An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Android login screen using jetpack compose [Part-1]
Almost all android application will have login or registration process in order to authenticate a user. In this article I will be demonstrating how to design android login and registration screen design using jetpack compose. This will be series of several posts where I will try to cover various android components.
Please do share and hit like to this post to encourage me. :)
Configuration used while creating this spinner ,
Compose version : 1.1.0-alpha06
Kotlin version: 1.5.31
Android studio : Android Studio Bumblebee | 2021.1.1 Patch 3
The final output screenshots of this tutorial will be like below image
Step 1: Create an Android Studio project by selecting an Empty compose activity.
Step 2: Add below dependency in app level gradle file as it is required for navigation
implementation "androidx.navigation:navigation-compose:2.4.0-alpha04"
Step 3: Create new file Routes.kt to store routes information
sealed class Routes(val route: String) { object Login : Routes("Login")}
Step 4: Create new package screen
Step 5: Create new File inside screen package screen/ScreenMain.kt and paste below code in it.
@Composablefun ScreenMain(){ val navController = rememberNavController() NavHost(navController = navController, startDestination = Routes.Login.route) { composable(Routes.Login.route) { LoginPage(navController = navController) } }}
Step 6: Create new file screen/Login.kt and paste below code in it
@Composablefun LoginPage(navController: NavHostController) { Box(modifier = Modifier.fillMaxSize()) { ClickableText( text = AnnotatedString("Sign up here"), modifier = Modifier .align(Alignment.BottomCenter) .padding(20.dp), onClick = { }, style = TextStyle( fontSize = 14.sp, fontFamily = FontFamily.Default, textDecoration = TextDecoration.Underline, color = Purple700 ) ) } Column( modifier = Modifier.padding(20.dp), verticalArrangement = Arrangement.Center, horizontalAlignment = Alignment.CenterHorizontally ) { val username = remember { mutableStateOf(TextFieldValue()) } val password = remember { mutableStateOf(TextFieldValue()) } Text(text = "Login", style = TextStyle(fontSize = 40.sp, fontFamily = FontFamily.Cursive)) Spacer(modifier = Modifier.height(20.dp)) TextField( label = { Text(text = "Username") }, value = username.value, onValueChange = { username.value = it }) Spacer(modifier = Modifier.height(20.dp)) TextField( label = { Text(text = "Password") }, value = password.value, visualTransformation = PasswordVisualTransformation(), keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Password), onValueChange = { password.value = it }) Spacer(modifier = Modifier.height(20.dp)) Box(modifier = Modifier.padding(40.dp, 0.dp, 40.dp, 0.dp)) { Button( onClick = { }, shape = RoundedCornerShape(50.dp), modifier = Modifier .fillMaxWidth() .height(50.dp) ) { Text(text = "Login") } } Spacer(modifier = Modifier.height(20.dp)) ClickableText( text = AnnotatedString("Forgot password?"), onClick = { }, style = TextStyle( fontSize = 14.sp, fontFamily = FontFamily.Default ) ) }}
Step 7: Inside your MainActivity.kt add below code snippet
class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { JetpackComposeDemoTheme { Surface( modifier = Modifier.fillMaxSize(), color = MaterialTheme.colors.background ) { ScreenMain() } } } }}@Preview(showBackground = true)@Composablefun DefaultPreview() { JetpackComposeDemoTheme { ScreenMain() }}
That's it, you are done with Login UI using jetpack compose.
In next part [Part-2] we will add some more screens and navigation to those screen.
Find complete code on GitHub, Dont forget to follow
Please follow to get updated posts and hit like to motivate me
Thanks
If this post was helpful, please click the clap button below a few times to show your support!
Original Link: https://dev.to/manojbhadane/android-login-screen-using-jetpack-compose-part-1-50pl

Dev To
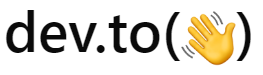
More About this Source Visit Dev To