An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
Connect Wallet to React dApp with ConnectKit
ConnectKit is a React library for connecting dApps to wallets. As at the time of writing this article, the v1.1.1 edges other competitors with typescript support, custom themes, and language translation.
This article was written with the assumption that the reader is familiar with css, javascript, react and blockchain rudimentary terms. Experience with ChakraUI is a plus.
Let's get to business.
Create a react app - of course
npx create-react-app hello-wallet
Install ConnectKit and its peer dependences
Co-dependencies for connection include wagmi and ethers , as connectKit uses both behind the scenes
npm install connectkit wagmi ethers
Create a client
I would suggest creating a separate js file for client creation to make the code cleaner. Let's name this file connectWeb3.js.
You'll need to generate one of Alchemy or Infura endpoint and key. These need to be provided to allow for walletConnect (an open source protocol that helps connect decentralised applications to mobile wallets with QR code scanning or deep linking)
ConnectKit also allows you to switch between networks seamlessly. You can do this by adding chains from wagmi. I am adding 4 chains here : Eth-mainnet, polygon, optimism and arbitrum.
connectWeb3.js
import { createClient } from "wagmi";import { mainnet, polygon, optimism, arbitrum } from "wagmi/chains"; import { getDefaultClient } from "connectkit";export const alchemyId = process.env.ALCHEMY_ID;const chains = [mainnet, polygon, optimism, arbitrum];export const client = createClient( getDefaultClient({ appName: "hello-wallet", alchemyId, chains, }));
Now that our client is set up, we move to the next step.
Wrap the App
Wrap the app with WagmiConfig from Wagmi and ConnectKitProvider from Conneckit. Since this is just a demonstration for this article, only one component - HelloWalletComponent - is included.
App.js
import { WagmiConfig } from "wagmi";import { client } from "./connectWeb3";import { ConnectKitProvider } from "connectkit";function App() { return ( <WagmiConfig client={client}> <ConnectKitProvider> <HelloWalletComponent/> </ConnectKitProvider ></WagmiConfig>);export default App;
Let's go for the button
You can simply import the connect button and have it displayed like the image below this code block.
HelloWalletComponent.js
import { Box, Flex, Text, VStack } from "@chakra-ui/react";import { ConnectKitButton } from "connectkit";function HelloWalletComponent() { return ( <Box> <Flex height="80vh" justifyContent="center" alignItems="center"> <VStack> <Text fontSize={{ base: "18vw", md: "12vw" }} textAlign="center"> GM <span> fren </span> </Text> //button here <ConnectKitButton /> //button here </VStack> </Flex> </Box> );}
Usually you would want the button matched up with your app theme. How then does one go about this? Let's have a walk through in the following sections.
Customization
There are quite a number of ways to do this. For brevity purpose, I'll discuss this just the way I think it's best. You can check out the Docs if you want to satisfy your curiosity.
ConnectKit provides a custom component - ConnectKitButton.Custom - that has render props for necessary wallet connection states. You can take advantage of this to build components like buttons, connection status signals, ens name / wallet address display or in fact make dynamic stylings.
The styling in the code block below takes the "sx" - ChakraUI's way of writing the style prop. That's why the styling is written as an object with cssv attributes in camel cases.
You can simply pass in the stylings with classNames, utility classes, or any form the react framework you use allows.
HelloWalletComponent.js
import { Box, Button, Flex, Text, VStack } from "@chakra-ui/react";import { ConnectKitButton } from "connectkit";function HelloWalletComponent() { const buttonStyle = { color: "black", fontSize: "2rem", border: "solid 2px black", padding: "2rem", fontFamily: "Bebas Neue", background: "transparent", fontWeight: "500", _hover: { border: "solid 1px black", }, }; return ( <Box> <Flex height="80vh" justifyContent="center" alignItems="center"> <VStack> <Text fontSize={{ base: "18vw", md: "12vw" }} textAlign="center"> GM <span> fren </span> </Text> //button here <ConnectKitButton.Custom> {({ isConnected, isConnecting, show, hide, address, truncatedAddress, ensName, }) => { return ( <Button onClick={show} sx={buttonStyle}> {isConnected ? truncatedAddress : "Connect wallet og"} </Button> ); }} </ConnectKitButton.Custom> //button here </VStack> </Flex> </Box> );}
It is important to mention that Connectkit provides you with custom themes, and ability to customize the theme and mode. You can override the styles of a theme by passing providing a customTheme prop to the ConnectKitProvider . You can modify styling variables for:
the whole ConnectKit System - just as the font-family, border-radius, and background are modified in the example below
Connect Wallet Button
Primary Buttons
Secondary Buttons
Tertiary Buttons
Modal
Texts and Miscellaneous
There is also an options prop that allows for more customizations. It is recommended that the embedGoogleFonts is set to true to ensure fonts appear correctly. Other options take mostly booleans and strings.
App.js
import { WagmiConfig } from "wagmi";import { client } from "./connectWeb3";import { ConnectKitProvider } from "connectkit";function App() { return ( <WagmiConfig client={client}> <ConnectKitProvider mode="light" customTheme={{ "--ck-font-family": "Bebas Neue", "--ck-border-radius": "0px", "--ck-background": "#F0FFF3", }} options={{ embedGoogleFonts: true, disclaimer: <> connect your wallet fren. Welcome to Web3 </>, showBalance: true, hideQuestionMarkCTA: true, }}> <HelloWalletComponent/> </ConnectKitProvider ></WagmiConfig>);
All the chains imported from wagmi are rendered as shown in the image below in the connectWeb3.js fileconst chains = [mainnet, polygon, optimism, arbitrum];
That's all I have got in my tiny head for now fren. I would appreciate your suggestions, and other ways you have done this implementation.
Gm and Happy Hacking!
Original Link: https://dev.to/samuelafolabi/connect-wallet-to-react-dapp-with-connectkit-2i3l

Dev To
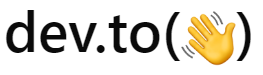
More About this Source Visit Dev To