An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Advent of code day1
Well, the goal is that we to solve 25 programming challenges to get a star and get better at out job.
As expected, it start with easy examples
Part One
In the first challenge (Sonar Sweep), as the submarine descends, a series of numvers appear on the screen that are the input of out puzzle.
We need to find all the numvers that are greater than the previous number.Usually the first numver does not count because the first number does not have previous number.
adventofcode gave an example:
* 199 (N/A - no previous number)+ 200 (increased)+ 208 (increased)+ 210 (increased)- 200 (decreased)+ 207 (increased)+ 240 (increased)+ 269 (increased)- 260 (decreased)+ 263 (increased)
In this example, the answer is 7
, because the are seven numbers that have icreased compared to the previous number.
After reveiving the input file, we go to solve problem using a programming language.Of course, you can also count these manually:)
Here we use Python
Read input data
The first step is for us to read the input of the puzzle and clear its data.
with open("input.txt") as f: lines = f.readlines() numbers = [int(i.strip()) for i in lines]
Iterate on data and calculating it
The next step is iterate on data with for
loop or any method we we can and see if it has increased or not.
# create a variable containing the first number for first itrationprev_num = numbers[-1]# on start result is 0result = 0for i in numbers: # check if the previous value is lower than the current value, increase count by 1 if i > prev_num: result += 1 # update the prev_num for the next iteration prev_num = iprint(f"[ part1: {result} ]")
Part Two
It is now said:
Considering every single measurment isn't as useful as you expected: ther's just too much noise in the data. Instead, consider sums of a three-measurment sliding window.
adventofcode gave an example:
199 A 200 A B 208 A B C 210 B C D200 E C D207 E F D240 E F G 269 F G H260 G H263 H
That means we have to add the numbers three by three and then compare them.
A: 607 (N/A - no previous sum)B: 618 (increased)C: 618 (no change)D: 617 (decreased)E: 647 (increased)F: 716 (increased)G: 769 (increased)H: 792 (increased)
In this example, the answer is 5
.
Now let's go on to writing a programe for this.
# on start result is 0result = 0size = len(numbers)# create a variable containing the sum of the first three-measurement sliding windowprev_window = sum(numbers[:3])# iterate over the size of the depths file for i in range(3, size): # calculate the current three-measurement sliding window curr_window = prev_window + numbers[i] - numbers[i-3] # check if the previous value is lower than the current value, increase count by 1 if prev_window < curr_window: result += 1 # update the prev_window for the next iteration prev_window = curr_windowprint(f"[ part2: {result} ]")
Goodbye :)
Original Link: https://dev.to/yasinazadpour/advent-of-code-day1-4175

Dev To
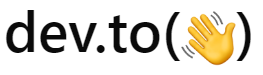
More About this Source Visit Dev To