An Interest In:
Web News this Week
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
- April 19, 2024
- April 18, 2024
Setup a Django project with Docker
We all know that Django is great for applications even if we want to, with Docker the conversation gets better!
How about I show you an initial Setup for your projects that can not only speed up your development, but also make your environment advanced and complete for your applications?
Interested? So read on and leave the rest to me.
Requirements
- Python
- Docker
Starting
We are going to use a test application to create the development environment, but remember that this setup applies to any project.
Just be mindful of the changes you make.
1 - First, we start a venv enviroment
python -m venv venv && source ./venv/bin/activate
2 - Now we install our dependencies ( and don't worry, I'll tell you what each one will do )
pip install django black python-dotenv psycopg2-binary
And don't forget to create the dependency files:
pip freeze > requirements.txt
- Django --> Our main framework
- black --> Python code formatter
- python-dotenv --> Python-dotenv reads key-value pairs from a
.env
file and can set them as environment variables. - psycopg2-binary --> most popular PostgreSQL database adapter for the Python
Tips:
- For MySQL, MariaDB and derivatives types, use
mysqlclient
3 - Let's init our app:
django-admin startproject your_fantastic_name .
4 - Creating the .env
file:
Go to the root of your workspace and create the .env
file with this values:
# AppSECRET_KEY=your_ultra_secret_key# 1 = True or 0 = FalseDEBUG=# localhost 127.0.0.1 0.0.0.0 ...HOSTS=# POSTGRESPOSTGRES_DB=POSTGRES_USER=POSTGRES_PASSWORD=# PGADMINPGADMIN_DEFAULT_EMAIL=PGADMIN_DEFAULT_PASSWORD=
later these values will be useful
5 - Configuring our application:
First let's go to the core of our application, located in the settings.py
file and we will add some imports
By default the Path is already imported, but to use our python-dotenv
package let's add two more imports:
# settings.pyfrom pathlib import Pathfrom dotenv import load_dotenvimport os
And just below we will use os
to configure the project:
# settings.pyload_dotenv()BASE_DIR = Path(__file__).resolve().parent.parentSECRET_KEY = os.getenv("SECRET_KEY")DEBUG = int(os.getenv("DEBUG", default=0))ALLOWED_HOSTS = os.getenv("HOSTS").split(" ")
Tips 2:
- For the people who gonna use Django with html and css, i like to put all the static files into the templates folder
# settings.pyTEMPLATES = [ { "BACKEND": "django.template.backends.django.DjangoTemplates", "DIRS": [os.path.join(BASE_DIR, "templates")], "APP_DIRS": True, "OPTIONS": { "context_processors": [ "django.template.context_processors.debug", "django.template.context_processors.request", "django.contrib.auth.context_processors.auth", "django.contrib.messages.context_processors.messages", ] }, }]
- For who gonna use Rest-Framework, here is my configs for the app:
# settings.pyREST_FRAMEWORK = { "DEFAULT_PAGINATION_CLASS": "rest_framework.pagination.LimitOffsetPagination", "PAGE_SIZE": 5, "DEFAULT_RENDERER_CLASSES": ["rest_framework.renderers.JSONRenderer"], "DEFAULT_PARSER_CLASSES": [ "rest_framework.parsers.JSONParser", "rest_framework.parsers.FormParser", ], "DEFAULT_THROTTLE_CLASSES": [ "rest_framework.throttling.AnonRateThrottle", "rest_framework.throttling.UserRateThrottle", ], "DEFAULT_THROTTLE_RATES": {"anon": "15/min", "user": "20/min"},}
6 - Setting up the Database in Django:
In this context and I will exclusively use postgres, to configure the connection just modify the DATABASE object with the following values:
# settings.pyDATABASES = { "default": { "ENGINE": "django.db.backends.postgresql", "NAME": os.getenv("POSTGRES_DB"), "USER": os.getenv("POSTGRES_USER"), "PASSWORD": os.getenv("POSTGRES_PASSWORD"), "HOST": "db", "PORT": 5432, }}
More Tips:
- This is optional but recomendy, for storage the Static Files and some other configs for language and more:
# settings.pyLANGUAGE_CODE = "pt-BR"TIME_ZONE = "America/Sao_Paulo"USE_I18N = TrueUSE_TZ = TrueSTATIC_URL = "static/"STATICFILES_DIRS = (os.path.join(BASE_DIR, "templates/static"),)STATIC_ROOT = os.path.join("static")MEDIA_URL = "media/"MEDIA_ROOT = os.path.join(BASE_DIR, "media")DEFAULT_AUTO_FIELD = "django.db.models.BigAutoField"
Docker Setup
First we need the build for our app, let's create him!
