An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
7 Best Node.js Logging Libraries for Your Next Projects
When you are developing a production-ready application, logging is one of the most important things that you need. It helps you track the execution flow and get insights into why certain things happen in your application. If you monitor your logs, you can easily see if there are any issues or anomalies happening in your app.
To help you monitor and debug problems in your Node.js application, we have compiled a list of the best logging libraries for Node.js apps. They will also assist you in identifying issues before they impact your users; enabling you to fix them before they become major problems.
Heres our list of the top Node.js logging libraries
Winston
Winston is a popular logging library. It is designed to be simple and enables universal logging with support for multiple transports. Winston defines itself as A logger for just about everything.
With Winston, we can route your logs to other services like AWS cloud watch, graylog2, logz.io, or even Sematext Logsene. We can even add the express middleware to make logging with Express better with Winston.
Below is a code example of Winston with Express using express-winston middleware:
const winston = require('winston');const expressWinston = require('express-winston');const express = require('express');const app = express();const port = 3001;app.use(expressWinston.logger({ transports: [ new winston.transports.Console() ], format: winston.format.combine( winston.format.colorize(), winston.format.json() ), meta: false, msg: "HTTP ", expressFormat: true, colorize: false, ignoreRoute: function (req, res) { return false; }}));app.get('/', (req, res) => { res.send('Hello World! - Winston logged');});app.get('/api/test', (req, res) => { res.json({'message': 'Hello winston!'});});app.listen(port, () => { console.log(`Example app listening at http://localhost:${port}`);});
It will give an output as follows when we run the server with node winston.js and hit http://localhost:3001/test/api on the browser:
Example app listening at http://localhost:3001
{"meta":{},"level":"\u001b[32minfo\u001b[39m","message":"GET /api/test 304 2ms"}{"meta":{},"level":"\u001b[32minfo\u001b[39m","message":"GET /favicon.ico 404 2ms"}{"meta":{},"level":"\u001b[32minfo\u001b[39m","message":"GET /favicon.ico 404 1ms"}
Bunyan
Bunyan is also another popular and fast JSON Node.js logging library. Just like Winston, it also supports logging into multiple transport options. Other features include a neat-printing CLI for logs, a log filter, serializers for rendering objects, snooping system, and the ability to support multiple runtime environments such as NW.js and WebPack. Bunyan enforces the JSON format for logs.
Install Bunyan using npm.
npm install bunyan
Once installed, you can now proceed to create a logger instance before calling methods corresponding to each log severity.
const bunyan = require('bunyan');const log = bunyan.createLogger({ name: 'myapp', streams: [ { level: 'info', stream: process.stdout }, { level: 'error', path: './myapp-error.log' } ] });log.info("This is log of level info");log.error("This is a log of error level");
Logs with the log severity of the error and less you can write to a file named myapp-error.log, while logs with a log level of info and less you can write to the process.stdout. The logs are formatted in JSON.
{"name":"myapp","hostname":"DESKTOP-T8C4TF4","pid":19164,"level":30,"msg":"This is log of level info","time":"2022-05-28T19:38:46.486Z","v":0}{"name":"myapp","hostname":"DESKTOP-T8C4TF4","pid":19164,"level":50,"msg":"This is a log of error level","time":"2022-05-28T19:38:46.487Z","v":0}
If youre using Express the express-bunyan-logger is a middleware powered by bunyan that you can enable to perform logging for your Express applications.
const express = require('express');const app = express();const port = 8080;app.use(require('express-bunyan-logger')({ name: 'logger', format: ":remote-address - :user-agent[major] custom logger", streams: [{ level: 'info', stream: process.stdout }, { level: 'error', path: './newapp-error.log' }]}));app.get('/', (req, res) => { res.send('Express application has been logged !');});app.get('/newpage/', (req, res) => { res.json({'message': 'Hello Developer!'});});app.listen(port, () => { console.log(`The application is listening at http://localhost:${port}`);});
When we execute the code above and hit the endpoint localhost:8080, logs with a severity level of error or less and those with the severity level of info or less are written to newapp-error.log and the console respectively. In both cases, the logs are formatted in JSON.
