An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
Introduction to variables in JavaScript
Variables are an essential part of any programming language, and JavaScript is no exception. In this article, we'll cover everything you need to know about variables in JavaScript, including how to declare them, assign values to them, and use them in your code.
Declaring variables in JavaScript
To declare a variable in JavaScript, you use the var keyword followed by the name of the variable. For example:
var myVariable
You can also declare multiple variables at once by separating them with commas:
var firstVariable, secondVariable, thirdVariable;
Starting with ECMAScript 6 (also known as ECMAScript 2015), you can also use the let
and const
keywords to declare variables. These keywords behave slightly differently than var
, as we'll see later in this article.
Assigning values to variables
Once you've declared a variable, you can assign a value to it using the assignment operator (=
). For example:
myVariable = 'Hello, world!';
You can also declare and assign a value to a variable in a single line:
var newVariable = 'Hello, world!';
Naming Variables
In JavaScript, there are a few rules to follow when naming variables:
Variable names must start with a letter, dollar sign ($
), or underscore (_
). They cannot start with a number.
Variable names are case-sensitive. This means that myVariable
and myvariable
are treated as two separate variables.
Variable names cannot contain spaces or special characters, with the exception of the dollar sign and underscore.
Variable names should be descriptive and meaningful, as this makes your code easier to read and understand.
It's also a good idea to follow a naming convention when declaring variables. One common convention is camelCase
, where the first letter of each word is capitalized except for the first word, which is all lowercase. For example, myVariable
or userName
.
Another convention is snake_case
, where all letters are lowercase and words are separated by underscores. For example, my_variable
or user_name
.
There are a few words that are reserved in JavaScript and cannot be used as variable names. These include break
, case
, catch
,continue
, debugger
,default
,delete
, do
, else
, finally
,for
, function
, if
, in
, instanceof
, new
, return
,switch
, this
, throw
, try
,typeof
, var
, void
, while
, and with
.
It's important to follow these rules and conventions when naming variables in JavaScript to ensure that your code is clear, readable, and easy to understand.
Using variables
To use a variable in your code, simply refer to its name. For example:
var myVariable = 'Hello, world!'; console.log(myVariable); // Outputs 'Hello, world!'
Variable scope
In JavaScript, variables have either global or local scope. Global variables are variables that are declared outside of any function and can be accessed from anywhere in your code. Local variables, on the other hand, are variables that are declared inside a function and are only accessible within that function.
Here's an example of a global variable:
var globalVariable = 'I am a global variable'; function myFunction() { console.log(globalVariable); // Outputs 'I am a global variable' } console.log(globalVariable); // Outputs 'I am a global variable'
And here's an example of a local variable:
function myFunction() { var localVariable = 'I am a local variable'; console.log(localVariable); // Outputs 'I am a local variable' } console.log(localVariable); // Throws an error because localVariable is not defined
The keyword highlight
As we mentioned earlier, you can use the var
keyword to declare variables in JavaScript. However, there are a few things you should be aware of when using var
.
First, var
declarations are hoisted to the top of the current scope. This means that you can use a var
declaration before it's actually declared in your code. For example:
console.log(myVariable); // Outputs undefinedvar myVariable = 'Hello, world!';
Second, var
declarations are not block-scoped. This means that if you declare a variable with var
inside a block of code (e.g. an if
statement or a for
loop), that variable will still be accessible outside of the block. For example:
if (true) { var myVariable = 'I am a block-scoped variable';}console.log(myVariable); // Outputs 'I am a block-scoped variable'
In the above code example, the variable myVariable
is declared within the block of an if
statement, but it is still accessible outside of the block. This is because variables declared with the var
keyword are not block-scoped in JavaScript.
This means that it is not limited to the block of code in which it is declared, like an if
statement for example. Instead, it is only scoped to the current function or the global scope. This can lead to unexpected behavior, which is why the let
, and const
keywords were introduced in ECMAScript 6 (also known as ECMAScript 2015). Let
and const
are block-scoped, meaning they are limited to the block of code in which they are declared. The main difference between let
and const
is that variables declared with let
can be reassigned, while const
variables cannot be reassigned.
Conclusion
So to sum it up, variables are a crucial part of JavaScript and are used to store and manipulate data in your code. Just remember to use the appropriate keyword (var
, let
, or const
) and to be aware of the scope of your variables. With this understanding, you should be well-equipped to work with variables in your projects!
Original Link: https://dev.to/lordsonsilver/introduction-to-variables-in-javascript-49nm

Dev To
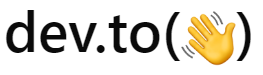
More About this Source Visit Dev To