# Image of python with DebianFROM python:3.9-bullseye# Set WorkdirWORKDIR /usr/src/app# Envs for don't generate pycacheENV PYTHONDONTWRITEBYTECODE 1ENV PYTHONUNBUFFERED 1# Some important libsRUN apt update && apt upgrade -y \ && apt install gcc python3-dev musl-dev bash build-essential libssl-dev libffi-dev -y# Upgrade pip, copy requirements and install all the project dependenciesRUN pip install --upgrade pipCOPY ./requirements.txt .RUN pip install -r requirements.txt# Entrypoint gonna be useful when we up the containerCOPY entrypoint.sh .RUN sed -i 's/\r$//g' /usr/src/app/entrypoint.shRUN chmod +x /usr/src/app/entrypoint.sh# Copy all the files for the root dirCOPY . .ENTRYPOINT ["/usr/src/app/entrypoint.sh"]
Now we need our compose file to up all the tools and our app:
version: "3"services: web: build: . container_name: "web-app-server" command: python manage.py runserver 0.0.0.0:8000 volumes: - .:/usr/src/app/ ports: - 8000:8000 networks: - postgres env_file: - ./.env depends_on: - db db: image: postgres:13.0-alpine container_name: "postgres-db-server" volumes: - postgres_data:/var/lib/postgresql/data/ environment: - POSTGRES_USER=${POSTGRES_USER} - POSTGRES_PASSWORD=${POSTGRES_PASSWORD} - POSTGRES_DB=${POSTGRES_DB} networks: - postgres env_file: - ./.env pgadmin: container_name: "pgadmin-django-server" image: dpage/pgadmin4 environment: - PGADMIN_DEFAULT_EMAIL=${PGADMIN_DEFAULT_EMAIL} - PGADMIN_DEFAULT_PASSWORD=${PGADMIN_DEFAULT_PASSWORD} volumes: - pgadmin:/root/.pgadmin ports: - "5050:80" networks: - postgres env_file: - ./.env depends_on: - dbvolumes: postgres_data: pgadmin:networks: postgres: driver: bridge
If you notice, our compose will be reading our values that we defined in the .env file, like database name, username, password and so on.
If you are not sure which values are defined in the file, run the command docker-compose config
in the terminal, it will display the compose with all the values.
And finally, our entrypoint file
#!/bin/sh# entrypoint.sh# Verify if the database is connectedif [ "$DATABASE" = "postgres" ]then echo "Waiting for postgres..." while ! nc -z $SQL_HOST $SQL_PORT; do sleep 0.1 done echo "PostgreSQL started"fi# Delete all the tablespython manage.py flush --no-input# Do a new migratepython manage.py migrateexec "$@"if [ "$DATABASE" = "postgres" ]then echo "Waiting for postgres..." while ! nc -z $SQL_HOST $SQL_PORT; do sleep 0.1 done echo "PostgreSQL started"fipython manage.py flush --no-inputpython manage.py migrateexec "$@"
Yea, more tips :):
- For security, add the file
.dockerignore
for exclude some folders of the build:
venv
Now let's start our app
All you will need is just to give a little command:
docker-compose up
And that's it, you will have uploaded all 3 containers, the database, pgAdmin and your own application, all in a setup that is easy to customize and implement new tools.
In case you are in doubt, "how can I access my application with docker?", simple, with Docker you can do everything!
First type:
docker ps
Take the id of your container and:
docker exec -it id_of_container bash
And that's it, you can develop your application in a simple way :)
Now we can see the result!
I hope that with this tutorial I have helped you to make your Django applications with a special touch.
See you next time!
Original Link: https://dev.to/drack112/setup-a-django-project-with-docker-128f

Dev To
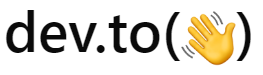
More About this Source Visit Dev To