An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Rest parameters of JavaScript
Have you ever dreamed of creating your own JavaScript function that can accept any number arguments? Today we will learn how to do that with modern JavaScript syntax. And as you might already have guessed: the name of that syntax is rest parameter.
Rest parameter
If we put 3 dots(...
) before the last parameter of a function, that last parameter is called rest parameter.
If we do so, we can access any number of arguments given to that function from the position of the rest paramter to the right, wrapped in an array. For example:
function foo(a, b, ...c) { console.log('a:', a); console.log('b:', b); console.log('c:', c);}foo(1, 2, 3, 4, 5, 6);
It will print the following:
a: 1b: 2c: [3, 4, 5, 6]
Note: The array that rest parameter gives us is a standard JavaScript array, which means we can use any available array method on it.
That's all there is to know about rest parameter.
Info: The ...
in JavaScript can have different meanings based on where it is used. Other JavaScript syntaxes that uses ...
are spread syntax and destructuring assignment.
When there was no rest parameter
From ancient times JavaScript gives all regular functions(non-arrow functions) an local variable named arguments
pointing to an array-like object that holds all the arguments we give to it. Developers faces few problems in that approach however. For example:
- Since it's not an array, array methods don't work on it.
- We need extra boilerplate code to convert
arguments
object to an actual array.
In ES6, rest parameter is introduced to overcome these limitations.
Exercises
Do the exercises below to sharpen your understanding:
Feel free to add your answers in the comment section. You can check my answers here.
When no arguments given
What will be the output of the following code:
function foo(...args) { console.log(args);};foo();
Adder
Write a function named adder
that takes a number as the first argument. After the first argument it takes any number of number arguments. What adder
does is:
- It adds the number of 1st argument to all the other numbers it gets and returns all of those resulted numbers wrapped in an array.
Original Link: https://dev.to/ashutoshbw314/rest-parameters-of-javascript-3jk3

Dev To
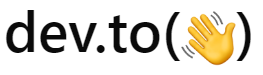
More About this Source Visit Dev To