An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
Recursive Function to Find All Folder Paths in vCenter Server Inventory using PowerCLI
This is a PowerShell / PowerCLI script I wrote to find all folder paths in a vCenter Server Inventory. A recursive function walks through the entire folder tree, pushing and popping each encountered folder to a stack to generate each full path. The paths are stored in an ArrayList, which is finally output to a text file.
Code
function build_folder_paths { Param($folders) foreach($folder in $folders) { $stack.Push($folder.Name) $sub_folders = $folder | Get-Folder -type VM -norecursion $stack_array = $stack.ToArray() [array]::Reverse($stack_array) $folder_path = $stack_array -join "/" $folder_paths.Add($folder_path) Write-Host("Folder Path: " + $folder_path) if(!$sub_folders) { try { $stack.Pop() | Out-Null } catch { Write-Host("Stack Empty.") } } else { build_folder_paths($sub_folders) } } try { $stack.Pop() | Out-Null } catch { Write-Host("Stack Empty.") } } $folder_paths = New-Object -TypeName "System.Collections.ArrayList" $stack = New-Object -TypeName "System.Collections.Stack" $start_folders = Get-Folder VM -type VM | Get-Folder -type VM -norecursion build_folder_paths($start_folders) $folder_paths | Out-File ./folder_paths.txt
Code Explanation
- Function
- A function
build_folder_paths
is defined that accepts a$folders
parameter.- On first-call, the
$folders
parameter holds the list of folders at the root of the vCenter inventory. - For each
$folder
in$folders
:- We push
$folder
to the stack. - We fetch the immediate
$sub_folders
of that$folder
. - We convert the stack to an array and reverse it so the top of the stack has the highest index.
- We join the array with
/
to build the folder path. - We add the
$folder_path
to the$folder_paths
ArrayList. - If there are not
$sub_folders
in this$folder
, we pop the stack.- Then, the
foreach
loop iterates to the next$folder
in$folders
.
- Then, the
- If there are
$sub_folders
in this$folder
, then we make a recursive call to the function and pass$sub_folders
it.- After all
$sub_folders
are processed on the recursive call, we pop the parent$folder
from the stack, and the recursive call returns.
- After all
- We push
- When a recursive call returns, its caller continues.
- On first-call, the
- A function
- Start
- We create a
$folder_paths
ArrayList to store all the folder paths. - We create a
$stack
to build each folder path. - We fetch the
$start_folders
by getting all the immediate sub-folders at the root of the vCenter inventory folder tree. - We call the
build_folder_paths
function and pass$start_folders
as the$folders
parameter.- This function recursively calls itself until every folder is visited, and every path is generated.
- We create a
- End
- Finally, the content of the
$folder_paths
ArrayList is written to a text file.
- Finally, the content of the
Simple Example
Let's say we have the following folder structure:
folder1 folder1a folder1b folder2
1. The function looks at folder1
, pushes it to the stack, fetches its sub-folders, builds its folder path, and adds its path to the ArrayList.
stack: folder1 <-- top sub-folders: ['folder1a','folder1b'] path: folder1 ArrayList: ['folder1']
- Since there are sub-folders in
folder1
, we make a recursive call to the functionbuild_folder_paths
and pass$sub_folders
it.
2. The function looks at folder1a
, pushes it to the stack, fetches its sub-folders, builds its folder path, and adds its path to the ArrayList.
stack: folder1a <-- top folder1 sub-folders: none path: folder1/folder1a ArrayList: ['folder1','folder1/folder1a']
Since there are no sub-folders in
folder1a
, it is popped from the stack, and theforeach
loop proceeds tofolder1b
.stack: folder1 <-- top
3. The function looks at folder1b
, pushes it to the stack, fetches its sub-folders, builds its folder path, and adds its path to the ArrayList.
stack: folder1b <-- top folder1 sub-folders: none path: folder1/folder1b ArrayList: ['folder1','folder1/folder1a', 'folder1/folder1b']
Since there are no sub-folders in
folder1b
, it is popped from the stack.stack: folder1 <-- top
Since there are no more folders for the
foreach
loop to process (remember, we are processing the sub-folders offolder1
), theforeach
loop exits andfolder1
is popped from the stack.stack: empty
The recursive function call returns, and the initial function call continues.
4. The function looks at folder2
, pushes it to the stack, fetches its sub-folders, builds its folder path, and adds its path to the ArrayList.
stack: folder2 <-- top sub-folders: none path: folder2 ArrayList: ['folder1','folder1/folder1a', 'folder1/folder1b', 'folder2']
Since there are no sub-folders in
folder2
, it is popped from the stack.stack: empty
Since there are no more folders for the
foreach
loop to process (remember, we are processing the folders from the initial function call), theforeach
loop exits.An attempt is made to pop the parent folder from the stack, but the stack is empty, and the exception is caught.
At this point, all folders have been visited and all folder paths have been generated.
Resources
Original Link: https://dev.to/tomkanabay/recursive-function-to-find-all-folder-paths-in-vcenter-server-inventory-using-powercli-lha

Dev To
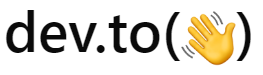
More About this Source Visit Dev To