An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
October 15, 2022 06:08 am GMT
Original Link: https://dev.to/rajivchaulagain/uploading-image-with-formik-and-base64-2loa
Uploading image with Formik and base64
Lets upload image with formik and react js.
Now,Base64 is a group of binary-to-text encoding schemes that represent binary data (more specifically, a sequence of 8-bit bytes) in sequences of 24 bits that can be represented by four 6-bit Base64 digits.
Now we will create a single image .
const singleImage = () => { const convertToBase64 = (file) => { return new Promise((resolve, reject) => { const fileReader = new FileReader(); fileReader.readAsDataURL(file); fileReader.onload = () => { resolve(fileReader.result); }; fileReader.onerror = (error) => { reject(error); }; }); }; const handleIcon = async (e, setFieldValue) => { const file = e.target.files[0]; //check the size of image if (file?.size/1024/1024 < 2) { const base64 = await convertToBase64(file); setFieldValue('profile_image', base64); } else { toast.error('Image size must be of 2MB or less'); }; }; return ( <div className="mb-3 form-group"> <label className="required">Upload Photo</label> <Field name='profile_image'> {({ form, field }) => { const { setFieldValue } = form return ( <input type="file" className='form-control' required onChange={(e) => handleIcon(e, setFieldValue)} /> ) }} </Field> <div className="text-danger"> <ErrorMessage name="profile_image" /> </div> </div> )};
So, here formik handles the image and then we take the image from onchange function and check the size and if the size is less than the condition then , convertToBase64 function converts the image into base64 as string and hence the image is converted to base64.
const multipleImage = () => {export const convertToBase64 = (file) => { return new Promise((resolve, reject) => { const fileReader = new FileReader(); fileReader.readAsDataURL(file); fileReader.onload = () => { resolve(fileReader.result); }; fileReader.onerror = (error) => { reject(error); }; }); };const handleProfile = async (e, setFieldValue) => { const file = e.target.files[0]; if (file?.size / 1024 / 1024 < 2) { const base64 = await convertToBase64(file); setFieldValue('image', base64); } else { toast.error('Image size must be of 2MB or less'); };return ( <div className="mb-3 form-group"> <label>Upload Cover Photo</label> <Field name={name}> {({ form, field }) => { const { setFieldValue } = form return ( <input type="file" className='form-control' onChange={(e) => handleProfile(e, setFieldValue)} /> ) }} </Field> </div>)}
Conclusion
Hence in the formik data we can find our image as converted to base64.
Original Link: https://dev.to/rajivchaulagain/uploading-image-with-formik-and-base64-2loa
Share this article:
Tweet
View Full Article

Dev To
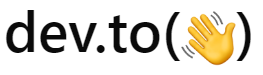
More About this Source Visit Dev To