{"name":"logger","hostname":"Thomas","pid":15124,"req_id":"83ff8181-89d2-4bd5-9db3-a3121ee7a194","level":30,"remote-address":"::1","ip":"::1","method":"GET","url":"/","referer":"-","user-agent":{"family":"Chrome","major":"99","minor":"0","patch":"4844","device":{"family":"Other","major":"0","minor":"0","patch":"0"},"os":{"family":"Windows","major":"10","minor":"0","patch":"0"}},"http-version":"1.1","response-time":747.4553,"response-hrtime":[0,747455300],"status-code":304,"req-headers":{"host":"localhost:8080","connection":"keep-alive","upgrade-insecure-requests":"1","user-agent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/99.0.4844.74 Safari/537.36","accept":"text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9","sec-gpc":"1","sec-fetch-site":"none","sec-fetch-mode":"navigate","sec-fetch-user":"?1","sec-fetch-dest":"document","accept-encoding":"gzip, deflate, br","accept-language":"en-US,en;q=0.9","cookie":"io=aQJ9Q4pzOENJkdftAAAC; connect.sid=s%3AvyrJ3mrWR2NERi4Fpi-E3xyYBDbW1m-O.uQdVPjtzJEKeN9XA29f4FvKG2icEnRI0MAdehMLy7r0","if-none-match":"W/\"20-HIBUXsVCk/nPIIFJblENJC3SnAg\""},"res-headers":{"x-powered-by":"Express","etag":"W/\"20-HIBUXsVCk/nPIIFJblENJC3SnAg\""},"req":{"method":"GET","url":"/","headers":{"host":"localhost:8080","connection":"keep-alive","upgrade-insecure-requests":"1","user-agent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/99.0.4844.74 Safari/537.36","accept":"text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9","sec-gpc":"1","sec-fetch-site":"none","sec-fetch-mode":"navigate","sec-fetch-user":"?1","sec-fetch-dest":"document","accept-encoding":"gzip, deflate, br","accept-language":"en-US,en;q=0.9","cookie":"io=aQJ9Q4pzOENJkdftAAAC; connect.sid=s%3AvyrJ3mrWR2NERi4Fpi-E3xyYBDbW1m-O.uQdVPjtzJEKeN9XA29f4FvKG2icEnRI0MAdehMLy7r0","if-none-match":"W/\"20-HIBUXsVCk/nPIIFJblENJC3SnAg\""},"remoteAddress":"::1","remotePort":61169},"res":{"statusCode":304,"header":"HTTP/1.1 304 Not Modified\r
X-Powered-By: Express\r
ETag: W/\"20-HIBUXsVCk/nPIIFJblENJC3SnAg\"\r
Date: Sat, 10 Sep 2022 15:26:34 GMT\r
Connection: keep-alive\r
Keep-Alive: timeout=5\r
\r
"},"incoming":"<--","msg":"::1 - 99 custom logger","time":"2022-09-10T15:26:35.216Z","v":0}(node:15124) [DEP0066] DeprecationWarning: OutgoingMessage.prototype._headers is deprecated(Use `node --trace-deprecation ...` to show where the warning was created)
Pino
Pino is also a popular Node.js logging library alternative for developers. There are claims that it is 5x faster than other Node.js logging libraries besides providing standard features such as the ability to choose a storage medium, log levels, and formatting capabilities. Pino is also highly extensible, flexible, and easy to integrate with Node.js frameworks such as Express, Fastify, and Restify. The library is a low-overhead Node.js logging library and supports asynchronous logging despite being fast. Pino also gives you the ability to create child loggers.
