An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
JAVASCRIPT : Hard to understand Concepts
We web developers either hate or love JAVASCRIPT.
I am here to make to fall in love with it again.
GOAL: To make you understand few concepts in an easy way.
TOPICS COVERED -
- HOISTING
- SCOPE-CHAIN
- CLOSURE
- EVENT LOOP
SO LET'S START:
HOISTING
AS PER MDN DOCS-
JavaScript Hoisting refers to the process whereby the interpreter appears to move the declaration of functions, variables or classes to the top of their scope, prior to execution of the code.
In Simple words -
you just need to remember two GOLDEN rules here-
- Variable declarations are scanned and made undefined.
- Function declarations are scanned and are made available.
Elaboration -
Every time a JS code is executed a Global execution context is created.
This Global execution context scans our code and assigns variables(i.e var) as undefined and when it comes across a function it stores the whole function definition before even the function is executed. And for each function a separate execution context is created.
var hey = "Hey!"; function sayHey(){console.log("Hey!")}console.log(hey);console.log(sayHey());
Here, the output will be as we imagine it to be.
But what confuses a lot of people is -
console.log(hey);console.log(sayHey());var hey = "Hey!"; function sayHey(){console.log("Hey!")var hello;}
here, the first will after executing of code will say "undefined";
Javascript is executed line by line and surprisingly the function sayHey() works perfectly.
one more point to note here is that as the last line of code is initializing a variable without declaring it.
yes its completely possible in variable initialization but not recommended as good practices.
Conclusion-
Remember the two Golden rules from above and you are good to go.
Before ES6 there was no other way to declare variables other than var.
ES6 brought us let and const.
Now lets talk about let and const.
Every developer says let and const is Blocked scoped.
But few get it.
so lets clear the confusion.
The scope in javascript is defined as -
{//BLOCK_SCOPE}
And now the second important point here is let and const are not stored in global execution context and are stored separately(i.e in Temporal Dead Zone)
console.log(a)let a = 2;
Here, you will get an error "Uncaught ReferenceError: a is not defined"
And trust me its better than "undefined".
Another side note here-
const cannot be initialized without assigned to a value.
SCOPE-CHAIN
To understand this concept lemme tell you a short story and you will never forget the concept.
var a = 1;function x(){var b = 2;console.log(a)function(y){console.log(b);}y();}console.log(a);x();
So now lets begin - considering a ice-cream is being eaten by a kid now as per society norms we don't ask it from a kid rather if a grand father is eating it can be asked by his child or grand child as they will pass it but a grand father or father won't ask it from his child for ice-cream.
So, in scope-chain runs in a similar way you can ask for the value of var/let/const from a bigger child to pass it to you but not from a smaller child.
Here in our case Global Execution Context is a Grand Father and everything precedes in a similar fashion.
CLOSURE
Definition(MDN) - A closure is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives you access to an outer function's scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
function makeAdder(x) { return function(y) { return x + y; };}var add5 = makeAdder(5);var add10 = makeAdder(10);console.log(add5(2)); // 7console.log(add10(2)); // 12
After placing a debugger in line 2 & 3 we see in scope a closure is formed.
Closure in simple terms is the lexical environment of its parent function.
EVENT LOOP
To understand event loop lets take a piece a code.
console.log("START")setTimeout(() => {function cb(){ console.log("when will i execute")}cb();}, 0);console.log("END")
here, if i ask what will be the output majority will say - as the setTimeout() has a delay of 0 ms.
the supposed output would be-
START
when will i execute
END
but that's not the case this is not how the callback works.
as js code is executed line by line.
as the js v8 engine see setTimout() it automatically goes to the next line and will execute it at the end.
now after every executing the whole code now as the setTimeout func is a web API and the func inside it will go to callback queue and the event loop will now pass this func to the execution context only when the execution context gets empty.
If you learned anything even a little bit.
Drop a like and for any queries related to the post do drop a comment i will answer it.
Thank you. :)
Original Link: https://dev.to/rajatgangwani/javascript-important-concepts-54m3

Dev To
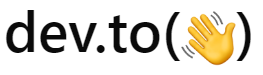
More About this Source Visit Dev To