An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
April 5, 2022 03:25 pm GMT
Original Link: https://dev.to/readymadecode/react-dropdown-component-1n36
React Dropdown Component
Dropdowns are toggleable, contextual overlays for displaying lists of links and more. They are made interactive with the included Bootstrap dropdown. Dropdown are toggled by clicking.
Create React Dropdown Component
class Dropdown extends React.Component { constructor(props) { super(props); this.toggleDropdown = this.toggleDropdown.bind(this); this.handleMouseEvent = this.handleMouseEvent.bind(this); this.handleBlurEvent = this.handleBlurEvent.bind(this); this.hasFocus = this.hasFocus.bind(this); this.state = { show: false }; } componentDidMount() { document.addEventListener('mouseup', this.handleMouseEvent); this.dropdown.addEventListener('focusout', this.handleBlurEvent); } hasFocus(target) { if (!this.dropdown) { return false; } var dropdownHasFocus = false; var nodeIterator = document.createNodeIterator(this.dropdown, NodeFilter.SHOW_ELEMENT, null, false); var node; while(node = nodeIterator.nextNode()) { if (node === target) { dropdownHasFocus = true; break; } } return dropdownHasFocus; } handleBlurEvent(e) { var dropdownHasFocus = this.hasFocus(e.relatedTarget); if (!dropdownHasFocus) { this.setState({ show: false }); } } handleMouseEvent(e) { var dropdownHasFocus = this.hasFocus(e.target); if (!dropdownHasFocus) { this.setState({ show: false }); } } toggleDropdown() { this.setState({ show: !this.state.show }); } render() { return ( <div className={`dropdown ${this.state.show ? 'show' : ''}`} ref={(dropdown) => this.dropdown = dropdown}> <button className="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-toggle="dropdown" aria-haspopup="true" aria-expanded={this.state.show} onClick={this.toggleDropdown}> Dropdown button </button> <div className="dropdown-menu" aria-labelledby="dropdownMenuButton"> <a className="dropdown-item" href="#nogo">Item 1</a> <a className="dropdown-item" href="#nogo">Item 2</a> </div> </div> ); }}
Now we have react <Dropdown /> component and we can use this in our functional or class components easily.
Please like share subscribe and give positive feedback to motivate me to write more for you.
For more tutorials please visit my website.
Thanks:)
Happy Coding:)
Original Link: https://dev.to/readymadecode/react-dropdown-component-1n36
Share this article:
Tweet
View Full Article

Dev To
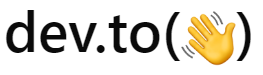
More About this Source Visit Dev To