An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Building a Full Stack NFT Market Place with Near Protocol and React.js
For this guide, I decided to build an NFT Market Place using Near Protocol, although there is a much faster way of setting up a near project using create-near-app, I wanted to put the pieces together to make it easier to understand.
Near Protocol Overview
Near Protocol is a Layer one(L1), developer friendly proof of stake public blockchain. Near Protocol compared to Ethereum has significantly lower Gas fees thanks to a more efficient contract execution model. It also uses Nightshade, a dynamic approach to Sharding.
Benefits of Near Protocol:
- NEAR allows transactions to be processed for an extremely low fee.
- Near Protocol is significantly faster than Ethereum Layer One
- Near features human readable addresses for contracts and accounts
- The use of Rust or AssemblyScript for smart contracts on the Near platform made it easy for developers to write code.
- Developers and users can move assets quickly thanks to the ETH-Near Rainbow Bridge.
Project Overview
This tutorial comprises of three sections, as follows:
- Part 1: Setting up the NFT Contract(Backend and Frontend)
- Part 2: Setting up the Market Place(Backend and Frontend)
We are going to build a Market place for both frontend and backend.
Part 1
The repository for part one of this project is located here.
Prerequisites
Nodejs: is a JavaScript runtime environment built on Chrome V8 engine.
NEAR Wallet Account:NEAR Wallet is a secure wallet and account manager for your accounts on the NEAR blockchain. A Near wallet allows you to interact with applications on Near and securely store tokens and NFTs. For this tutorial we are using a testnet wallet.
Rust Toolchain: Rust Toolchain is a particular version of a collection of programs needed to compile a Rust application it includes, but is not limited to, the compiler rustc
, the dependency manager and build tool, cargo
, the documentation generator, rustdoc
and the static and/ or dynamic libraries.
NEAR-CLI: is a NodeJS command line interface that utilizes near-api-js to connect to and interact with the NEAR blockchain.
Getting Started
Near Protocol uses rust programming language for it's smart contracts. We are going to start with a rust contract template.
From your CLI create a folder/directory named nft-marketplace-part-1
Enter your project root directory:
cd nft-marketplace-part-1
Clone the following rust template into your nft-market-place-part-1
root folder/directory:
git clone https://github.com/near-examples/rust-template.git
Rename the file rust-template
to nft-contract
update your Cargo.toml file:
[package]- name = " rust-template"+ name = "nft-contract"version = "0.1.0"- authors = ["Near Inc <[email protected]>"]+ authors = ["Your name<[email protected]>"]edition = "2021"[lib] - crate-type = ["cdylib"]+ crate-type = ["cdylib", "rlib"][dependencies]near-sdk = "4.0.0-pre.4"+ serde_json = "1.0"[profile.release]codegen-units = 1# Tell `rustc` to optimize for small code size.opt-level = "z"lto = truedebug = falsepanic = "abort"overflow-checks = true
By changing name
, we'll be changing the compiled wasm file's name after running the build script. For Windows it's the build.bat
for OS X and linux build.sh
From this near protocol nft-tutorial in github. Copy the src
directory of nft-contract
folder and copy it into your new own nft-contract
folder .
Your nft-contract
folder/directory should look like this:
nft-contract |___ build.sh |____build.bat |___ Cargo.lock |___ Cargo.toml |___ README.md |___ test.sh |____src |____ approval.rs |____ enumeration.rs |____ events.rs |____ internals.rs |____ lib.rs |____ metadata.rs |____ mint.rs |____ nft_core.rs |____ royalty.rs
approval.rs
: contains functions that controls the access and transfers of non-fungible tokens.
enumeration.rs
: contains the methods to list NFTs tokens and their owners.
lib.rs
: Holds the smart contract initialization functions.
metadata.rs
: Defines the token and metadata structure.
mint.rs
: Contains token minting logic
nft_core.rs
: Core logic that allows you to transfer NFTs between users
royalty.rs
: Contains payout related functions
Logging into near account
We are going to login into near account from CLI.
near login
This is take you to the Near Wallet again where you can confirm the creation of a full-access key. Follow the instructions from the login command to create a key on your hard drive. The key will be located in your operating system's home directory in a folder called near-credentials
.
Build the contract
From nft-contract
directory via CLI
For Windows users, type :
./build.bat
For Mac and Linux users:
./build.sh
Creating a subaccount
if you have followed the prerequisites recommended, you already have a near wallet account created and NEAR CLI installed with full-access key on your machine. The next step is to create a subaccount and deploy the contract to it.
To create subacccount from nft-contract
directory via CLI run:
near create-account nft-contract.youraccountname.testnet --masterAccount youraccountname.testnet
youraccountname
is the name of the testnet wallet that should have been created by you earlier.
After creating the account, you can view the state with the following command:
near state nft-contract.youraccountname.testnet
Your account state should look like this:
Note your codehash, the numbers are all ones(1s), this means that no contract has been deployed to this account, this will change when the contract is deployed.
Deploy the contract
near deploy --accountId nft-contract.youraccountname.testnet --wasmFile res/nft_contract.wasm
Check your account state again:
you can see that your hash changed from only ones, this is a code hash of a deployed smart contract.
