7 Must Know JavaScript Tips & Tricks
Let's have a look at 7 valuable tips and tricks found in the most popular language in the world, JavaScript.
1. Destructuring with Parameters
You can use object destructuring within function parameters. A popular use case for this would be something like event listeners, and gaining access to the target
property on the event object.
buttonElement.addEventListener("click", ({ target }) { // is the same as using e.target console.log(target);});
2. Deep Copy with JSON
You may have heard of using object spread or Object.assign()
to make shallow copies of an object, but did you know about using JSON to make deep copies?
Simply convert an object to JSON using JSON.stringify()
and then re-parse it using JSON.parse()
to create a deep copy.
Just remember to only do this for simple objects, as doing it on large objects may raise performance issues.
const person = { name: "Dom", age: 28, skills: [ "Programming", "Cooking", "Tennis" ]};const copied = JSON.parse(JSON.stringify(person));// false console.log(person.skills === copied.skills);
3. Easy Defaults with OR
I talk about this one a lot. Basically, you can use the logical OR operator (||
) for defaults as opposed to using an if statement.
Alternatively, for stricter comparisons, you can take advantage of the nullish coalescing operator
Old Code (using if statement - 4 lines)
let username = getUsername();if (!username) { username = "Dom";}
New Code (using ||
- 1 line)
const username = getUsername() || "Dom";
The new code also means you can use const
over let
.
4. Advanced Array Searching
Step aside, indexOf()
and includes()
because there's another method that allows for advanced array searching and it's called find()
.
The find()
method allows you to pass in a test function. The first element within the array to pass the test function will be returned.
This makes for more useful array searching.
const occupations = [ "Lawyer", "Doctor", "Programmer", "Chef", "Store Manager",];const result = occupations.find(o => o.startsWith("C"));// "Chef" console.log(result);
5. Remove Array Duplicates
You may have heard of this one before, but there's a really simple way to remove duplicates from an array using the Set
data structure.
Basically, Set
doesn't allow duplicate values. We can take advantage of that by turning an array into a Set
, and then back into an array.
const numbers = [5, 10, 5, 20];const withoutDuplicates = Array.from(new Set(numbers));// [5, 10, 20] console.log(withoutDuplicates);
6. Self-Invoking Functions
This one is a classic. Self-invoking functions are functions that execute themselves. A common use case for these is to assign a variable or constant that requires complex logic.
const someComplexValue = (() => { const a = 10; const b = 20; if (a > b) { return a * b; } return b / a;})();
Of course, the above example is trivial but you can imagine the kind of complex code you may require to be within the self-invoking function.
7. Array Copying with Spread
Last on this list involves creating shallow copies of an array. We can do this using spread (...
).
const numbers = [5, 19, 24, 36];const numbersCopy = [...numbers];// falseconsole.log(numbers === numbersCopy);
I hope you learnt at least something new from this list. For more JavaScript content, check out my YouTube channel, dcode.
Enrol Now JavaScript DOM Crash Course
If you're learning web development, you can find a complete course on the JavaScript DOM at the link below
https://www.udemy.com/course/the-ultimate-javascript-dom-crash-course/?referralCode=DC343E5C8ED163F337E1
Original Link: https://dev.to/dcodeyt/7-must-know-javascript-tips-tricks-2m8f

Dev To
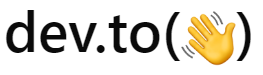
More About this Source Visit Dev To