid; place-items: center; background-color: rgba(0, 0, 0, .5);}.modal-content { display: flex; flex-direction: column; width: 90%; max-width: 300px; padding: 1rem; background-color: #ffffff;}.close-modal { background-color: #d11a2a; color: #ffffff;}
And in the App.js
located in src
folder of the created app, import the ModalPage
component.
import ModalPage from './components/ModalPage';function App() { return ( <div> <ModalPage /> </div> );}export default App;
Now, let's relate our css
with our Modal
component. Note the Modal
can have its class to be either modal
when the value of isModalOpen
is false
or modal open
when the value of isModalOpen
is true. This is where the logic lies. In the css
file, element with class modal
is given display
property of none
, that is not to display while element with class modal open
is given display
property of grid
. Hence as the value of isModalOpen
toggles between false
and true
, the Modal
is removed and added back to the DOM
appropriately.
Then render your App in the index.js
located in the src
folder of the created app
import React from 'react';import ReactDOM from 'react-dom/client';import './index.css';import App from './App';import reportWebVitals from './reportWebVitals';const root = ReactDOM.createRoot(document.getElementById('root'));root.render( <React.StrictMode> <App /> </React.StrictMode>);
Note, there are things you can change here, such as the styling of your modal content, where your declare the variables and function used and also the background color of the Modal
. The most important information is understanding the technique used here.
Yes that is it with creating modal in ReactJS. Kindly put your questions or observations in the comment box below, I will be really grateful if I can use your insights to improve my work.
If you love my work and want to support me, you can buy me a coffee here. No amount is small. Thank you and watch out for my coming articles
Original Link: https://dev.to/ismailadegbite/how-to-make-a-modal-with-reactjs-4n69

Dev To
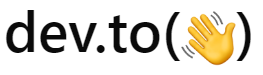
More About this Source Visit Dev To