An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Debugging in JavaScript
Since bugs are quite obvious in software development, Debugging is a skill that every software developer should learn to succeed in their career.
Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.
Benjamin Kernighan
Debugging is a part of software testing process which involves finding and fixing errors in a software before releasing it to the public. It entails not only inserting corrective code but also removing defective code.
Besides, that it helps software developers understand why and how things are happening in the code. As a result, debugging helps software developers learn faster and better.
Types of errors to debug
1. Syntax errors
A syntax error occurs when a programming rule is broken. For instance, misspelling keywords, using a variable that has not been declared, or failing to close an opening bracket.
Note that, syntax errors depend on the syntax rules of the programming language.
The execution of the program stops when a syntax error is encountered and an error is thrown. Hence, it is relatively easy to find syntax errors.
2. Logic errors
Logic error occurs when a program produces an unexpected output. Such errors are usually caused by algorithm issues such as
- Incorrect program design. Use flowcharts to visualize the code flow
- Creating an infinite loop without a break statement
- Incorrect use of Booleans or logical operators
- Having the same variable names for different objects
The key difference between logic and syntax errors is that a program with logic error will run but produce an unexpected result. On the other hand, a program with logic error will not run.
3. Run-time errors
Unlike other errors which occur when you are interacting with the code on your device, Run time errors occur when the user is interacting with the software.
For instance, if a user clicks a button to display the sixth element in an array of 5 elements which returns an undefined message.
How to debug in JavaScript
The common ways to debug JavaScript code are;
Using the console
The console object provides an access to the browser debugging console by using ctrl + shift + I for windows and command + option + k for mac.
Besides console.log() method which you must have interacted with as a JavaScript dev, there several other console methods.
JavaScript Console methods
- console.log()
Console.log() prints an output on the console. You can use it to check the input or outputs of a program.
For instance;
const sum = function( x, y){ return x + 'y' } sum(2,'3')
We expect the above function to return 5, however it returns 23. It is quite easy to spot the error here since the arguments are static but could be hard if the arguments were dynamically received from another function.
You can console.log each of the parameters in the function to check their types
const sum = function( x, y){ console.log(typeof x, y) // prints number string return x + 'y' } sum(2,'3')
2.console.table()
console.table() displays an array or object as a table. It helps visualize various object keys and their values. For instance;
const user = [ { name: "Erica", role: "Admin", department: "IT", }, { name: "Wanja", role: "Hr", department: "IT", },];console.table(user);//output (index) name role department 0 'Erica' 'Admin' 'IT' 1 'Wanja' 'Hr' 'IT'
3.Console.group()
Have you ever had several console messages that it was difficult to differentiate them? Using console.group() helps group the console messages into indented blocks. Even better you can add a label to help identify them.
To exit a console group, use console.groupEnd() as shown below.
function userData(users_details){ console.group('userData') console.log('first user', users_details[0].name ) console.groupEnd()}userData(user) /*outputuserData first user Erica*/
- Console.trace()When interacting with a large codebase, it might be strenuous to understand where various functions have been declared. Console.trace() helps to trace the call path of the function.
function foo() { function bar() { console.trace(); } bar();}foo();
outputs;
- console.assert()
Console.assert prints a message to the console if the expression evaluates to false
syntax: console.assert(expression, message)
console.assert(x + y == 11, "Expression returned false"); // prints the message if the sum is not equal to `11
Debugger
The debugger
keyword stops code execution and calls for available debugging functionalities.
Run the below code on the browser and inspect to see the debugger
in action. You can check on this demo to understand JavaScript debugger and breakpoints.
let a = 3;let b = 5;debugger;console.log(a*b)
3. Final Words
Follow me on twitter
More resources on debugging
Original Link: https://dev.to/ericawanja/debugging-in-javascript-4ppb

Dev To
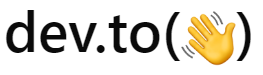
More About this Source Visit Dev To