An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
How to build your first VR app with React360 today!
As a tech head, youre probably aware of virtual reality and its various applications. Video games, web and mobile apps, and more can reap the benefits of VRs amazing features.
If your next development goal is creating VR apps and youre familiar with the React ecosystem, youre in luck. You can now develop amazing VR experiences using React 360 and JavaScript.
In this tutorial, well show you how to develop a simple and interactive React virtual reality application using React 360. By the end, you should be ready to build your first VR app in React.
What is React 360?
React 360 is a library that utilizes a lot of React Native functionality to create virtual reality apps that run in your web browser. It uses Three.js for rendering and comes as an npm package. By combining modern APIs such as WebGL and WebVR with the declarative power of React, React 360 helps simplify the process of creating cross-platform VR experiences.
Learning how to use React 360 is a great way to kickstart your VR development career. In this tutorial, well cover all the basics to help you get started using React 360.
Installing React 360
First and foremost, you need to install the React 360 CLI. This will give you access to all the necessary commands to assist you in developing a virtual reality application.
Now, go to the desired folder through the command terminal and run the following command:
npm install -g react-360-cli
This is a one-time install, so you dont have to do this every time. The benefit of being in the project folder is that it makes the following step easier.
After installation, create a new project called my-project
(creative, right?) and type:
react-360 init my-project
You have successfully created your first virtual reality application with React 360.
To view the app in your browser, navigate to the my-project
folder via the terminal and then run npm start
. This will guide you to the destination in the browser. Alternatively, you can access the output by following http://localhost:8081/index.html
.
Heres what the app should look like:
Now that you have the app up and running, lets talk about the code in detail. Two important files well be working with are client.js
and index.js
. The index.js
file consists of four parts:
Class
Imports
Styles
Component Registry
Were importing React to use its class functionality. Well gather some parts from React 360 to build a VR environment:
import React from 'react';import { AppRegistry, StyleSheet, Text, View,} from 'react-360';export default class my_project extends React.Component { render() { return ( Welcome to React 360 ); }};const styles = StyleSheet.create({ panel: { width: 1000, height: 600, backgroundColor: 'rgba(255, 255, 255, 0.4)', justifyContent: 'center', alignItems: 'center', }, greetingBox: { padding: 20, backgroundColor: '#000000', borderColor: '#639dda', borderWidth: 2, }, greeting: { fontSize: 30, },});AppRegistry.registerComponent('my_project', () => my_project);
The class syntax and components are quite similar to React and React Native, respectively. The View
component allows you to render various aspects of the VR world and, if you want, to play with the look and feel. The Style
attribute and StyleSheet
will help you do that. There are numerous resemblances to React Native and CSS functionality here.
When it comes to the Text
component, you can create dynamic text to display all kinds of data for the user. In the end, the class needs to be registered to the client for rendering.
Youll notice that the init
function in the client.js
file makes a new instance for your project and then utilizes the class from the index.js
file to assign a render method to this project. After that, the project environment is applied to a panoramic image, followed by the execution of the init
function.
import {ReactInstance} from 'react-360-web';function init(bundle, parent, options = {}) { const r360 = new ReactInstance(bundle, parent, { fullScreen: true, ...options, }); r360.renderToSurface( r360.createRoot('my_project', { }), r360.getDefaultSurface() ); r360.compositor.setBackground(r360.getAssetURL('simmes-start-screen.jpg'));}window.React360 = {init};
Those are the basics of React 360 functionality, and youre good to go with your first VR app development. Now that you know how a React VR app is made, lets go through some steps to customize it.
Adding background assets
You can apply any panoramic image to the VR background using React 360. For this tutorial, were using a Simmes free image; you can use any image you like.
To use a panoramic image for the background, add the desired image to the static_assets
folder. This folder contains all the static assets, such as pictures, music, and models, and React 360 searches for them here.
Use the command below to update the background:
r360.compositor.setBackground(r360.getAssetURL('simmes-start-screen.jpg'));
VR interactions
One of the most important and engaging aspects of any application is interaction. Without interaction, an app is lifeless. You can add this vital functionality to your React VR app by adding the VrButton
component to the index.js
files imports as follows:
import { AppRegistry, StyleSheet, Text, View, VrButton} from 'react-360';
Next, add a counter to record the number of clicks. Start by setting the click count to zero:
state = { count: 0};
The next step is to write an increment function:
_incrementCount = () => { this.setState({ count: this.state.count + 1 })}
In the end, well render the VrButton
like so:
<View style={styles.panel}> <VrButton onClick={this._incrementCount} style={styles.greetingBox}> <Text style={styles.greeting}> {`You have visited Simmes ${this.state.count} times`} </Text> </VrButton></View>
Youve successfully set up the button and you can now see the number of people who visit your VR world.
Adding immersive sounds
Not all apps you create will require sound. However, when it comes to games, videos, and other immersive experiences, sounds are essential.
To add sound to our VR app, we need to fetch a few more things from React 360.
import { asset, AppRegistry, NativeModules, StyleSheet, Text, View, VrButton} from 'react-360';
The next step is to import the AudioModule
from NativeModules
and set a new const
:
const { AudioModule } = NativeModules;
Once the new const
is set, we can add specific functionality to the way the sound plays. For example, we can make it start playing upon the click of the button and stop when the button is clicked again.
To make this work, well add a Boolean value to the state:
state = { count: 0, playSound: false};
Finally, well write another function to manage the way sound plays:
_playSound = () => { this.setState({ playSound: !this.state.playSound }); if (this.state.playSound) { AudioModule.createAudio('sza', { source: asset('brokenclocks.mp3'), volume: 0.5 }); AudioModule.play('sza'); } else { AudioModule.stop('sza'); } }
Once executed, this function upgrades the playSound
state, which is set to false
. The way the sound plays will depend on the value assigned to playSound
. To make it play, an audio instance has to be created via the createAudio
component.
Once created, you can play the audio via the assigned name. This only occurs when playSound
is set to true
. The sound stops playing when it is false
. Thats why we make a new instance every time playSound
is true
.
Well now create a button to start and stop playing the sound. Heres what the code will look like:
<View style={styles.panel}> <VrButton onClick={this._incrementCount} style={styles.greetingBox}> <Text style={styles.greeting}> {`You have visited Simmes ${this.state.count} times`} </Text> </VrButton> <VrButton onClick={this._playSound} style={styles.greetingBox}> <Text style={styles.greeting}> {'You can play the music of your people'} </Text> </VrButton></View>
Your VR app is now complete! Lets run the application to see how it looks.
Running your React VR application
You can view the app via the run npm start
command. Your first React VR app, called My Project, should contain the background that you chose and two buttons. One button controls the audio and the other keeps track of the number of users who visit the application.
You can now invite your friends and family to play with your new VR app and brainstorm new ideas for the app with fellow programmers. The possibilities are endless.
I hope this tutorial helped you understand React 360 a little better.
Tell us about your experience with React 360. What do you think is the best way to create VR apps? We would love to hear from you.
*Thats it and if you found this article helpful, please hit the , button and share the article, so that others can find it easily :) *
If you want to see more of this content you can support me on Patreon!
Have a nice day!
Original Link: https://dev.to/kai_wenzel/how-to-build-your-first-vr-app-with-react360-today-4loi

Dev To
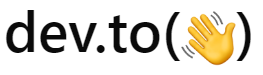
More About this Source Visit Dev To