An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
Understanding and Using GraphQL in React: A Beginner's Guide
Understanding and using GraphQL in a React application can seem like a daunting task, especially for beginners. However, with the right tools and knowledge, it can be a powerful way to handle data in your application. In this blog post, we'll explore what GraphQL is, how it works and how to use it in a React application.
First, let's start by understanding what GraphQL is. GraphQL is a query language for APIs that allows you to request exactly the data you need, and nothing more. Unlike REST, where you have to make multiple requests to different endpoints to get all the data you need, with GraphQL you can make a single request and get all the data in one place.
An example of how GraphQL works is by making a query to a GraphQL server. Let's say you have a server that has data about movies and you want to get the title and release date of a specific movie. With GraphQL, you can make a query like this:
query { movie(id: 1) { title releaseDate }}
This query is asking for the title and release date of the movie with an id of 1. The server will then respond with the data in the following format:
{ "data": { "movie": { "title": "The Matrix", "releaseDate": "1999-03-31" } }}
Now that we understand what GraphQL is, let's take a look at how to use it in a React application. One popular library for working with GraphQL in a React application is Apollo Client. Apollo Client is a community-driven library that allows you to easily work with GraphQL in your application. It provides a set of hooks and components that make it easy to fetch data, handle errors and update the UI.
An example of how to use Apollo Client in a React application is by installing the package and setting up the client. First, you'll need to install the necessary packages:
npm install apollo-client apollo-cache-inmemory apollo-link-http
Once the packages are installed, you'll need to set up the client by creating an instance of ApolloClient and passing in the necessary configurations.
import { ApolloClient, InMemoryCache } from 'apollo-client-preset'import { HttpLink } from 'apollo-link-http'const client = new ApolloClient({ cache: new InMemoryCache(), link: new HttpLink({ uri: 'https://your-graphql-server.com/graphql' })});
Now that the client is set up, you can use it in your components by using the useQuery
hook to fetch data and the useMutation
hook to make changes to the data.
An example of how to use the useQuery
hook is by making a query to the server and updating the UI with the data:
import { useQuery } from '@apollo/react-hooks';import gql from 'graphql-tag';const GET_MOVIES = gql` query { movies { title releaseDate } }`;function MovieList() { const { loading, error, data } = useQuery(GET_MOVIES); if (loading) return 'Loading...'; if (error) return `Error! ${error.message}`; return ( <div> {data.movies.map(movie => ( <div key={movie.id}> <h2>{movie.title}</h2> <p>{movie.releaseDate}</p> </div> ))} </div> );}
In this example, we are using the useQuery
hook to fetch data from the server and displaying it in the UI. The loading
state is used to show a loading message while the data is being fetched, the error
state is used to show an error message if there is an issue with the query and the data
state is used to display the data once it's successfully fetched.
Similarly, you can use the useMutation
hook to make changes to the data by sending a mutation to the server. An example of how to use the useMutation
hook is by creating a new movie:
import { useMutation } from '@apollo/react-hooks';import gql from 'graphql-tag';const CREATE_MOVIE = gql` mutation createMovie($title: String!, $releaseDate: String!) { createMovie(title: $title, releaseDate: $releaseDate) { id title releaseDate } }`;function CreateMovie() { const [createMovie, { data }] = useMutation(CREATE_MOVIE); return ( <form onSubmit={e => { e.preventDefault(); createMovie({ variables: { title, releaseDate } }); }} > <input type="text" placeholder="Title" ref={title} /> <input type="text" placeholder="Release Date" ref={releaseDate} /> <button type="submit">Create</button> </form> );}
In this example, we are using the useMutation
hook to send a mutation to the server to create a new movie. We pass in the variables of title and release date and then update the UI with the new data once the mutation is successful.
To conclude, understanding and using GraphQL in a React application can seem overwhelming, but with the right tools and knowledge, it can be a powerful way to handle data in your application. Apollo Client is a popular library that makes it easy to work with GraphQL in your React application. By using the useQuery
and useMutation
hooks, you can easily fetch data and make changes to it. Remember that GraphQL allows you to request exactly the data you need and nothing more, making it more efficient and flexible than traditional REST APIs.
Additionally, it's important to note that GraphQL also provides a built-in way to handle errors and validation. When making a query or a mutation, you can specify what type of errors you expect to receive and how to handle them. This can be especially useful for handling user input errors, for example.
An example of how to handle errors in a GraphQL mutation is by specifying the onError
prop in the useMutation
hook and handling the errors in your component:
const [createMovie, { data, error }] = useMutation(CREATE_MOVIE, { onError: (error) => { // handle errors here }});
To sum up, GraphQL is a powerful tool that allows you to handle data in your React application in a more efficient and flexible way. By using a library like Apollo Client, you can easily work with GraphQL in your React application. Remember to take advantage of the built-in error handling and validation features, it can help you to develop more robust and error-free applications.
Original Link: https://dev.to/abhaysinghr1/understanding-and-using-graphql-in-react-a-beginners-guide-45an

Dev To
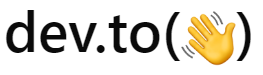
More About this Source Visit Dev To