To install Pino, use the command below.npm install pino
const pino = require('pino');const logger = pino({ level: process.env.NODE_ENV === 'production' ? 'info' : 'debug', });logger.info('Hello, Developer!');const child = logger.child({ a: 'property' })child.info('Hello Developer!')
logs :
{"level":30,"time":1653773121769,"pid":8964,"hostname":"DESKTOP-THOMAS","msg":"Hello, Developer!"}{"level":30,"time":1653773121770,"pid":8964,"hostname":"DESKTOP-THOMAS","a":"property","msg":"Hello Developer!"}
Alternatively, you could create this logging instance in a separate file, export it and use it throughout your project. As aforementioned, Pino is also easy to configure Node.js logging library, especially when working with other frameworks. For example, using the express-pino logger, you can also log your express application. To install the middleware, we use this command.
npm install express-pino-logger --save
Here is a simple Express web server with two endpoints illustrating how we can use the express-pino-logger middleware. Since Pino logs are very verbose you can also use the pino-pretty module to format the logs. To install the module use the command shown below.
'use strict'const express = require('express');const app = express();const pino = require('express-pino-logger')();const PORT = process.env.PORT || 8080;app.use(pino)app.get('/', (req, res) => { res.send('Your express app has been logged');});app.get('/newpage/', (req, res) => { req.log.info(); res.json({'message': 'Hello Dev!'});});app.listen(PORT, () => { console.log(`The application is listening at http://localhost:${PORT}`);});
Logs:
{"level":30,"time":1662818930367,"pid":12764,"hostname":"Thomas","req":{"id":1,"method":"GET","url":"/",host":"localhost:8080","connection":"keep-alive","upgrade-insecure-requests":"1","user-agent":"Mozilla/5guage":"en-US,en;q=0.9","cookie":"io=aQJ9Q4pzOENJkdftAAAC; connect.sid=s%3AvyrJ3mrWR2NERi4Fpi-E3xyYBDbW1m-O.uQdVPjtzJEKeN9XA29f4FvKG2icEnRI0MAdehMLy7r0"},"remoteAddress":"::1","remotePort":61303},"res":{"statusCode":200,"headers":{"x-powered-by":"Express","content-type":"text/html; charset=utf-8","content-length":"32","etag":"W/\"20-HIBUXsVCk/nPIIFJblENJC3SnAg\""}},"responseTime":18,"msg":"request completed"}{"level":30,"time":1662818936220,"pid":12764,"hostname":"Thomas","req":{"id":2,"method":"GET","url":"/favicon.ico","query":{},"params":{},"headers":{"host":"localhost:8080","connection":"keep-alive","user-agent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/99.0.4844.74 Safari/537.36","accept":"image/avif,image/webp,image/apng,image/svg+xml,image/*,*/*;q=0.8","sec-gpc":"1","sec-fetch-site":"same-origin","sec-fetch-mode":"no-cors","sec-fetch-dest":"image","referer":"http://localhost:8080/","accept-encoding":"gzip, deflate, br","accept-language":"en-US,en;q=0.9","cookie":"io=aQJ9Q4pzOENJkdftAAAC; connect.sid=s%3AvyrJ3mrWR2NERi4Fpi-E3xyYBDbW1m-O.uQdVPjtzJEKeN9XA29f4FvKG2icEnRI0MAdehMLy7r0"},"remoteAddress":"::1","remotePort":61315},"res":{"statusCode":404,"headers":{"x-powered-by":"Express","content-security-policy":"default-src 'none'","x-content-type-options":"nosniff","content-type":"text/html; charset=utf-8","content-length":150}},"responseTime":3,"msg":"request completed"}
LogLevel
A relatively popular, simple, minimal and lightweight Node.js logging library that works both in the browser and for Node.js. According to its documentation, the LogLevel Node.js logging libraries seek to replace the console methods with more features such as the ability to disable error logging in production and filter logs by their severity. LogLevel is a Node.js logging library that has no dependencies and can continue logging even in browsers that dont have support for the console object. Like other Node.js logging libraries, LogLevel is also extensible and offers log redirection capabilities, formatting, and filtering. For developers that develop in TypeScript, LogLevel already has included type definitions making it even more convenient to use.
