An Interest In:
Web News this Week
- April 20, 2024
- April 19, 2024
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
How to use ES6 import in Node Project
ES6 Module
A file containing reusable code is known as a module. Whether or not it is declared, the import modules are in strict mode.
Modules that are exported by another module are imported using the import statement.
How to use import Syntax:
import name from 'module-name'
Importing an entire module:
import * as name from 'module-name'
Import default export from a module:
import name from 'module-name'
Importing a single export from a module:
import { name } from 'module-name'
Importing multiple exports from a module:
import { nameOne , nameTwo } from 'module-name'
Importing a module for side effects only
import './module-name'
Exporting a module
export default module_name
Node JS does not directly support ES6 import. If we attempt to utilize node js import function to directly import modules, an error will be generated. For instance, Node JS would raise the following error if we attempt to import the express module by writing import express from "express".
ES modules are experimentally supported by Node. We must make some adjustments to the package.json file in order to enable them. Make sure Node is installed before continuing with the instructions. The steps to do so are shown below.
Add "type": "module" to the package.json file. This is added to enable ES6 modules.
This is how the package.json file should appear:
//package.json
{
"name": "index",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Make a file called index.js, and then use ES6 import to write your program. Let's try importing Express in the index.js file as an example.
//index.js
import express from 'express';
const app = express();
app.get('/', async(req,res) => {
res.send('It is working');
})
const PORT = 5000;
app.listen(PORT,() => {
console.log(Running on PORT ${PORT}
);
})
Another method of utilizing the esm module is to create a different file, such as server.js, which loads esm prior to the application itself. Write the following code in the server.js file.
//server.js
require = require("esm")(module);
module.exports = require("./index.js");
We are importing the index.js file, which contains the actual program that has to be run, into the server.js file.
To run the program, type node server.js into the terminal.
Thanks for your time, I have more helpful content like this, please subscribe to be among the first to get them when they are released.
Original Link: https://dev.to/davidalimazo/how-to-use-es6-import-in-node-project-2ek1

Dev To
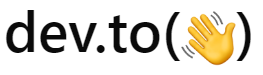
More About this Source Visit Dev To