An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Finding the quickest solution to a problem, and other mistakes
I was going through the help channels of TPH looking for questions I could help with, as usual, when this question popped up:
Looks like they're using their console wrong. And sure enough, that's exactly what happened.
So their problem's solved and I scrolled down, but something just seemed a bit off about their code. I asked them what the code actually does, and they sent me this:
Given a fixed-length integer array
arr
, duplicate each occurrence of zero, shifting the remaining elements to the right.
Pretty simple question, right? Well not exactly. There are multiple ways to achieve this, and the first one that comes to mind is probably not the most ideal one.
Let's look through some ways to solve this:
Add new elements at certain indexes
This is the solution our fellow programmer chose to follow.
It creates a new empty array with the same length as the original, and uses a for loop to add new elements at the array's indexes, but in the case of zero it adds two zeroes and skips an index.
const array = [1, 0, 2, 3, 0, 4, 5, 0];const temp = new Array(arr.length);let tempIndex = 0;for (let i = 0; tempIndex < array.length; i++) { if (arr[i] === 0) { temp[tempIndex] = 0; temp[tempIndex + 1] = 0; tempIndex++; } else { temp[tempIndex] = arr[i]; tempIndex++; }}return temp;
This code works fine, but there's two major flaws:
- Readability: You can't figure out what the code does without paying really close attention to how things are going.
- Maintenance: What if the interviewer asked them to make the code change
1
and not0
? Or both1
and5
? They would have to change 4 lines containing 0, and copy the code to make it work for 5.
Clearly, a temp array + loop would not suffice for this. It's too much work for a simple problem.
We can refactor the code to a better method by removing the tempIndex
portions:
const array = [1, 0, 2, 3, 0, 4, 5, 0];const temp = [];for (let el of array) { if (el === 0) { temp.push(0); temp.push(0); } else { temp.push(el); }}return temp;
We can even use concat
instead of push to reduce one line:
- temp.push(0);- temp.push(0);+ temp.concat([0, 0]);
But now look where we've come, we started off with a simple problem, found an easy at first glance solution that quickly turned out to be too complex, and now we are spending a ton of time trying to improve the solution.
Wouldn't it have been better if the user started off with a good solution?
This brings me to the topic of this post: Problem solving does not mean finding the quickest solution.
Problem solving, what does it mean?
In my opinion, problem solving is comparing multiple solutions with your team and finding the most ideal one before you write a single line of code.
Our fellow programmer thought of the first solution that came to their mind and worked upon it, leading to the issues they got. If they had spent a long time problem solving, they might just have come up with a proper solution and wouldn't even have needed to ask for help.
An example of problem solving
Imagine a scenario where your team has been given a problem and you have to find a solution. There is no time limit, all the boss is looking for is a solution that is:
- Readable.
- Easily maintainable and can be updated to do new stuff without breaking anything.
- Written in the fewest lines of code possible.
You and your team sit down at a coffee table, and you start brainstorming. One guy suggests a probable solution, so the team tries to find issues with the solution that go against what the boss asked for. You find issues, and go on to the next solution that could work.
After some time of comparing solutions, you finally come up with one that accords to the boss's requirements.
Finally, you turn the solution to code and get a promotion .
But this isn't what happens in real life
It would seem like a bigger waste of time to find the best solution when there already exists one, even if it's not a good one. It gets the job done quicker, which gives the false impression that the solution is best.
When it initially released, React's virtual DOM was a game changer in the industry. But since then, React has been trying to squeeze just a little bit more performance out of vdom even though it would be better to start from scratch.
And when Svelte came out, it was the fastest framework ever because it did not use a virtual dom at all.
Let's apply this principle of problem solving to our array problem:
What are common ways of mutating an array's elements? map
and reduce
.
But map can only replace elements at indexes, it cannot insert new ones. Reduce seems like a good solution, but let's find some other solutions before we try it out.
"But wait, you can also flat
an array..."
The ideal solution
This one line solution I came up with was the simplest, shortest possible one:
array.map(num => num === 0 ? [0, 0] : num).flat();
It replaces 0
with an array with two zeroes, then flat
s the array so the nested arrays become actual zeroes.
There is still a way to improve upon this, suggested by another user, which was to use flatMap
instead of map so we can get rid of the extra flat at the end:
array.flatMap(num => num === 0 ? [0, 0] : num);
Conclusion
A simple problem can turn into an overly complex one if you solve it wrongly. Spend more time problem solving than writing code, and while it could increase the amount of time needed to do anything, it will help you and your team in the long run.
And with that, I end today's post. I plan on writing more articles like this in the future, so be sure to follow me on DEV. If you've got anything to say about this post, comment down below because it boosts my post in the forem algorithm.
Adios
Original Link: https://dev.to/code913/finding-the-quickest-solution-to-a-problem-and-other-mistakes-3i87

Dev To
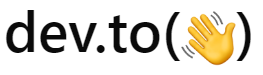
More About this Source Visit Dev To