An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Integrating an ORM in Node.js in 5 easy steps
Hi developers! In this post we are going to implement an ORM (TypeORM) to interact with the Database (MySQL) performing the basic operations CRUD (Create, Read, Update and Delete). Let's start.
Follow me
Get started
In this section we are going to define our model, global configuration, definition of repositories and others.
You can also visit this repository on Github and clone it to your computer. By the way, leave your little star and by the way follow me
Repository: https://github.com/thebugweb/todo-express-api
1. Define model
Create src/entity/Task.ts
file
@Entity
: Convert a class into a TypeORM entity, this entity can be a table or Document, depending on the Database we are using.@Column
: Column decorator is used to mark a specific class property as a table column.@PrimaryGeneratedColumn
: This decorator will automatically generate a primary key.@CreateDateColumn
: This column will store a creation date of the inserted object.@UpdateDateColumn
: This column will store an update date of the updated object.
import { Entity, Column, PrimaryGeneratedColumn, CreateDateColumn, UpdateDateColumn} from "typeorm";@Entity()export class Task { @PrimaryGeneratedColumn() id!: number; @Column() title!: string; @Column() description!: string; @Column("boolean", { default: false }) isCompleted!: boolean; @CreateDateColumn() createdAt!: Date; @UpdateDateColumn() updatedAt!: Date;}
2. Setting TypeORM
Create src/config/database.ts
file. In this file we are going to add the global settings of TypeORM. Properties like:
- Specify the type of database (type, host, username)
- Register our entities.
- Register our migrations.
- Other features.
import { DataSource } from "typeorm";import { Task } from "../entity/Task";export default new DataSource({ type: "mysql", host: "localhost", port: 3306, username: "root", password: "", database: "todo", entities: [Task], synchronize: true, logging: false});
If you want more information visit here
3. Connect database
We import the configuration (src/config/database.ts) and initialize TypeORM to establish connection with the database
import "reflect-metadata";import express from "express";import database from "./config/database";const app = express();database.initialize() .then(() => console.log("Database connected")) .catch(console.error)app.listen(3030, ()=> { console.log("App execute in port:3030");});
4. Define repository
Create src/tasks/task.repository.ts
file. This file will have a class, which is responsible for interacting with TypeORM.
import database from "../config/database";import { DatabaseRepository, Id, Query } from "../declarations";import { Task } from "../entity/Task";export class TaskRepository implements DatabaseRepository<Task> { async create(data: Partial<Task>, query?: Query): Promise<Task> { const repository = database.getRepository(Task); const task = repository.create(data); await repository.save(task); return task; }}
5. Consume the repository
Once we define the repository methods, we can import this class anywhere in our application.
The following example shows an implementation in a controller.
Create src/tasks/task.controller.ts
file
import { Request, Response, NextFunction } from "express"import { DatabaseRepository } from "../declarations";import { Task } from "../entity/Task";export class TaskController { constructor(private repository: DatabaseRepository<Task>) {} async create(req: Request, res: Response, next: NextFunction): Promise<void> { try { const body = req.body; const task = await this.repository.create(body) res.status(200).json(task); } catch (error) { next(error); } }}
Add it on a router and ready!
import { Router } from "express";import { TaskController } from "./task.controller";import { TaskRepository } from "./task.repository";const router = Router();const controller = new TaskController(new TaskRepository());router.post("/tasks", controller.create.bind(controller));export default router;
Conclusions
With these simple steps we have integrated an ORM (TypeORM) to our application. Now is the time for you to let yourself be carried away by your imagination and do more complex things.
In case you have a doubt, you can visit this repository on Github and clone it to your computer. By the way, leave your little star and by the way follow me
Repository: https://github.com/thebugweb/todo-express-api
Follow me
Original Link: https://dev.to/ivanzm123/integrating-an-orm-in-nodejs-in-5-easy-steps-d4a

Dev To
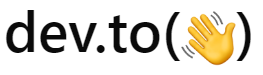
More About this Source Visit Dev To