Below is an example of using the LogLevel logging library under the CommonJS module specification, particularly on the server side with Node.js. If youre using npm, you can install LogLevel using the command below.
npm install loglevel
const log = require('loglevel');log.warn("This is a warning !");log.error("This is an error !");
Executing the code above with node index.js should return the following output in the console.
This is a warning!This is an error !
You can also use the LogLevel Node js logging library for logging with other Node js frameworks, for example in express js without any middleware. Here is a simple web server with a single endpoint.
const express = require('express');const app = express();const logger = require('loglevel');const port = 8080;app.get('/', (req, res) => { logger.warn('This is a warning'); res.json({'message': 'Hello Developer !'}); logger.error('This is an error');});app.listen(port, () => { console.log(`The application is listening at http://localhost:${port}`);});
Tracer
Tracer calls itself the customizable and powerful logging library for Nodejs. With Tracer, you can print simple logs, colored logs, and even set output levels. You can customize it by printing a timestamp, file name, line number, and even the call stack! The list is huge!
You can download it using the below NPM command:npm i tracer
var logger = require('tracer').console();logger.log('hello');logger.trace('hello', 'world');logger.debug('hello %s', 'world', 123);logger.info('hello %s %d', 'world', 123, {foo:'bar'});logger.warn('hello %s %d %j', 'world', 123, {foo:'bar'});logger.error('hello %s %d %j', 'world', 123, {foo:'bar'}, [1, 2, 3, 4], Object);
Logs:
2022-09-10T13:59:30+0000 <log> tracer.js:3 (Object.<anonymous>) hello2022-09-10T13:59:30+0000 <trace> tracer.js:4 (Object.<anonymous>) hello world2022-09-10T13:59:30+0000 <debug> tracer.js:5 (Object.<anonymous>) hello world 1232022-09-10T13:59:30+0000 <info> tracer.js:6 (Object.<anonymous>) hello world 123 { foo: 'bar' }2022-09-10T13:59:30+0000 <warn> tracer.js:7 (Object.<anonymous>) hello world 123 {"foo":"bar"}2022-09-10T13:59:30+0000 <error> tracer.js:8 (Object.<anonymous>) hello world 123 {"foo":"bar"} [ 1, 2, 3, 4 ] function Object() { [native code] }
Signale
Signale consists of 19 loggers for Javascript applications. It supports TypeScript and scoped logging. It consists of timers that help log the timestamp, data, and filename. Apart from the 19 loggers like await, complete, fatal, fav, info, etc., one can create custom logs.
Custom logs are created by defining a JSON object and fields with the logger data. Interactive loggers can also be created. When an interactive logger is set to true, new values from interactive loggers override the old ones.
Morgan
Morgan is a logging tool (middleware) that can be used in HTTP servers implemented using Express & Node.js. It can be used to log requests, errors, and more to the console.
To install the middleware, use this command:npm i morgan
Usage of Morgan with express in development mode:
const express = require('express');const logger = require('morgan');const port = 3000;const app = express();app.use(logger('dev'));app.get('/', (req, res) => { res.send('<h1>Front Page</h1>');});app.listen(port, () => { console.log(`Started at ${port}`);});
Logs:
GET / 200 9.428 ms - 19GET /favicon.ico 404 4.822 ms - 150
Summary
This list of the best logging libraries for Node.js apps has been compiled with the goal of making it easy for you to find the one that meets your needs. If you use one of these libraries, you can rest assured you will be able to effectively monitor the health of your applications, troubleshoot and debug issues, and save important information for later use.
Thanks for reading! If you have any questions or feedback, please leave a comment below.
Original Link: https://dev.to/devland/7-best-nodejs-logging-libraries-for-your-next-projects-38df

Dev To
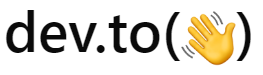
More About this Source Visit Dev To