Initialize Your contract
To initialize our contract from CLI:
For Mac and Linux Users:
near call nft-contract.youraccountname.testnet new_default_meta '{"owner_id": "nft-contract.youraccountname.testnet"}' --accountId nft-contract.youraccountname.testnet
For Windows Users:
Windows command prompt doesn't accept single quotes so we have to escape them with the backward slash("\"). See below:
near call nft-contract.youraccountname.testnet new_default_meta '{\"owner_id\": \"nft-contract.youraccountname.testnet\"}' --accountId nft-contract.youraccountname.testnet
After initializing, to view metadata via CLI, use command
near view nft-contract.youraccountname.testnet nft_metadata
Minting Token
For Mac and Linux users:
near call nft-contract.youraccountname.testnet nft_mint '{"token_id": "token-1", "metadata": {"title": "My Non Fungible Team Token", "description": "The Team Most Certainly Goes :)", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}, "receiver_id": "youraccountname.testnet"}' --accountId youraccountname.testnet --amount 0.1
For Windows users:
near call nft-contract.youraccountname.testnet nft_mint '{\"token_id": "token-1\", "metadata": {\"title": \"My Cat Fungible Meme Token\", \"description\": \" Grumpy Cat:(\", \"media\": \"https://res.cloudinary.com/dofiasjpi/image/upload/v1649353927/near-tutorial-nfts/OIP.jpg\"}, \"receiver_id\": \"youraccountname.testnet\"}' --accountId youraccountname.testnet --amount 0.1
You can check the collectibles section of your testnet wallet for your freshly minted NFT.
View NFT Information via CLI:
For Mac and Linux users:
near view nft-contract.youraccountname.testnet nft_token '{"token_id": "token-1"}'
For Windows users:
near view nft-contract.youraccountname.testnet nft_token '{\"token_id\": \"token-1\"}'
Transferring NFTs
To transfer an NFT create another testnet wallet account
To transfer run command via CLI:
near call nft-contract.youraccountname.testnet nft_transfer '{"receiver_id": "yoursecondaccountname.testnet, "token_id": "token-1", "memo": "Go Team :)"}' --accountId youraccountname.testnet --depositYocto 1
In this call 1 yoctoNEAR is deposited for security so that the user will be redirected to the NEAR wallet.
Windows users should remember to add backslash before every quote mark like we did previously.
Creating the Frontend
You can follow this nice tutorial to add react with parcel bundler on your nft-marketplace-part-1/src
directory. Create a src
folder/directory inside the nft-marketplace-part-1
and move your index.html
and index.js
inside it.
From the nft-marketplace-part-1
directory via CLI install:
npm install near-api-js regenerator-runtime react-scripts
update your the scripts sections of your package.json
"scripts": { "start": "parcel src/index.html", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" }
Entry point
Update our src/index.js
file with the following code
We are starting with an asynchronous JavaScript function that sets up the required parameters that are passed to the React app.
Let's breakdown the code above, starting with the imports.
We imported from:
config.js
This file contains details of the different networks.
near-api-js
We import all the functions of this dependency to nearAPI
const keyStore = new nearAPI.keyStores.BrowserLocalStorageKeyStore()
Creates a keystore for signing transactions using the user's key which is located in the browser's local storage after the user logs in.
const near = await nearAPI.connect({ keyStore, ...nearConfig });
initializing the connection to the NEAR testnet.
const walletConnection = new nearAPI.WalletConnection(near)
Initializes wallet connection
initContract().then(({ currentUser, nearConfig, walletConnection, near})=> { ReactDOM.render(<App currentUser={currentUser} nearConfig={nearConfig} walletConnection={walletConnection} near={near}/>, document.getElementById('root'));})
The initContract
method is called and data is passed to the App.js
Component
App Component
Let's start be discussing the imports:
Modal
: is a component overlay that will enable us to add a form inside it.useModal
: uses useState
to open and close modal.
Now let's discuss the functions:
signIn
: Remember we passed a walletConnection
object to App.js
Component, once logged in, the object will be tied to the logged-in user and they'll use that key to sign in transactions and interact with the contract.
signOut
: allows user to sign out of wallet account.
sendMeta
: is a function call to the contract method new_default_meta
to set metadata, remember we used this method to set metadata when we used CLI.
displayAllNFT
: is a function call to nft_tokens_for_owner
method in the contract account which retrieves all nfts from the collectibles section of the wallet account.
mintAssetToNFT
: is a function call to mint an assets(picture, video) using the nft_mint
method from the contract.
Create file names config.js
, Modal.js
, useModal.js
Close.js
and App.css
in your nft-marketplace-part-1/src
directory with the following code:
config.js
useModal.js
Modal.js
Close.js
App.css
For this file you can find the CSS code here
To run React app from CLI:
From nft-marketplace-part-1
directory, use command:
npm start
Original Link: https://dev.to/kels_orien/building-a-full-stack-nft-market-place-with-near-protocol-and-reactjs-ak9

Dev To
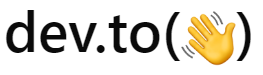
More About this Source Visit Dev To